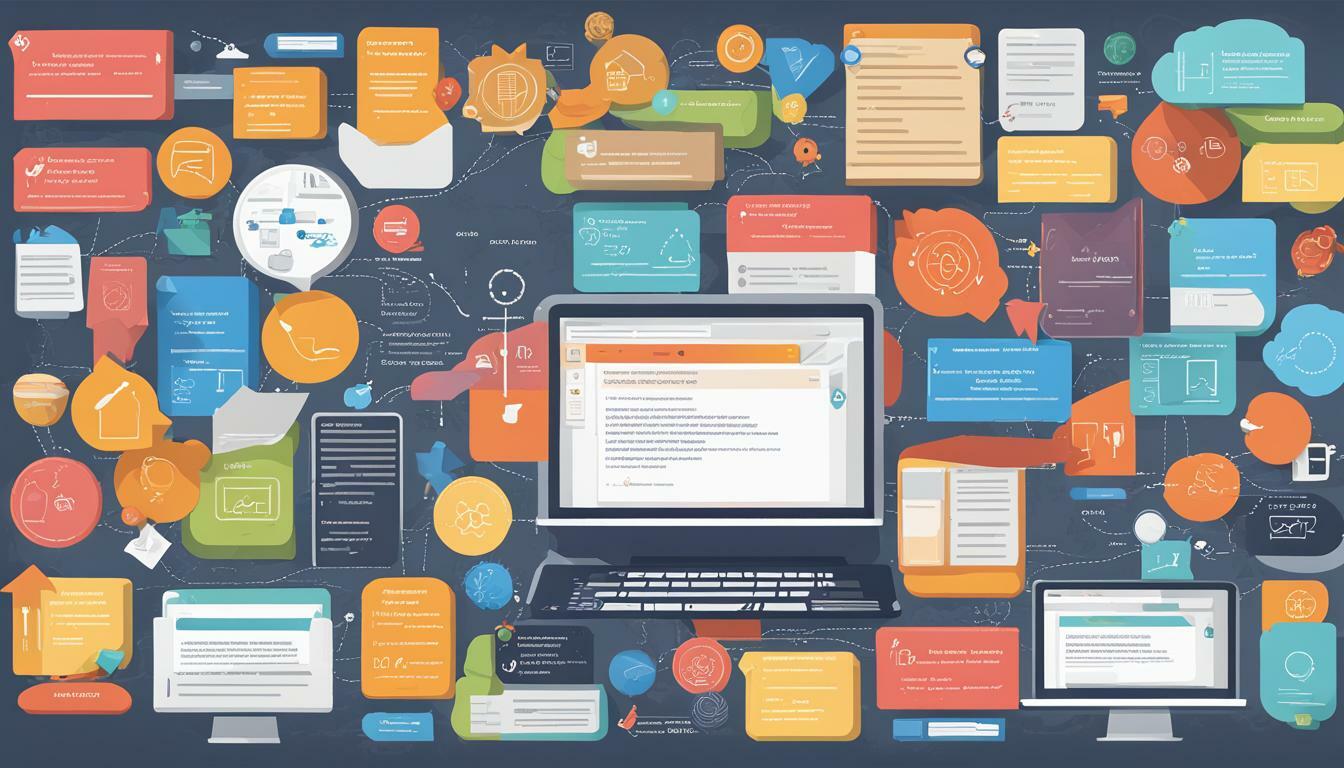
If you’re a software developer or architect, you’re probably familiar with the challenges of building complex systems. One of the biggest challenges is ensuring that your architecture can handle queries and commands effectively without sacrificing performance or scalability. This is where CQRS comes in.
CQRS (Command Query Responsibility Segregation) is a powerful architectural pattern that separates read and write operations into two distinct models. By dividing responsibilities, CQRS allows you to optimize each model independently, resulting in a more scalable and flexible architecture overall.
In this guide, we’ll take a closer look at CQRS, covering everything from its core principles to its implementation and best practices. We’ll also explore the benefits of using CQRS and provide real-world examples of successful implementations. Whether you’re new to CQRS or looking to enhance your skills, this guide has got you covered.
Key Takeaways:
- CQRS (Command Query Responsibility Segregation) is a powerful architectural pattern that separates read and write operations into two distinct models
- CQRS allows you to optimize each model independently, resulting in a more scalable and flexible architecture overall
- In this guide, we’ll cover everything from the core principles of CQRS to its implementation and best practices
- We’ll explore the benefits of using CQRS and provide real-world examples of successful implementations
Understanding CQRS: A Brief Overview
If you’re a software architect or developer, you’ve probably heard of CQRS – Command Query Responsibility Segregation. This pattern has gained popularity in recent years as a way of building scalable, high-performance applications. But what is it, exactly?
At its core, CQRS is an architectural pattern that separates commands and queries into distinct paths. This means that commands – requests that modify data – are handled separately from queries – requests that fetch data. By segregating these responsibilities, CQRS can help to streamline code, improve performance, and simplify testing.
So why is CQRS so important? Well, in traditional architectures, commands and queries are often handled by the same components. This can lead to a number of issues, such as coupling between data retrieval and modification, and contention for shared resources. By separating these responsibilities, CQRS helps to mitigate these issues, allowing for greater flexibility and scalability.
Key Components of CQRS Architecture
The CQRS pattern is implemented through a set of key components that work together to achieve its objectives. Understanding these components is crucial to designing and implementing a successful CQRS architecture.
Command Handlers
Command handlers are responsible for receiving and processing commands from the application layer. A command contains information about an intent to change the system’s state, such as creating an order or updating a record. Command handlers validate the commands and execute the corresponding business logic.
Query Handlers
Query handlers are responsible for receiving and processing queries from the application layer. Unlike commands, queries do not modify the system’s state but retrieve information from it. Query handlers execute the corresponding query logic and return the requested data to the application layer.
Event Sourcing
Event sourcing is a technique used to store an application’s state as a sequence of events that represent changes to that state. Events are immutable and are stored in an event store, which acts as the source of truth for the application’s state. Event sourcing enables the application to derive any current state from the event stream’s history, making it easier to audit and understand the system’s behavior.
Event Stores
An event store is a database that stores an application’s events in a sequence. The event store can be queried to retrieve a specific event or stream of events. Event stores can be either SQL or NoSQL databases, but they are usually optimized for sequential writes and reads.
Message Buses
A message bus is a communication channel that transfers messages between components in a system using a publish-subscribe or point-to-point communication model. In CQRS, message buses are used to communicate between the application layer, command handlers, query handlers, and event handlers. Message buses enable loose coupling between components and allow for easy scalability and fault tolerance.
Implementing CQRS: Step-by-Step Guide
Implementing CQRS in a software project can be broken down into several key steps that cover both design and development. Here’s a step-by-step guide to implementing CQRS:
Step 1: Design the Command and Query Models
The first step in implementing CQRS is to design the command and query models. Commands represent the write operations in the system and queries represent the read operations. It’s important to define these models clearly and ensure they are decoupled from each other.
Tip: Use domain-driven design (DDD) principles to define your models. This can help ensure that your models accurately represent the business processes and operations in your system.
Step 2: Handle Data Persistence
CQRS requires data to be stored separately for reads and writes. This means that you’ll need to handle data persistence differently for each model. For writes, you can use a traditional relational database, while for reads, you could use a NoSQL database or a read-only replica of the relational database.
Tip: Consider using event sourcing to handle data persistence for writes. This can provide a more flexible and scalable way of storing data.
Step 3: Manage Event Streams
Event sourcing is a key part of CQRS. It involves storing events that represent changes to the state of the system. To use event sourcing, you’ll need to manage event streams for each aggregate root in the system. This involves appending new events to the stream and retrieving events from the stream to rehydrate the aggregate root.
Tip: Use a message bus to handle event streams. This can provide a scalable way of distributing events across different parts of the system.
Step 4: Integrate with Existing Systems
CQRS can be integrated with existing systems that may already have a different architecture. However, this requires careful planning and consideration of how the different systems will interact with each other.
Tip: Use a well-defined integration pattern to integrate CQRS with other systems. For example, you could use an event-driven architecture to facilitate communication between different systems.
Step 5: Test and Refine
Like any major architectural change, it’s important to thoroughly test your CQRS implementation. You should also be prepared to refine and tweak your implementation as you encounter real-world scenarios.
Tip: Use automated testing to ensure that your implementation is robust and reliable. This can help you catch issues early and avoid costly bugs in production.
Unlocking the Benefits of CQRS
CQRS offers a range of benefits that can significantly impact the effectiveness of software architecture. By utilizing CQRS, developers can:
- Improve scalability: Separating commands from queries can allow for easier horizontal scaling of the system. Commands can be processed independently without interfering with the querying of data.
- Optimize performance: By removing the need for complex joins and queries, performance can be improved. Additionally, event sourcing allows for quick access to historical data, which can be useful for auditing and debugging.
- Simplify the codebase: Separating commands and queries can lead to cleaner code, reducing complexity and making it easier to maintain over time.
- Enhance flexibility: CQRS architecture allows for easier integration with different systems and services, providing greater flexibility and adaptability.
Real-world examples have demonstrated the effectiveness of CQRS in addressing common architectural challenges. For example, CQRS has been successfully implemented in e-commerce systems to speed up order processing and improve scalability. In healthcare systems, CQRS has been used to improve patient data management and enhance the overall user experience.
Overall, mastering CQRS can offer significant benefits to software developers and architects. By utilizing the CQRS pattern, developers can improve scalability, optimize performance, simplify the codebase, and enhance flexibility.
Designing for CQRS: Best Practices
Designing software systems with CQRS requires careful consideration to ensure successful implementation and optimal performance. Here are some best practices to guide your design decisions:
1. Choose the Right Granularity for Commands and Queries
Commands should be designed to accomplish a single task and should be kept simple to avoid unnecessary complexity. On the other hand, queries should be designed to retrieve data efficiently and should be optimized for performance. It’s important to strike the right balance between simplicity and efficiency for both commands and queries to ensure the smooth operation of your system.
2. Ensure Consistency Across Aggregate Roots
When working with multiple aggregate roots, consistency can become a challenge. It’s essential to ensure that changes made to aggregate roots are consistent across the system. To ensure consistency, consider the use of distributed transactions or event-driven communication between aggregate roots.
3. Handle Data Synchronization Carefully
When working with distributed systems, data synchronization can be complex. To avoid inconsistencies, consider using event-driven communication to propagate updates between systems, and ensure that your system can handle data conflicts robustly.
4. Maintain Event-Driven Communication
CQRS relies on event-driven communication between components, and it’s essential to maintain this communication throughout your system. Use reliable queuing systems and message buses to ensure that events are delivered reliably and your system can handle failures gracefully.
5. Stay Aligned with Domain-Driven Design Principles
CQRS fits well with Domain-Driven Design principles, which emphasize the importance of modeling the problem domain accurately. When implementing CQRS, ensure that the model accurately represents the domain and that commands and queries are aligned with the domain model.
By following these best practices, you can ensure that your CQRS implementation is well-designed, robust, and efficient. Keep in mind that these practices are not fixed rules, but rather guidelines to help you make informed design decisions that work best for your specific use case and system requirements.
Real-World Examples of CQRS Implementation
CQRS has been successfully implemented in various industries to address different architectural challenges. Here are some examples:
E-Commerce Platform
An e-commerce platform implemented CQRS to improve performance and scalability. By using separate read and write models, the platform was able to handle high-volume transactions and complex search queries. The write model was responsible for handling orders and inventory management, while the read model provided real-time product information and search results. The platform also utilized event sourcing and message buses to ensure consistency across different components.
Healthcare System
A healthcare system used CQRS to simplify the codebase and improve modularity. The system had to handle a large number of patient records and complex workflows, making it challenging to maintain a cohesive architecture. By using domain-driven design and event-driven communication, the system was able to separate business logic from infrastructure concerns and ensure that different modules could communicate effectively. The CQRS implementation also allowed the system to achieve eventual consistency while minimizing data duplication.
Transportation Management System
A transportation management system used CQRS to enhance flexibility and adaptability. The system had to handle unpredictable changes in logistics and optimize resource allocation in real-time. By using event-driven architectures and message buses, the system was able to react quickly to changing conditions and adjust routes and schedules on the fly. The CQRS implementation also provided a clear separation between different responsibilities, enabling the system to evolve and scale without compromising performance.
These are just a few examples of how CQRS can be used to address different architectural challenges. By leveraging its benefits, developers can create software systems that are more scalable, performant, and adaptable.
Maximizing CQRS with Advanced Techniques
In order to fully harness the power of CQRS, there are several advanced techniques that can be employed to further optimize a system. These techniques provide greater flexibility and scalability, allowing developers to tackle complex challenges with ease.
Event-Driven Architectures
Event-driven architectures are a great way to achieve loose coupling and improve scalability in CQRS systems. By emitting events from one component and consuming them in another, a system can more easily adapt to changes without impacting other parts of the system. This promotes a more modular and flexible architecture, making it easier to scale individual components as needed.
Eventual Consistency
Eventual consistency is a technique that allows for data to be eventually synced across a system, rather than having it immediately consistent at all times. This approach can help improve system performance, as it allows for data to be read from multiple locations without the need for immediate synchronization. By using this technique, systems can ensure that data is eventually consistent across the board while maintaining high scalability.
Event Sourcing
Event sourcing is a way of modeling state changes in a system as a sequence of events. By maintaining an immutable log of events, developers can more easily trace back the state of the system to a specific point in time. This technique is highly scalable, as it allows developers to easily scale write operations by simply appending new events to the log.
Domain-Driven Design
Domain-driven design (DDD) is an approach to designing software systems that focuses on the business domain and the interactions between different components. By modeling the system based on the business domain, developers can create a more natural and intuitive architecture that is better suited to the needs of the business. This approach works particularly well with CQRS, as it allows for a more natural separation of commands and queries and promotes greater code reuse.
By utilizing these advanced techniques, developers can take full advantage of the power of CQRS and build highly scalable and flexible software systems that can adapt to changing business requirements with ease.
Conclusion
CQRS is a powerful pattern that can revolutionize the way software architecture is approached. In this article, we covered the basics of CQRS, its benefits, and how it can be implemented in real-world scenarios. We also discussed best practices for designing CQRS systems, advanced techniques for maximizing its potential, and provided real-world examples of successful implementations.
By mastering CQRS, software architects and developers can achieve improved scalability, performance optimization, simplified codebase, and enhanced flexibility. The separation of commands and queries in CQRS architecture enables developers to focus on specific functionality without affecting other parts of the system. This results in more robust and maintainable software systems.
We encourage readers to start implementing CQRS in their own projects and explore the different strategies and techniques discussed in this article. CQRS is a pattern that can adapt to various scenarios and industries, and can bring significant benefits to any software project.
FAQ
Q: What is CQRS?
A: CQRS stands for Command Query Responsibility Segregation. It is an architectural pattern that separates the read and write operations in a software system. By segregating the command and query responsibilities, CQRS enables better scalability, performance, and flexibility.
Q: How does CQRS differ from traditional architectural approaches?
A: Unlike traditional architectures where both read and write operations are handled together, CQRS separates the two. In CQRS, commands handle write operations, while queries handle read operations. This segregation allows for independent scaling, optimization, and design of each operation type.
Q: What are the key components of CQRS architecture?
A: The key components of CQRS architecture include command handlers, query handlers, event sourcing, event stores, and message buses. Command handlers receive and execute write commands, while query handlers retrieve and return data. Event sourcing records all domain events, and event stores persist these events. Message buses facilitate communication between components.
Q: How can CQRS be implemented in software projects?
A: Implementing CQRS involves designing the command and query models, handling data persistence, managing event streams, and integrating with existing systems. It is a step-by-step process that requires careful planning and consideration of the specific project requirements.
Q: What are the benefits of using CQRS?
A: Some benefits of using CQRS include improved scalability, performance optimization, simplified codebase, and enhanced flexibility. By separating read and write operations, CQRS allows for better control and optimization of each component, resulting in a more efficient and adaptable system.
Q: What are some best practices for designing with CQRS?
A: When designing with CQRS, it is important to consider factors such as command and query granularity, consistency across aggregate roots, data synchronization, and event-driven communication. Following these best practices can help ensure a well-designed and maintainable CQRS implementation.
Q: Can you provide examples of successful CQRS implementations?
A: Certainly! There are numerous real-world examples of successful CQRS implementations across various industries. These examples showcase how CQRS can be adapted to different scenarios, addressing specific challenges and achieving significant benefits in terms of scalability, performance, and flexibility.
Q: Are there any advanced techniques that can be used to maximize the benefits of CQRS?
A: Yes, there are advanced techniques that can enhance the effectiveness of CQRS. These include event-driven architectures, eventual consistency, event sourcing, and domain-driven design. By incorporating these techniques, developers can further optimize their CQRS implementations in complex systems.