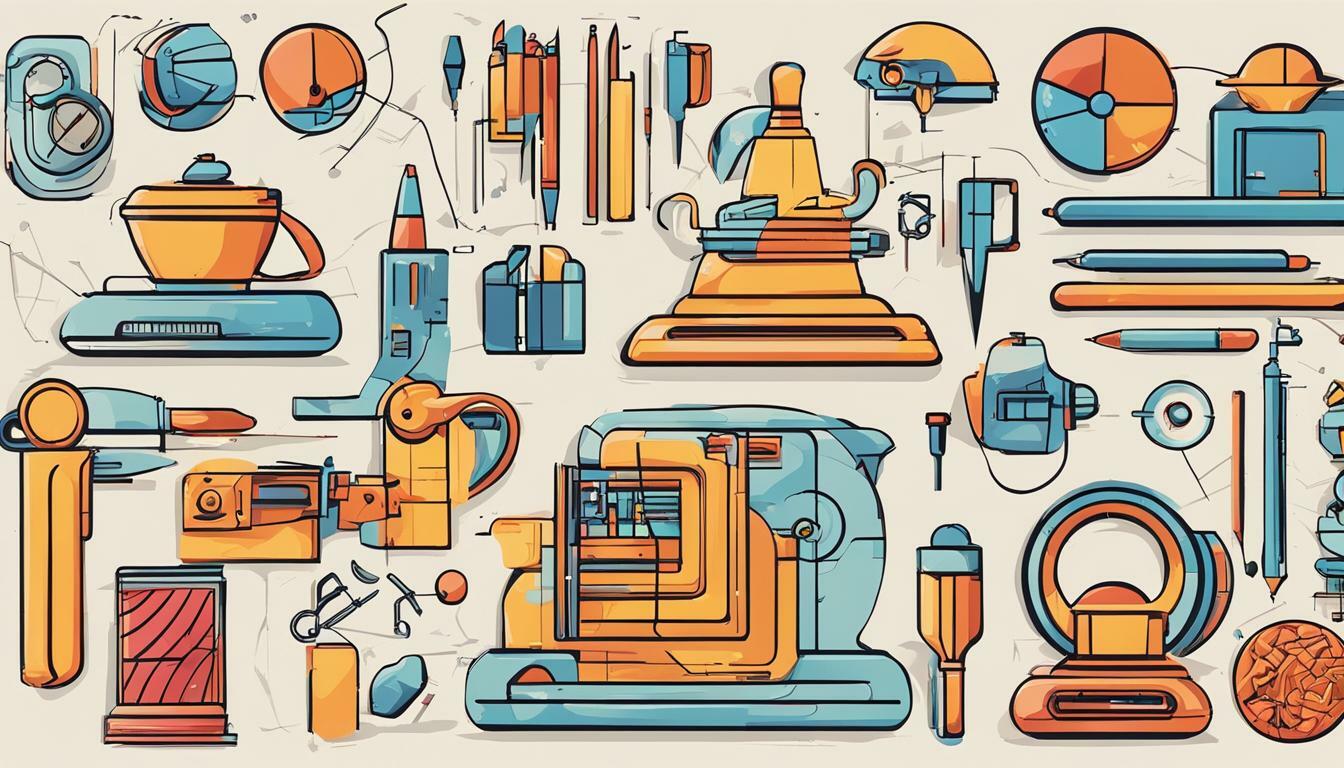
Object-oriented programming (OOP) enables software developers to create complex systems by building objects from other objects. Composite patterns are a powerful tool for achieving this goal. In this article, we will explore the concept of composite patterns and their role in building scalable and reusable software systems.
Key Takeaways
- Composite patterns enable developers to build complex objects from other objects.
- Object composition, aggregation, and inheritance are key concepts of composite patterns.
- The composite design pattern provides a flexible and scalable approach to building composite objects.
- Mastering composite patterns is essential for effective software development.
Understanding Composite Patterns
Composite patterns involve building complex objects by combining simpler ones. There are three ways to achieve this: object composition, object aggregation, and object inheritance. Object composition involves creating objects that contain other objects as part of their internal structure. Object aggregation creates objects that reference other objects, and object inheritance involves creating objects that inherit properties and behaviors from other objects.
Object composition is the most common approach to creating composite objects. It involves creating a container object that contains one or more instances of other objects. These objects can be of different types and can have different behaviors, but they work together to achieve a common goal.
Object aggregation is similar to object composition, but instead of containing other objects, a container object only holds references to them. This approach is useful when the objects being aggregated are too complex to be created directly by the container object.
Object inheritance involves creating a new object that inherits properties and behaviors from an existing object. The new object can then add its own properties and behaviors to create a composite object. This approach can be useful when you want to create a new object that has most of the functionality of an existing object, but with some additional features.
Understanding the different ways to achieve composite patterns is crucial in object-oriented programming. By mastering these patterns, you can create more flexible and scalable objects that can be easily modified and maintained.
The Composite Design Pattern Explained
The composite design pattern is a fundamental concept in object-oriented programming (OOP) and design patterns. Its purpose is to allow developers to create hierarchical structures of objects, where each object can contain other objects and behave as a single entity.
The composite design pattern is commonly used in software development to simplify the creation of complex objects that are made up of smaller, simpler objects. It is a structural pattern that focuses on object composition, rather than object inheritance.
Object-Oriented Programming
Object-oriented programming is a programming paradigm that relies on the concept of objects and their interactions to build applications. Objects are instances of classes that encapsulate data and behaviors. They can communicate with each other by sending messages, which trigger methods that perform specific actions.
The composite design pattern is based on the principles of OOP, as it allows developers to create objects that are composed of other objects, forming a tree-like structure of related entities.
Design Patterns
Design patterns are reusable solutions to common software design problems. They provide a standard way of solving a particular problem, making it easier for developers to understand, communicate, and implement design ideas.
The composite design pattern is one of the 23 original patterns described in the book “Design Patterns: Elements of Reusable Object-Oriented Software”, published in 1994 by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides.
Software Development
The composite design pattern is widely used in software development, particularly in user interfaces, where it allows developers to create complex graphical elements that are made up of simpler ones. It is also used in database systems, where it helps to organize and manipulate hierarchical structures of data.
The composite design pattern is a powerful tool for building scalable and flexible software systems. Its hierarchical structure allows developers to add or remove components easily, without affecting the behavior of the overall system.
Building Composite Objects
Now that we have discussed object composition and aggregation, let’s dive into the process of building composite objects using the composite design pattern. There are several strategies for achieving this, each with its own advantages and trade-offs.
Strategy 1: Recursive Composition
In recursive composition, objects are combined into tree-like structures, where each node can be treated as a composite or a leaf object. This approach allows for flexible and scalable object construction, as composite objects can be built up from simpler ones.
Advantages | Considerations |
---|---|
-Easy to extend and modify the structure of composite objects -Allows for treating composite and leaf objects uniformly | -Can be memory-intensive if structure is too large -Can be complex to implement and maintain |
Strategy 2: Shared Composition
In shared composition, composite objects are built by sharing components between them. This approach can be useful when two objects share a common set of components, such as a graphical user interface.
Advantages | Considerations |
---|---|
-Minimizes memory usage by sharing common components -Allows for easy modification of shared components | -Can be challenging to implement and maintain sharing mechanisms -Shared components may not always fit the requirements of each object |
Strategy 3: Hierarchical Composition
Hierarchical composition involves building composite objects using a combination of recursive and shared composition. This strategy offers a balance between flexibility and efficiency.
Advantages | Considerations |
---|---|
-Offers a balance between flexibility and efficiency -Allows for easy modification and extension of composite objects | -Can be complex to implement and maintain -May require additional mechanisms for sharing and updating components |
When building composite objects, it is important to consider the specific requirements of your project and choose the strategy that best fits those needs.
Advantages and Considerations
Composite patterns, including object composition, object aggregation, and object inheritance, offer numerous benefits in software development. By building complex objects from simpler ones, you can achieve code reuse and flexibility in object manipulation. However, there are also some considerations to keep in mind when working with composite patterns.
Advantages
- Code reuse: By reusing existing objects to build new ones, you can save time and effort in software development.
- Flexibility in object manipulation: With composite patterns, you can easily modify and manipulate objects at runtime, without affecting the rest of the codebase.
- Scalability: Composite patterns allow you to add or remove objects from a composite structure, making it easy to scale your software as needed.
Considerations
While composite patterns offer many advantages, they also introduce some complexities and challenges:
- Object composition vs. object aggregation: Choosing between object composition and object aggregation can be tricky, as each has its own strengths and weaknesses.
- Object inheritance: Using object inheritance in composite patterns can create a tight coupling between objects, which can make code maintenance difficult.
- Managing composite objects: As composite objects become more complex, managing them can become challenging, particularly if the structure changes frequently.
By taking these considerations into account and carefully planning your software architecture, you can fully realize the benefits of composite patterns in your software development projects.
Conclusion
Mastering composite patterns is essential for building complex and scalable objects in software development. By leveraging object composition and aggregation, developers can create flexible and reusable code that can be easily manipulated and maintained.
In this article, we have explored the concept of composite patterns, delved deeper into object composition and aggregation, and discussed the composite design pattern in the context of object-oriented programming. We have also provided practical tips and resources to help you enhance your understanding of this topic.
Key Takeaways
With composite patterns, you can:
- Build complex objects from simpler ones
- Reuse code and reduce redundancy
- Create flexible and scalable objects
However, it’s important to consider the potential challenges of managing composite objects, such as the complexity of the code and the need for careful maintenance. By understanding the advantages and considerations of using composite patterns, you can make informed decisions about when and how to implement them in your software development projects.
Overall, mastering composite patterns is a valuable skill for any software developer. By building objects from other objects, you can create efficient and effective code that is both flexible and scalable. We hope this article has provided you with a solid foundation for exploring composite patterns further and integrating them into your software development projects.
FAQ
Q: What are composite patterns?
A: Composite patterns refer to the practice of building objects from other objects. It involves combining simpler objects to create more complex ones.
Q: What are the benefits of building objects from other objects?
A: Building objects from other objects allows for code reuse, flexibility in object manipulation, and the creation of scalable and modular systems.
Q: What is the composite design pattern?
A: The composite design pattern is a specific implementation of the composite pattern in the context of object-oriented programming. It provides a way to treat individual objects and groups of objects uniformly.
Q: How is the composite design pattern implemented?
A: The composite design pattern is typically implemented using a tree-like structure. There is a common interface or abstract class that represents both individual objects and groups of objects.
Q: Can you provide some examples of where the composite design pattern is commonly used?
A: The composite design pattern is commonly used in software development for scenarios such as organizing file systems, representing hierarchies in graphical user interfaces, and creating complex data structures.
Q: What strategies can be used to build composite objects?
A: Different strategies for building composite objects include object composition, where objects are combined to form a larger object, and object aggregation, where objects are grouped together without forming a hierarchical structure.
Q: What are the advantages of using composite patterns?
A: Using composite patterns allows for code reuse, flexibility in object manipulation, and the creation of scalable and modular systems.
Q: What are some considerations when using composite patterns?
A: When using composite patterns, it is important to consider the complexity of managing composite objects, potential performance implications, and ensuring proper encapsulation and abstraction.
Q: How can I further enhance my understanding of composite patterns?
A: To further enhance your understanding of composite patterns, you can explore additional resources, such as books and online tutorials, and also practice implementing the composite design pattern in your own projects.