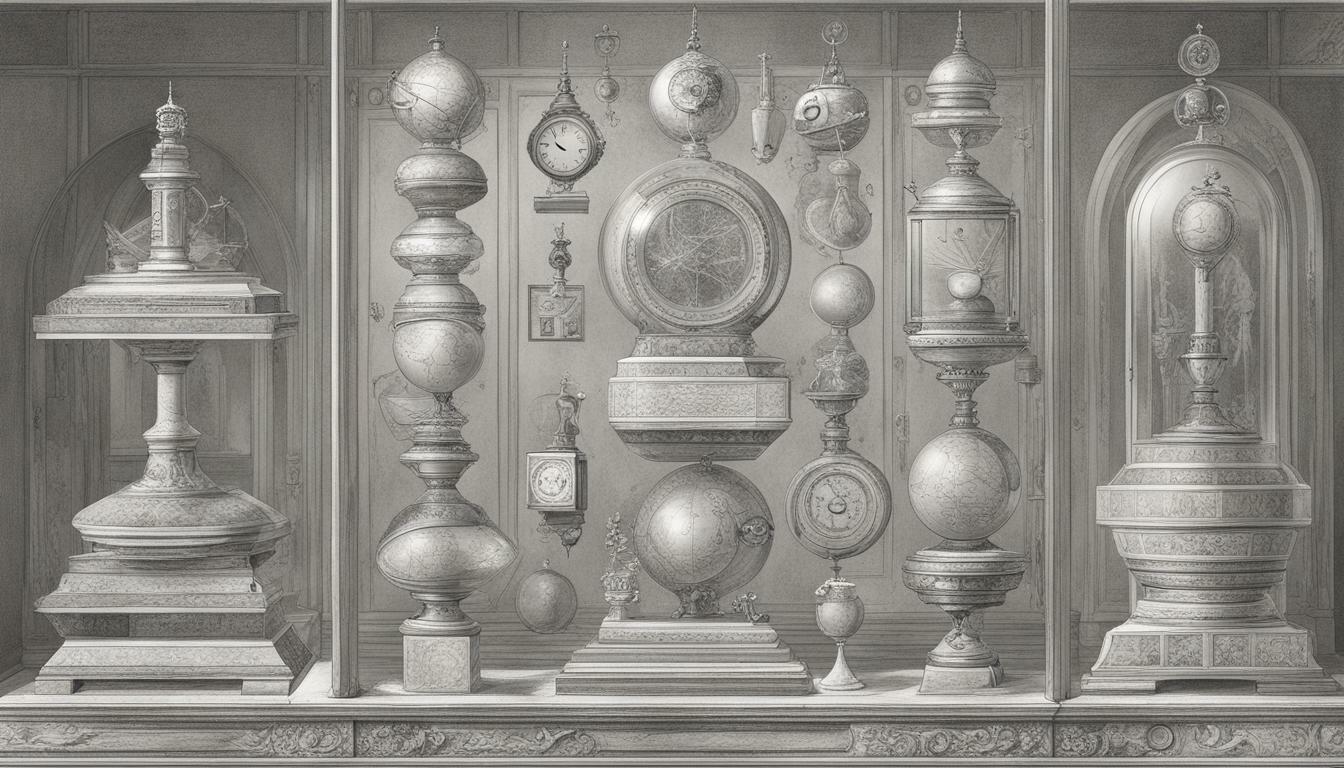
In object-oriented programming, managing object states is crucial for effective software development. The Memento Pattern offers an elegant solution to safeguard and restore object states as needed. By following this design pattern, developers can create flexible and maintainable code that offers a range of benefits.
Through the use of this pattern, object states can be easily saved and restored, even if they were previously unavailable. By safeguarding these states, developers can ensure their projects remain responsive and resilient, even in the face of unexpected errors.
Key Takeaways
- The Memento Pattern is a design pattern used in object-oriented programming.
- The pattern focuses on safeguarding object states for potential restoration.
- Understanding and effectively applying this pattern can result in flexible and maintainable code.
Understanding the Memento Pattern
The Memento Pattern is a powerful design pattern used in object-oriented programming to safeguard object states for potential restoration. It allows developers to save and restore object states efficiently, ensuring seamless code execution in complex software projects.
The Memento Pattern operates on the principle of encapsulation — by separating an object’s state from its behavior, developers can modify the code without affecting the object’s state. This design pattern ensures that the object’s state remains safe and secure, providing a reliable method for restoring it when required.
Understanding the Memento Pattern requires a thorough understanding of object state and its significance in software development. Object states refer to the values of an object’s properties or attributes at a specific point in time. By preserving the object state, developers can restore the object to its previous configuration when required, providing a valuable undo/redo mechanism.
Preserving Object States for Potential Restoration
The Memento Pattern involves three primary actors: the Originator, the Memento, and the Caretaker. The Originator is the object whose state needs to be saved, while the Memento acts as a lightweight object that stores the Originator’s state. The Caretaker objects manage the Memento, storing and retrieving the Originator’s state as required.
The Memento Pattern provides developers with an effective way to separate object state from the behavior, ensuring that the object’s state remains consistent and reliable throughout the application’s lifecycle. By adopting this design pattern, developers can improve code flexibility, simplify state management, and enhance the overall software quality.
In conclusion, the Memento Pattern is an essential tool for developers working on complex software projects. By understanding its principles and adopting best practices for its implementation, developers can create efficient and scalable code that ensures the seamless operation of their software applications.
Implementing the Memento Pattern
After gaining a clear understanding of the Memento Pattern, it’s time to explore its practical implementation in software development. The first step is to identify the object state that needs to be safeguarded. This state could be a single variable or a group of related variables that need to be preserved for potential restoration.
Once the object state is identified, the next step is to create a Memento class that encapsulates the state. This class should have getter methods for retrieving the saved state and setter methods for restoring it. It’s important to ensure that the Memento class’s state is not directly accessible to other classes.
The Originator class is responsible for creating and restoring the object’s state. It should have a method to create a Memento that captures the current state of the object and another method to restore a previously saved Memento. Additionally, the Originator class should have a method to set its state based on the Memento object’s saved state.
The Caretaker class acts as a caretaker for the Memento object, responsible for storing and retrieving it. It should maintain a list of Memento objects, each representing a saved state. When creating a Memento object, the Caretaker class should add it to its list. When restoring an object state, it should retrieve the appropriate Memento object from its list and pass it to the Originator class.
The Memento Pattern’s implementation ensures that object states are safeguarded and can be restored when needed. Following a clear structure ensures easy maintenance and modification of the codebase. Developers can employ this design pattern effectively by creating the necessary classes and following the basic principles of the pattern.
Benefits of the Memento Pattern
The Memento Pattern offers numerous benefits that make it a valuable tool for software development. By safeguarding object states, this design pattern simplifies state management and enhances code flexibility. Let’s take a closer look at some of the key advantages:
- Easy state restoration: The Memento Pattern makes it easy to restore an object’s state to a previous version. By keeping track of object states, developers can retrieve a specific state when needed, simplifying the restoration process.
- Improved flexibility: By separating concerns and delegating responsibility, the Memento Pattern enhances flexibility and modifiability. This makes it easier to adapt to changing requirements and extend functionality without affecting the entire codebase.
- Reduced complexity: With the Memento Pattern, developers can simplify state management by isolating the state-saving and restoring functionality. This reduces the complexity of the codebase and makes it easier to maintain and debug.
Incorporating the Memento Pattern into software development can lead to more efficient and effective code that is easier to maintain and adapt to changing requirements. By safeguarding object states for potential restoration, this design pattern can help developers avoid costly mistakes and ensure the reliability and consistency of their software.
Use Cases of the Memento Pattern
Implementing the Memento Pattern can provide powerful benefits in software development. Let’s examine some real-world use cases where it can be applied effectively:
- Undo/Redo Functionality: Applications that offer undo/redo capabilities require the ability to restore an object to a previous state. The Memento Pattern is ideal for implementing this functionality by saving the states of the object and restoring them as needed.
- Transaction Management: In complex systems that involve multiple transactions, the Memento Pattern can be used to save the state of an object before a transaction and restore it if the transaction fails. This ensures that the system remains in a consistent state.
- Collaborative Editing: When multiple users collaborate on a document, changes are often made simultaneously. The Memento Pattern can be used to save the state of the document before each change and restore it if necessary.
The Memento Pattern proves to be a valuable tool in maintaining object state for potential restoration. By incorporating it into their software development, developers can ensure the integrity and consistency of their applications.
Best Practices for Using the Memento Pattern
Implementing the Memento Pattern can greatly enhance the flexibility and maintainability of your code. To ensure its effective usage, it is essential to follow the best practices outlined below:
- Safeguard only necessary object state: Only object states that require restoration should be safeguarded. This not only reduces memory usage but also makes your code more efficient.
- Ensure state encapsulation: Encapsulate the object’s state to prevent direct access from external sources. This ensures that the state is safeguarded correctly and prevents unwanted modifications to the object’s state.
- Use originators effectively: The originator class should be responsible for creating the memento and restoring the object’s state. This ensures that the memento is created correctly and the object is restored to its previous state without any issues.
- Design for extensibility: The Memento Pattern can be extended to support additional functionalities such as version control. Building extensibility into your implementation can save significant effort in future updates.
By following these best practices, you can ensure that the Memento Pattern is integrated seamlessly into your codebase. However, certain pitfalls should also be avoided during implementation:
- Overuse of the pattern: The Memento Pattern should only be used when necessary. Overusing the pattern can lead to unnecessary complexity and reduced code efficiency.
- Not using the caretaker effectively: The caretaker class should be responsible for storing and managing the mementos. If the caretaker is not implemented correctly, it can lead to memory leaks and issues with object restoration.
- Not updating the memento when necessary: If the object’s state changes frequently, the memento must be updated regularly to ensure it reflects the latest state. Failure to do so can lead to issues during object restoration.
Following these best practices and avoiding common pitfalls can help ensure the effective usage of the Memento Pattern in your codebase, making it more resilient and flexible for future changes.
Comparison with Other Design Patterns
The Memento Pattern is not the only design pattern that deals with saving and restoring object states. Let’s compare it with similar design patterns and see how they differ.
Command Pattern
The Command Pattern is a behavioral design pattern that focuses on encapsulating a request as an object, allowing for it to be parameterized, passed as an argument, and executed at any time. In contrast, the Memento Pattern focuses solely on saving and restoring object states.
Observer Pattern
The Observer Pattern is another behavioral design pattern where an object maintains a list of its dependents and is notified automatically of any state changes. While it also involves object state, it differs from the Memento Pattern in that it does not focus on saving and restoring the state.
Prototype Pattern
The Prototype Pattern is a creational design pattern where objects can create duplicate copies of themselves. While it also involves object state management, it doesn’t focus on saving and restoring the state.
Thus, while the Memento Pattern shares some similarities with other design patterns, it stands apart in its deliberate focus on safeguarding object states for potential restoration.
Conclusion
Overall, the Memento Pattern is a crucial design pattern in object-oriented programming. It allows for the safeguarding of object states for potential restoration, enabling developers to efficiently manage state changes and enhance code flexibility. By following the best practices outlined, developers can effectively apply this pattern in their projects.
Throughout this article, we have explored the core principles of the Memento Pattern and its practical implementation. We have highlighted its numerous benefits and examined real-world use cases where it can be applied effectively. Additionally, we have provided guidelines for using this pattern efficiently and compared it with other relevant design patterns.
In conclusion, a clear understanding of the Memento Pattern is essential for proficient software development. By leveraging this powerful pattern, developers can enhance code flexibility, simplify state management, and safeguard object states for potential restoration. With its practical implementation and numerous benefits, the Memento Pattern can help developers create robust and efficient software systems.
FAQ
Q: What is the Memento Pattern?
A: The Memento Pattern is a design pattern in object-oriented programming that allows for the safeguarding of object states for potential restoration. It is used to preserve the state of an object and provide the ability to restore it to a previous state.
Q: Why is the Memento Pattern important in software development?
A: The Memento Pattern is important in software development as it helps in managing object states and enables potential restoration. It provides flexibility and simplifies state management, making it easier to handle complex operations.
Q: How is the Memento Pattern implemented?
A: The Memento Pattern is implemented by following specific steps. First, the originator object creates a memento object to store its state. The memento object is then stored in a caretaker object. When needed, the originator retrieves the state from the memento to restore its previous state.
Q: What are the benefits of using the Memento Pattern?
A: Using the Memento Pattern offers several benefits in software development. It simplifies state management, enhances code flexibility, and allows for the undo/redo functionality. It also helps in maintaining object state during complex operations.
Q: In what use cases can the Memento Pattern be applied?
A: The Memento Pattern can be applied in various use cases such as implementing undo/redo functionality, saving and restoring game states, and maintaining object state during long-running processes or complex operations.
Q: What are the best practices for using the Memento Pattern?
A: To use the Memento Pattern efficiently, it is recommended to follow these best practices: clearly define the originator and memento objects, ensure proper encapsulation of object states, design a robust caretaker to manage mementos, and avoid modifying the memento directly.
Q: How does the Memento Pattern compare to other design patterns?
A: The Memento Pattern has distinct features and use cases compared to other design patterns. While the Memento Pattern focuses on preserving and restoring object states, other patterns like the Observer Pattern or the Strategy Pattern serve different purposes in software development.