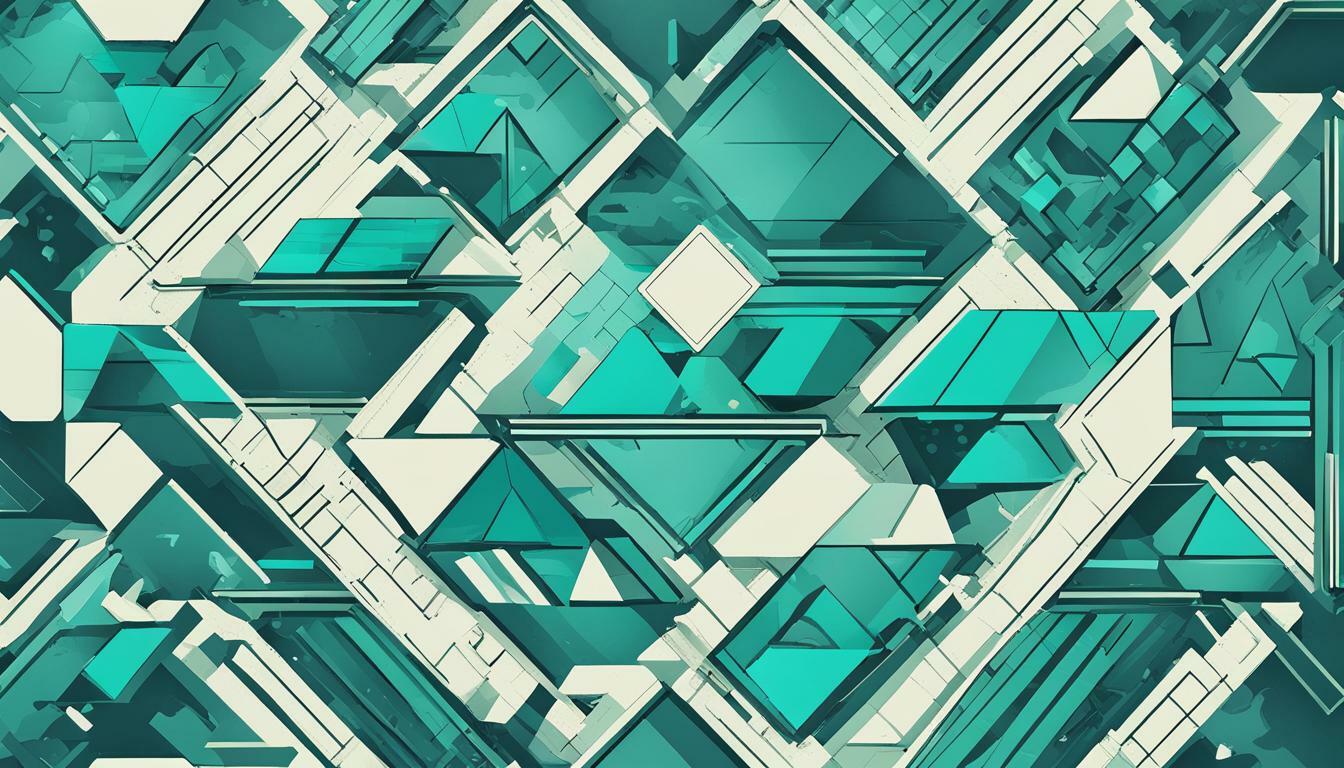
Software development is a dynamic and ever-evolving field. However, in the pursuit of making better software, we often overlook the importance of designing unchangeable elements. Immutable design, a concept derived from functional programming, proposes to create software that prioritizes stability and reliability over flexibility.
Immutable design is all about creating software elements that cannot be modified once they are created. This approach can contribute to better software architecture and engineering by simplifying complex code structures, improving performance, and optimizing maintainability.
Key Takeaways
- Immutable design prioritizes stability and reliability over flexibility in software development.
- Unchangeable software elements can contribute to better software architecture and engineering.
- Immutable design simplifies complex code structures, improves performance, and optimizes maintainability.
Understanding Immutable Design in Software Architecture
Immutable design is a powerful approach to software architecture that prioritizes unchanging software elements. This approach is gaining in popularity due to its ability to deliver significant benefits in terms of scalability, reliability, and performance.
Software architecture is the foundation of any software development project, and it is essential to incorporate immutable design principles into the overall design. By doing so, developers can create a more robust, efficient, and scalable system that can withstand the test of time.
The Fundamental Principles of Immutable Design
Immutable design is based on two key principles: immutability and purity. Immutability refers to the idea that once a data structure is created, it cannot be modified. Instead, any changes to the structure result in the creation of a new structure.
Purity, on the other hand, refers to the idea that functions should have no side effects and should always return the same output for a given input. This means that pure functions are entirely predictable, making them easier to test and debug.
By incorporating these principles into software architecture, developers can create a more stable and scalable system. Immutable data structures ensure that objects retain their integrity, and functional programming techniques eliminate the risk of unexpected side effects.
The Benefits of Immutable Design in Software Architecture
Immutable design offers several benefits in terms of software architecture. By using immutable data structures and pure functions, developers can:
- Create more robust systems that are less prone to bugs and errors.
- Reduce the complexity of the codebase, making it easier to maintain and debug.
- Improve the efficiency of the system by reducing the need for expensive data copying operations.
- Make the system more scalable by reducing the impact of concurrent access and enabling horizontal scaling.
- Enhance the reliability of the system by minimizing the risk of unexpected side effects and improving error handling.
Overall, immutable design is an essential part of modern software architecture and software engineering. By adopting these principles, developers can create more efficient, scalable, and reliable software systems that can meet the demands of today’s complex business environments.
Enhancing Code Maintainability through Immutable Design
Code maintainability is a crucial aspect of software development. It refers to the ease with which a software system can be modified, understood, and debugged by developers. Immutable design can significantly enhance code maintainability, making it easier to manage code over time.
One of the primary advantages of immutable design is that it promotes consistency and predictability in the behavior of software elements. Immutable objects cannot be changed once they are created, which eliminates unexpected side effects due to mutable state changes.
Immutable design also enables better version control, as changes made to an immutable element create a new instance of that element, rather than modifying the original element in place. This makes it easier to track changes and revert to a previous version if necessary.
By reducing the complexity of code, immutable design can make it easier to understand. Developers can focus on the essential aspects of the code without worrying about the effects of changing mutable state. This simplification can make it easier to spot errors and debug code.
There are several techniques that can be used to apply immutable design principles to software development. One common approach is to use immutable data structures, which are not modified after they are created. Another technique is to use immutable variables, which cannot be reassigned once they are initialized.
By adopting immutable design principles, developers can significantly improve code maintainability, making it easier to maintain and modify software systems over time.
Achieving Software Scalability with Immutable Design
Immutable design can greatly contribute to software scalability. The ability to scale a software system effectively relies on the system’s ability to handle concurrent access and distribute workloads across multiple nodes. Immutable design can play a significant role in achieving both of these goals.
Immutable data structures, such as those used in functional programming, provide a way to ensure that data is thread-safe and can be accessed by multiple threads simultaneously without the risk of data corruption. This is because once a data structure is created, it cannot be modified. Instead, any updates to the data structure create a new instance of the structure, leaving the original intact.
By incorporating immutable design principles, software developers can also enable easier horizontal scaling. Immutable data structures can be easily replicated across multiple nodes, allowing the workload to be distributed evenly and ensuring that each node can operate independently from others. This redundancy can reduce downtime and increase system availability.
Additionally, immutable design can help to reduce the overhead associated with locking and synchronization, making it easier and more efficient to scale a system. Without the need for locks, concurrent access to data becomes much simpler. This can result in a significant performance improvement for large-scale systems.
Overall, by using immutable design principles, software developers can create software systems that are more scalable and can handle large amounts of data more efficiently. This can lead to cost savings, increased productivity, and a better end-user experience.
Ensuring Software Reliability through Immutable Design
Software reliability is a critical aspect of software development. Immutable design can significantly contribute to improving software reliability by minimizing the risk of unexpected side effects and improving error handling.
One of the main challenges in software development is dealing with unexpected changes in the state of a program. Immutable design can help address this challenge by ensuring that software elements remain unchangeable throughout the program’s lifecycle. This approach mitigates the need for developers to track changes manually, reducing the likelihood of introduction of errors and bugs.
Immutable design can also help improve error handling by making it easier to isolate and identify errors. Since the elements are unchangeable, developers can focus on identifying the origin of the error, rather than investigating a complex series of changes that may have caused the error.
There are several real-world examples where immutable design has contributed to software reliability. For instance, the popular open-source database management system, PostgreSQL, uses immutable design for its transactional processing, reducing the risk of data corruption and improving reliability.
In summary, immutable design plays a vital role in ensuring software reliability by minimizing the risk of unexpected side effects, improving error handling, and enabling better debugging. Its use in software development can help developers create more reliable software that meets the demands of users.
Optimizing Software Performance with Immutable Design
Immutable design can be a valuable tool for optimizing software performance. By reducing memory usage, eliminating unnecessary state transitions, and enabling more efficient caching, software can run faster and smoother. Here are some techniques and benchmarks to consider:
Minimizing Memory Usage
Immutable design can help reduce memory usage by eliminating unnecessary copies of objects and data structures. When data is immutable, it only needs to be created once, and then can be shared across different parts of the application without the need for duplication. This can result in significant memory savings, especially in larger applications.
Eliminating Unnecessary State Transitions
By using immutable data, you can simplify your code and reduce the number of state transitions that occur. This can make your application more efficient and easier to understand, as there are fewer opportunities for bugs and errors to arise. Additionally, because immutable data cannot be changed, it is less likely to be accidentally modified or corrupted.
Enabling More Efficient Caching
Immutable data is also ideal for caching, as it can be safely shared across multiple threads and processes without the need for synchronization. By using caching to store frequently accessed data, you can reduce the amount of time your application spends waiting for data to be retrieved from disk or memory. This can result in faster response times and a smoother user experience.
By incorporating immutable design principles into your software development processes, you can help ensure that your applications are both performant and maintainable. Whether you’re working on a small project or a large-scale application, immutability can be a key factor in achieving success.
Overcoming Challenges in Implementing Immutable Design
The concept of immutable design can be a paradigm shift for many software developers, and as with any new approach, it can present challenges. However, the benefits of incorporating immutable elements into software architecture are well worth the effort. Let’s explore some common challenges and how to overcome them.
Challenge 1: Changing Mindset
One of the biggest challenges of adopting immutable design is changing the developer’s mindset. As developers, we are accustomed to modifying and updating code to add new features or fix bugs. Immutable design requires a different way of thinking, where instead of changing existing code, developers create new objects and data structures to represent changes.
To overcome this challenge, it is important first to understand the benefits of immutable design and how it can improve software reliability, scalability, and performance. Developers can start by experimenting with immutable data structures and gradually incorporating them into their code.
Challenge 2: Performance Trade-offs
Some developers may perceive immutable design as having a negative impact on software performance due to the overhead of creating new objects and data structures. However, in many cases, the performance benefits of immutable design outweigh the costs.
One solution to improve performance is to use persistent data structures, which allow sharing of unchanged parts between different versions of data structures, thus avoiding unnecessary object creation and memory allocation. Additionally, modern languages and frameworks provide libraries and language features that enable efficient implementation of immutable data structures.
Challenge 3: Integration with Legacy Code
Another challenge is integrating immutable design with existing legacy code, which may not be designed to support immutable data structures. In some cases, it may be necessary to refactor legacy code to make it more compatible with immutable design principles.
One solution is to gradually incorporate immutable design principles into new code and gradually refactor legacy code to take advantage of immutable design. Additionally, developers can use wrapper classes or adapters to convert legacy code to use immutable data structures.
With patience and persistence, developers can overcome these challenges and enjoy the benefits of immutable design.
Conclusion
Incorporating immutable design principles into software development can have numerous benefits, including enhanced stability, efficiency, maintainability, scalability, reliability, and performance. By ensuring that certain software elements remain unchangeable, developers can reduce the risk of unexpected side effects, improve error handling, and optimize memory usage, among other advantages.
While implementing immutable design may present some challenges and require a shift in mindset, the long-term benefits are well worth the effort. By embracing immutable design, developers can build more robust and reliable software systems that are easier to maintain and enhance over time.
So, if you are a software developer looking to improve the quality and performance of your code, consider incorporating immutable design principles into your next project. With careful planning and implementation, you can achieve the advantages of unchangeable software elements and take your software development skills to the next level.
FAQ
Q: What is immutable design?
A: Immutable design refers to the practice of creating software elements that cannot be changed once they are created. This means that once an element is instantiated, its state cannot be modified. Immutable design promotes stability and efficiency in software development.
Q: Why is immutable design important in software development?
A: Immutable design is important in software development because it helps create more stable and efficient software systems. By ensuring that certain elements cannot be changed, developers can avoid unexpected side effects and simplify debugging processes.
Q: How does immutable design contribute to code maintainability?
A: Immutable design improves code maintainability by making it easier for developers to understand, modify, and debug code. Immutable elements reduce the complexity of code and provide a clear separation between data and behavior.
Q: How can immutable design enhance software scalability?
A: Immutable design can contribute to software scalability by reducing the impact of concurrent access and facilitating horizontal scaling. Immutable elements eliminate the need for locking and help ensure consistency in distributed systems.
Q: How does immutable design enhance software reliability?
A: Immutable design enhances software reliability by minimizing the risk of unexpected side effects and improving error handling. Immutable elements eliminate the possibility of mutable state causing issues and help ensure predictable behavior.
Q: In what ways can immutable design optimize software performance?
A: Immutable design can optimize software performance by reducing memory usage, eliminating unnecessary state transitions, and enabling more efficient caching. Immutable elements allow for better memory management and reduce the need for costly operations.
Q: What are the common challenges in implementing immutable design?
A: Common challenges in implementing immutable design include the need for careful consideration of data updates, potential performance trade-offs, and overcoming conventional programming habits. However, with the right strategies and best practices, these challenges can be overcome.