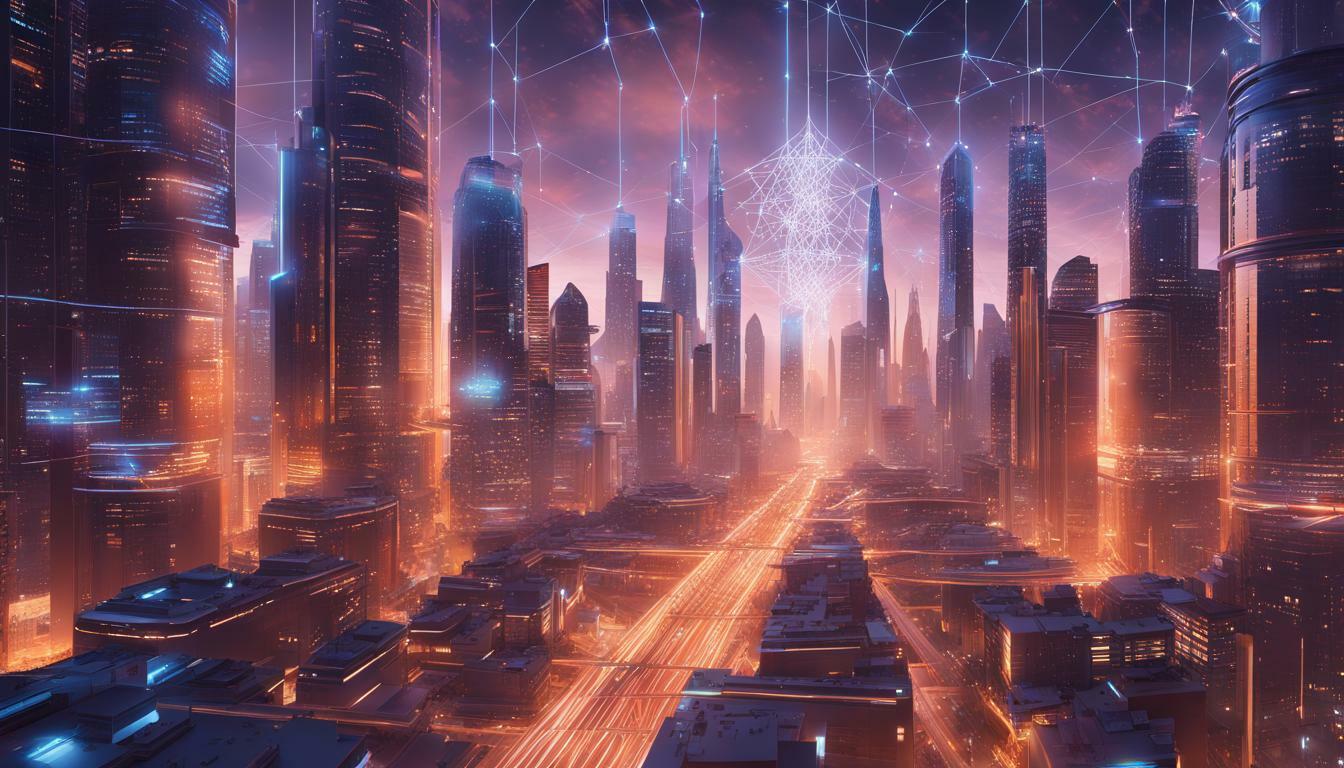
Building APIs with .NET Core is a popular choice among developers due to its flexibility and versatility. However, handling high traffic can be challenging and may require scaling APIs to ensure optimal performance. In this section, we will explore the tools and techniques available for scaling APIs built with .NET Core. We will discuss how to handle high traffic and ensure your .NET Core applications are optimized for performance.
Key Takeaways
- Scaling APIs with .NET Core is essential to ensure optimal performance.
- There are various tools and techniques available for handling high traffic in .NET Core.
- Optimizing the performance of your .NET Core APIs plays a vital role in effective traffic management.
Understanding the Importance of API Scaling
Scaling APIs is an essential aspect of modern software development. As applications gain popularity and usage grows, it becomes necessary to scale APIs to handle the increased traffic. There are many API scaling techniques available, with a variety of tools for scaling APIs, including those geared towards .NET Core applications.
One critical aspect of scaling APIs is the ability to handle high traffic in an efficient and scalable manner. The challenges associated with managing high traffic include the need to ensure high availability and low latency while maintaining adequate throughput and managing resources effectively.
Thankfully, there are many tools available for solving these challenges. By utilizing the right tools and techniques, you can ensure your .NET Core applications are scalable and optimized for performance.
Tools for Scaling APIs
There are many tools available for scaling APIs, including third-party services and custom-built solutions. Third-party services like AWS Elastic Load Balancer, Azure Application Gateway, and Google Cloud Load Balancer provide easy-to-use solutions for load balancing, scaling, and traffic management.
Custom-built solutions offer greater flexibility and control over scaling your APIs and can be tailored to your specific needs. Many .NET Core libraries and frameworks, such as Microsoft.AspNetCore.HttpOverrides and Microsoft.Extensions.Caching.Memory, provide additional functionality for optimizing performance and scaling your applications.
API Scaling Techniques
There are several API scaling techniques that developers can use to ensure their applications can handle high traffic. Horizontal scaling involves adding more instances of your application to handle increased traffic, while vertical scaling involves adding more resources to an existing instance.
Load balancing is another technique used to distribute traffic across multiple instances of your API application. This can be achieved using various load balancing algorithms, including round-robin, least-connections, and IP-hash. Load balancers can also be used to monitor the health of your API instances and automatically remove or add instances as needed.
Throttling is another technique where you limit the number of requests a user can make to your API over a given period to prevent excessive usage. This can help control traffic and maintain application performance during peak loads.
Scaling .NET Core Applications
Scaling .NET Core applications involves several techniques, including caching, asynchronous programming, and optimizing database queries. Caching can help reduce the number of requests to your application and improve response times, while asynchronous programming can help free up resources and improve scalability.
Optimizing database queries can also improve performance and scalability. Tools like Microsoft.EntityFrameworkCore.Proxies and Microsoft.Data.SqlClient can help optimize database queries and improve application performance.
In conclusion, there are many tools and techniques available for scaling APIs built with .NET Core. By utilizing these tools and techniques, developers can build scalable, high-performance applications that can handle high traffic and provide a seamless user experience.
.NET Core Performance Optimization and Traffic Management
As your API application scales, it is crucial to optimize its performance to maintain the quality of service. Consequently, traffic management plays a vital role in ensuring the efficient operation of .NET Core APIs. In this section, we will explore performance optimization techniques and traffic management strategies that will help you maintain the quality of service while handling high traffic.
Performance optimization techniques
One optimization technique that can help increase the performance of your API is caching. By storing frequently accessed data in the cache, you can reduce the load on your application and improve response times.
Asynchronous programming is another powerful performance optimization technique. By running tasks simultaneously, you can reduce the time it takes to complete requests and free up resources for other tasks.
Another important technique is optimizing database queries to ensure that they execute quickly and efficiently. By fine-tuning your database queries, you can reduce the overall response time of your application.
Traffic management strategies
To ensure smooth API operations, it is vital to have traffic management strategies in place. One traffic management technique is to limit the number of requests that your API can handle at a given time. This can be achieved through throttling, where you can set limits on the number of requests a user can make or the number of requests your system will accept.
Another technique that can help manage traffic is queue-based systems. By queueing requests, you can ensure that the API processes them in a timely and efficient manner. This can help prevent your system from becoming overwhelmed with requests during peak traffic periods.
In summary, performance optimization and traffic management are critical components of any .NET Core API application. By implementing the strategies outlined in this section, you can enhance the efficiency and performance of your API applications and ensure that they can handle high traffic volumes.
Load Balancing for .NET Core APIs
When scaling APIs to handle high traffic, load balancing becomes a crucial aspect. Load balancing distributes incoming traffic across multiple API instances, preventing any single instance from becoming overwhelmed with requests. This section will delve into load balancing techniques specifically tailored for .NET Core APIs.
Load Balancing Algorithms: There are multiple load balancing algorithms available, such as round-robin, least connections, and IP hash. The round-robin algorithm directs each new request to the next available API instance, while the least connections algorithm directs requests to the instance with the fewest outstanding connections. IP hash uses the client’s IP address to determine which instance should receive the request.
Using Load Balancers: Load balancers can help manage traffic by distributing incoming requests to different API instances. Load balancers can be hardware-based or software-based, and they offer various features such as SSL termination, session persistence, and Health checks. Examples of popular load balancers for .NET Core applications include Nginx, HAProxy, and Microsoft’s Application Request Routing (ARR) module.
Distributing Traffic: When using load balancing, traffic must be distributed efficiently across all API instances to prevent any one instance from being overloaded. One approach is to use a load balancer to direct API requests to different API instances based on the geographical location of the user. Another approach is to use a service discovery tool to automatically register and deregister API instances as needed.
Load Balancing in Action
Instance | Requests |
---|---|
Instance 1 | 200 |
Instance 2 | 150 |
Instance 3 | 100 |
In the sample table above, we have three API instances handling incoming requests. Using the round-robin load balancing algorithm, the first request would go to instance 1, the second to instance 2, and the third to instance 3, continuing in a cycle. This approach ensures that incoming traffic is evenly distributed across all API instances, preventing any one instance from being overloaded.
Handling Spikes in API Traffic
Sudden surges in API traffic can be challenging to manage, but with the right tools and strategies, you can ensure your .NET Core APIs can handle peak loads efficiently.
Auto-scaling is a technique that allows your API application to automatically adjust its resources to handle increased traffic. By setting thresholds based on usage, your API can spin up additional instances to handle the load.
Throttling is a technique that helps control the amount of traffic to your API by limiting the number of requests allowed per unit of time. This can help prevent the API from becoming overwhelmed and ensure a smooth user experience.
Another strategy is to implement queue-based systems, which help manage traffic spikes by temporarily storing incoming requests in a queue and processing them in a controlled manner rather than immediately responding to each request. This can help prevent the API from becoming overwhelmed and ensure a smooth user experience for all users.
By implementing these techniques, you can ensure your .NET Core APIs can handle sudden spikes in traffic and provide a seamless user experience.
Conclusion
In this comprehensive guide, we have explored the tools and techniques available for scaling APIs built with .NET Core. By adopting the strategies discussed in this article, you can enhance the efficiency and performance of your API applications. Handling high traffic becomes manageable, ensuring a seamless user experience.
Scaling APIs with .NET Core is crucial to meet the demands of modern applications. By implementing performance optimization, load balancing, and handling spikes in API traffic, you can ensure your API application delivers a seamless experience to your users. Utilizing these tools and techniques to manage high traffic can significantly improve the performance of your .NET Core APIs.
FAQ
Q: What is the importance of scaling APIs built with .NET Core?
A: Scaling APIs is essential to handle high traffic and ensure optimal performance of .NET Core applications.
Q: What are the tools and techniques available for scaling .NET Core APIs?
A: There are various tools and techniques, including load balancing, caching, asynchronous programming, and traffic management strategies.
Q: How can performance optimization be achieved in .NET Core APIs?
A: Performance optimization in .NET Core APIs can be achieved through techniques such as caching, asynchronous programming, and optimizing database queries.
Q: How do load balancing techniques work for .NET Core APIs?
A: Load balancing techniques help distribute traffic across multiple instances of your API application, ensuring efficient handling of high traffic.
Q: How can spikes in API traffic be managed?
A: Strategies such as auto-scaling, throttling, and queue-based systems can be implemented to handle unexpected surges in API traffic.