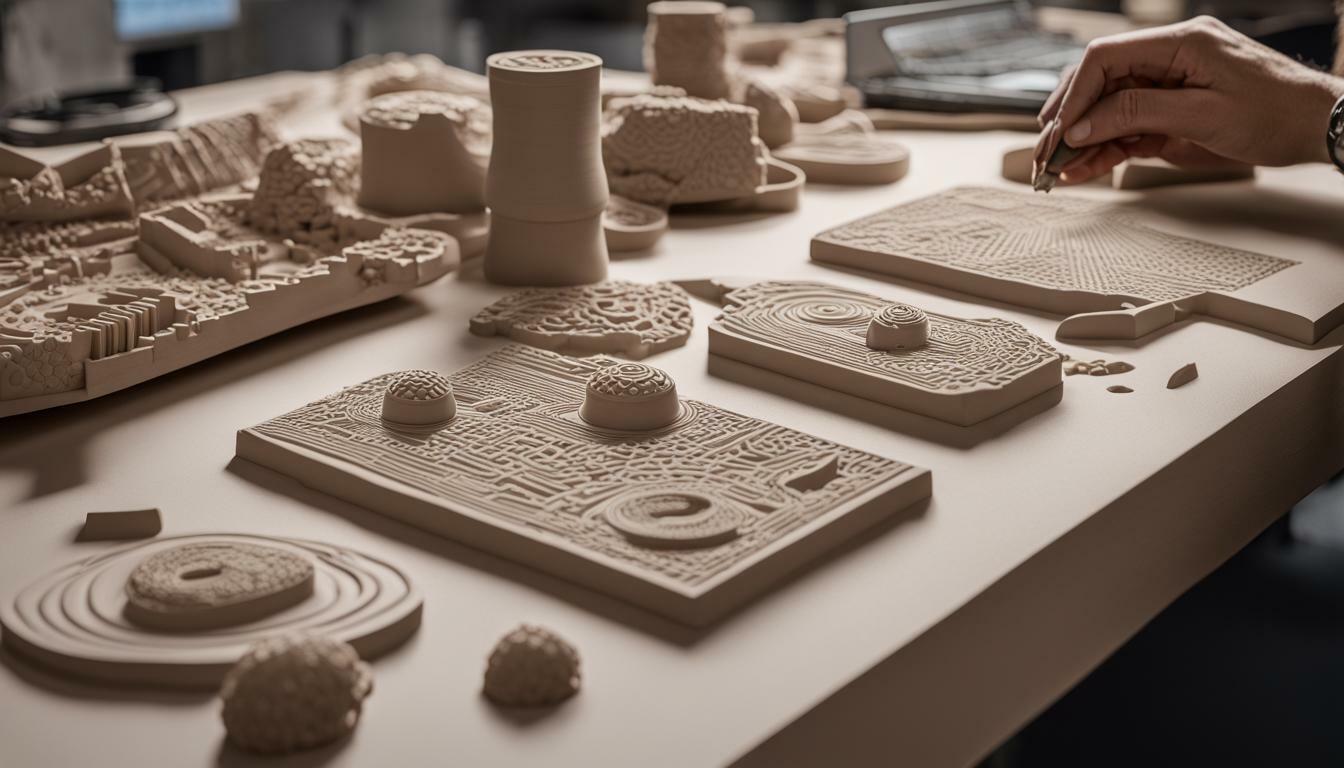
If you’re a software developer, you understand the importance of crafting objects in the most suitable manner – efficient, reusable, and easy to maintain. This is where creational patterns come into play. Creational patterns are design patterns that focus on efficient object creation. They enable developers to create objects in a structured and optimized way, leading to highly maintainable and scalable codebases.
Creational patterns are a fundamental part of Object-Oriented Programming, and mastering them can take your coding skills to the next level. In this article, we’ll explore the different types of creational patterns and the best practices for implementing them in software development. We’ll also discuss the benefits of using design patterns in object-oriented programming and highlight the need for efficient object construction and reusable code.
Key Takeaways:
- Creational patterns are design patterns that focus on efficient object creation.
- They enable developers to create objects in a structured and optimized way.
- Creational patterns are a fundamental part of Object-Oriented Programming.
- Mastering creational patterns can take your coding skills to the next level.
- Using design patterns in object-oriented programming leads to highly maintainable and scalable codebases.
Understanding Creational Patterns
Creational patterns refer to a set of design patterns used in object-oriented programming to support object creation. The primary objective of these patterns is to enhance object creation flexibility and efficiency while reducing system complexity and redundancy.
There are several types of creational patterns, each with its unique approach and applicability in software development. Understanding these patterns and their use cases is essential for building robust and scalable software solutions.
Types of Creational Patterns
The following are the primary types of creational patterns:
- Singleton Pattern: This approach ensures that only one instance of a class is created and allows access to this instance across the entire application.
- Factory Method Pattern: This technique allows a class to delegate object creation to subclasses based on the factory method’s inputs.
- Abstract Factory Pattern: This approach provides an interface for creating related or dependent objects without specifying their concrete classes.
- Builder Pattern: This technique separates the object construction process from its representation, enabling the same construction process to create different representations.
Each of these patterns has its specific advantages and use cases, making them applicable in various software development scenarios.
Implementing Creational Patterns in Object Creation
Implementing creational patterns allows developers to improve object creation efficiency, enhance code reusability, and reduce system complexity. The most suitable creational pattern for a specific software development project depends on various factors such as application requirements, scalability, and flexibility.
Developers should consider the advantages and disadvantages of each creational pattern before implementing it in their project. Furthermore, they must ensure that the pattern aligns with the overall software design architecture and provides the necessary object creation flexibility and scalability.
Conclusion
Understanding creational patterns and their role in object creation is crucial for building efficient and robust software solutions. Implementing these patterns into software development projects enhances code reusability and reduces system complexity while improving object creation efficiency.
Key Object Creation Techniques
When creating objects, it’s important to use efficient and reusable techniques. Creational patterns provide a set of proven approaches for object creation. Let’s explore some key techniques:
Factory Method
The factory method pattern is one of the most commonly used creational patterns. It provides a way to create objects without specifying their concrete classes. This allows for greater flexibility and code reusability. The pattern involves defining a creator class that acts as a factory for producing objects of various types. The creator class may have a method that creates objects based on input parameters, or it may use a template method to create objects using a predefined algorithm.
The factory method pattern is particularly useful when creating families of objects that share common characteristics. In this case, the creator class can be implemented as an abstract class, with concrete subclasses implementing the actual creation logic for each family of objects.
Abstract Factory
The abstract factory pattern provides a way to create families of related objects without specifying their concrete classes. It’s a higher-level pattern than the factory method, as it deals with creating multiple objects that are related to each other. The pattern involves defining a set of factory classes, each of which is responsible for creating a family of related objects.
For example, an abstract factory for creating GUI components might define factories for creating buttons, labels, and text fields. Each factory would create components that are designed to work together and have a common look and feel.
Builder
The builder pattern provides a way to create complex objects using a step-by-step approach. It involves defining a builder class that takes care of the object construction process, while allowing the client to specify the details of the object being created.
This pattern is useful when creating objects that have multiple parts or complex initialization requirements. The builder class can manage the creation of each part of the object and ensure that the final product is created in a consistent and reliable manner.
Singleton
The singleton pattern ensures that only one instance of a class is created within an application. This is useful when you need to ensure that there is only one instance of a resource, such as a database connection or a configuration object.
The pattern involves defining a class that has a single instance and a global point of access. The class constructor is made private to prevent the creation of additional instances, and the single instance is accessed through a static method.
Overall, these object creation techniques provide developers with efficient and reusable ways to create objects. By utilizing these patterns, programmers can improve code quality, increase code reuse, and streamline software development.
Implementing Creational Patterns in Practice
Applying creational patterns in software development can lead to code reusability, efficient object creation and overall better program structure. However, successful implementation requires an understanding of the correct techniques and best practices.
Real-world examples
The factory method pattern is a popular choice for object creation, especially when dealing with varying object types. By separating object instantiation from the application logic, the factory method provides code flexibility and reusability. An example of this is seen in the creation of a document management system where different types of documents such as PDF, Word, and Excel are created using the same factory method with different parameters passed in.
The abstract factory pattern is another technique for organizing object creation. Rather than relying on a single factory method, this pattern creates a family of related objects. This is useful when creating products that have multiple variations, such as a furniture store that produces different styles of chairs, tables, and sofas. By using abstract factory pattern, the store can produce product lines with related variants instead of having a separate factory for each product.
Common pitfalls
Despite its benefits, improper use of creational patterns can lead to performance issues and bloated codebases. One of the most significant pitfalls is premature optimization, where developers choose complex patterns before they are needed, leading to code that is difficult to maintain. Another pitfall is having too many singletons, which can hinder unit testing and make code more prone to errors. Developers should carefully consider the applicability of each pattern before implementation.
Best practices
When implementing creational patterns, it’s essential to follow best practices to ensure optimal performance and code maintainability. One of the best practices is to encapsulate object creation logic within the pattern classes. This ensures that the code is modular, well-organized, and easy to maintain. Another best practice is to use dependency injection, which allows for more flexible and testable code. With dependency injection, objects can be passed around as dependencies instead of being created inside classes.
Conclusion
In summary, creational patterns are an essential element of crafting objects in a suitable manner in software development. By utilizing design patterns such as factory method, abstract factory, builder, and singleton patterns, developers can ensure efficient object construction and increase code reusability. The benefits of implementing creational patterns in software development cannot be overstated.
Incorporating creational patterns in your programming skills can improve your efficiency and effectiveness in creating objects, leading to the development of better applications. It is important to note that there are potential pitfalls when applying creational patterns, and developers should be aware of these and adhere to best practices in their implementation.
In conclusion, creational patterns are a vital aspect of crafting objects in software development. By incorporating them into your development projects, you can enhance your programming skills and create better applications. Always strive to use creational patterns in a suitable manner to maximize the benefits they offer.
FAQ
Q: What are creational patterns?
A: Creational patterns are design patterns that focus on the creation of objects in a suitable manner. They provide guidelines and best practices for efficiently constructing objects in object-oriented programming.
Q: Why are creational patterns important in software development?
A: Creational patterns are important in software development because they promote code reusability and efficient object creation. By following creational patterns, developers can ensure that object construction is done in a consistent and optimal way.
Q: What are the different types of creational patterns?
A: There are several types of creational patterns, including factory method, abstract factory, builder, and singleton patterns. Each pattern has its own advantages and use cases, allowing developers to choose the most suitable technique for their specific application.
Q: How can I implement creational patterns in my software projects?
A: To implement creational patterns, you can follow the guidelines and techniques provided by each pattern. It is important to understand the principles and best practices of each pattern and apply them appropriately in your code.
Q: What are some common pitfalls when implementing creational patterns?
A: Common pitfalls when implementing creational patterns include overcomplicating the code, not considering future changes, and not properly understanding the pattern’s intent. It is important to carefully plan and design your implementation to avoid these pitfalls.