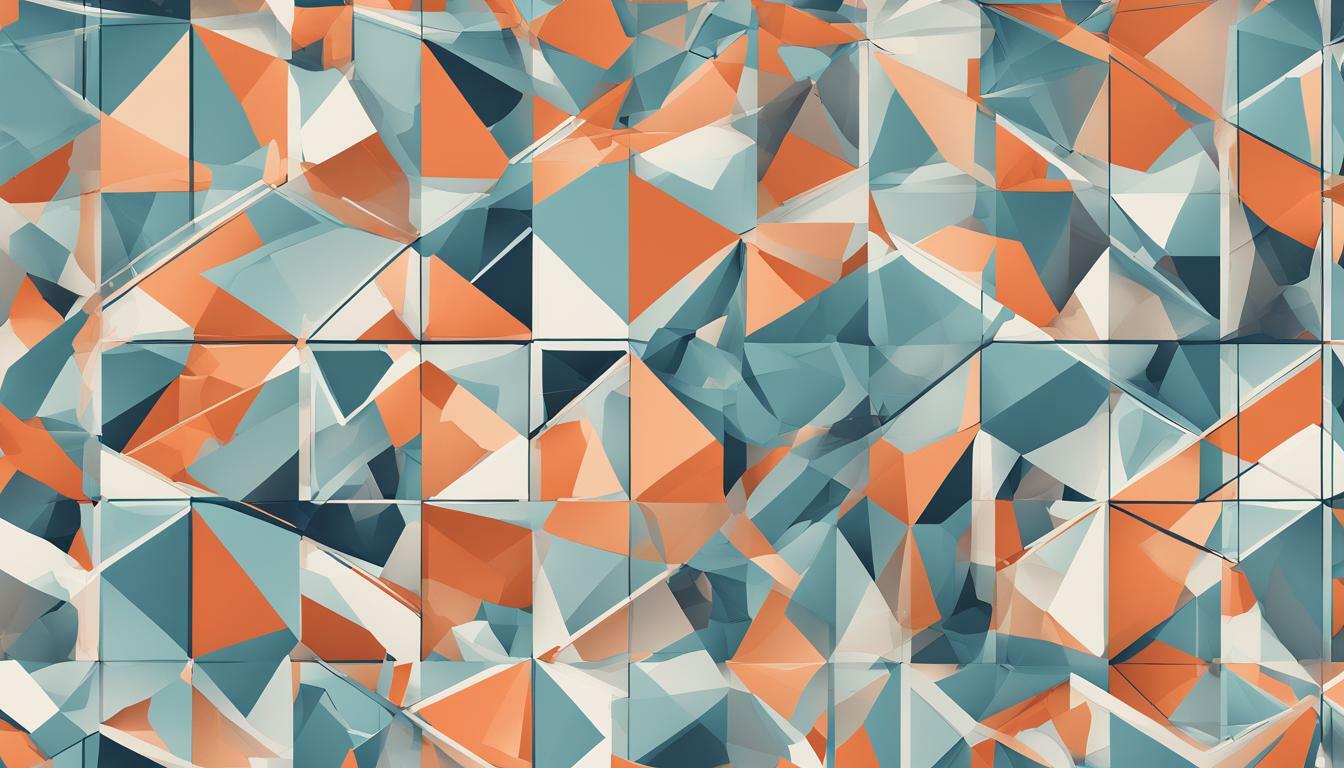
Developing APIs that require consistent and efficient database access can be a complex and time-consuming process. However, with Entity Framework Core, this task becomes seamless and straightforward.
EF Core is an open-source, cross-platform, lightweight, and extensible ORM framework that simplifies the process of accessing and manipulating data in a database. It offers straightforward API access to the database, allowing developers to work with objects rather than writing complex SQL queries
This article will explore how EF Core can enhance API development with seamless database integration, making it easy for developers to work with databases and focus on developing API functionalities without worrying about the underlying data management system.
Key Takeaways
- Entity Framework Core provides seamless database integration with APIs.
- EF Core simplifies the process of accessing and manipulating data through object-relational mapping (ORM).
- EF Core offers improved efficiency and reduced development time with straightforward API access to the database.
Understanding Entity Framework Core
If you’re developing APIs that require seamless integration with a database, Entity Framework Core (EF Core) is a powerful tool that can simplify the process of data access. EF Core is a lightweight, extensible, and cross-platform version of the popular Entity Framework data access technology, designed specifically for .NET Core applications.
To use EF Core effectively, it’s essential to understand the basics of how it works. EF Core relies on object-relational mapping (ORM) to map database tables to CLR objects, allowing you to manipulate data in a database using object-oriented programming techniques. This approach simplifies database access and eliminates the need for tedious manual SQL queries.
There are two main ways to use EF Core: code-first and database-first. In the code-first approach, you define the entities and their relationships in code, and EF Core generates the database schema based on the code. In the database-first approach, you start with an existing database, and EF Core generates the corresponding entity classes based on the schema.
For example, consider a simple scenario where you want to store information about products and their categories in a database. In code-first, you would define two entity classes: Product and Category, and specify their relationships using navigation properties:
public class Product { public int Id { get; set; } public string Name { get; set; } public decimal Price { get; set; } public int CategoryId { get; set; } public Category Category { get; set; } } public class Category { public int Id { get; set; } public string Name { get; set; } public ICollection<Product> Products { get; set; } }
In the database-first approach, you would start with an existing database schema that defines the Products and Categories tables, and use the EF Core tools to generate the corresponding entity classes:
dotnet ef dbcontext scaffold "Server=myServer;Database=myDb;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -o Models
Once you have defined your entities, you can use EF Core to perform a wide range of database operations, such as querying, updating, and transaction management. EF Core provides a powerful query language that allows you to retrieve data from the database using LINQ expressions, and supports a variety of query operators for filtering, sorting, grouping, and joining data.
For example, you could use the following code to retrieve all the products in a specific category:
var products = _context.Products .Where(p => p.CategoryId == categoryId) .ToList();
Overall, EF Core is an essential tool for any API developer who needs to integrate with a database. By leveraging EF Core’s capabilities, you can simplify and optimize your data access code, improve performance, and reduce development time.
Integrating Entity Framework Core with APIs
Integrating Entity Framework Core with APIs is a straightforward process with several steps involved. The first step is to configure EF Core to connect to the database and handle migrations. This can be done by specifying the database provider, connection string, and migration assembly in the application’s startup code.
Once EF Core is properly configured, you can define entities and relationships using either the code-first or database-first approach. In code-first, you define C# classes that map to database tables and use attributes or Fluent API to configure their relationships. In database-first, you generate C# classes from an existing database schema using the EF Core tooling.
After defining entities, you can use EF Core to query, update, and delete data through LINQ expressions. EF Core also supports transaction management, allowing you to group database operations into atomic units of work that can be rolled back in case of failure.
Integrating EF Core with APIs can be done by defining data access logic in API controllers. For example, you can define methods that retrieve, create, update, and delete entities using EF Core’s DbContext class. You can also use EF Core to perform complex queries, joins, and filtering to retrieve specific data from the database.
However, it’s important to balance the benefits of EF Core with the performance and security requirements of APIs. Best practices for integrating EF Core with APIs include minimizing the number of queries executed, optimizing query performance through caching and indexing, and using appropriate security measures to protect sensitive data.
Enhancing API Functionality with Entity Framework Core
Entity Framework Core offers several advanced features for improving the functionality of your APIs. By leveraging EF Core’s capabilities, you can optimize data access and enhance API response times. Here are some techniques for enhancing API functionality using EF Core:
Eager Loading
Eager loading is a technique for loading related data along with the primary data in a single query. This can help reduce the number of database round-trips, thereby improving performance. With EF Core, you can use the Include extension method to specify which related entities to load. For example:
var blogs = _context.Blogs .Include(b => b.Posts) .ToList();
This loads all blogs along with their associated posts in a single query.
Lazy Loading
Lazy loading is a technique for loading related data on-demand, i.e., only when the data is actually accessed. This can help reduce the amount of unnecessary data loaded into memory, improving performance and reducing resource usage. With EF Core, you can enable lazy loading by adding the virtual keyword to navigation properties. For example:
public class Blog { public int BlogId { get; set; } public string Url { get; set; } public virtual ICollection<Post> Posts { get; set; } }
Using lazy loading, accessing the Posts property of a blog entity will automatically load the associated posts from the database.
Caching
Caching is a technique for storing frequently-accessed data in memory for faster access. With EF Core, you can use the MemoryCache class to cache query results. For example:
var blogs = await _cache.GetOrCreateAsync("blogs", async entry => { entry.SlidingExpiration = TimeSpan.FromMinutes(5); return await _context.Blogs.ToListAsync(); });
This caches the results of a query for 5 minutes, reducing the number of database queries made during that time.
Complex Queries
EF Core supports advanced query features such as joins, filtering, and grouping. You can use these features to retrieve specific data from the database and shape it into the desired format for your API responses. For example:
var data = _context.Blogs .Where(b => b.Url.Contains("example.com")) .Join(_context.Posts, b => b.BlogId, p => p.BlogId, (b, p) => new { Blog = b.Url, Post = p.Title }) .ToList();
This retrieves all posts from blogs with URLs containing “example.com” and returns a list of anonymous objects containing the blog URL and post title.
Pagination, Sorting, and Filtering
EF Core makes it easy to implement pagination, sorting, and filtering in your APIs. You can use the Skip and Take methods for pagination, the OrderBy and ThenBy methods for sorting, and the Where method for filtering. For example:
var page = 2; var pageSize = 10; var data = _context.Posts .Where(p => p.PublishedDate < DateTime.Now) .OrderByDescending(p => p.PublishedDate) .Skip((page - 1) * pageSize) .Take(pageSize) .ToList();
This retrieves the second page of posts that have been published and orders them by the publication date in descending order.
By using these advanced features of Entity Framework Core, you can enhance the functionality of your APIs and provide a better user experience for your consumers.
Conclusion
In conclusion, Entity Framework Core is a powerful tool for achieving seamless database integration for APIs. By simplifying the process of accessing and manipulating data through object-relational mapping, EF Core enhances API development, efficiency, and reduces development time.
Understanding the basics of EF Core and its different approaches to using it, including code-first and database-first, is essential for achieving optimal data access to databases. By integrating EF Core with APIs, developers can configure it to connect to the database and handle migrations, generate SQL queries, and perform CRUD operations within API controllers.
Moreover, by exploring EF Core’s advanced features, such as eager loading, lazy loading, and caching techniques, developers can optimize data access and improve API response times. Complex queries, joins, and filtering further enhance data retrieval and provide support for pagination, sorting, and filtering for APIs.
For those interested in mastering EF Core, tutorials, documentation and hands-on practice are recommended to fully leverage its capabilities for creating efficient and robust APIs. Incorporating EF Core into API development can help achieve seamless database integration, and provide an efficient solution for data access and manipulation.
FAQ
Q: What is Entity Framework Core?
A: Entity Framework Core is a technology that simplifies the process of accessing and manipulating data in a database through object-relational mapping (ORM). It provides seamless integration between databases and APIs, making database access and data manipulation more efficient and reducing development time.
Q: How does Entity Framework Core work with .NET Core?
A: Entity Framework Core can be used with .NET Core to provide data access to databases. By defining entities and relationships, developers can query, update, and manage transactions with ease. There are different approaches to using EF Core, such as code-first and database-first.
Q: How do I integrate Entity Framework Core with APIs?
A: To integrate Entity Framework Core with APIs, you need to configure EF Core to connect to the database, handle migrations, and generate SQL queries. Within API controllers, you can perform CRUD operations using EF Core. It is important to follow best practices to maintain API performance and security while leveraging EF Core’s capabilities.
Q: What advanced features does Entity Framework Core offer for API functionality?
A: Entity Framework Core offers advanced features that can enhance API functionality. Techniques like eager loading, lazy loading, and caching can optimize data access and improve API response times. EF Core also supports complex queries, joins, and filtering to retrieve specific data from the database. It can be used to implement pagination, sorting, and filtering in APIs.