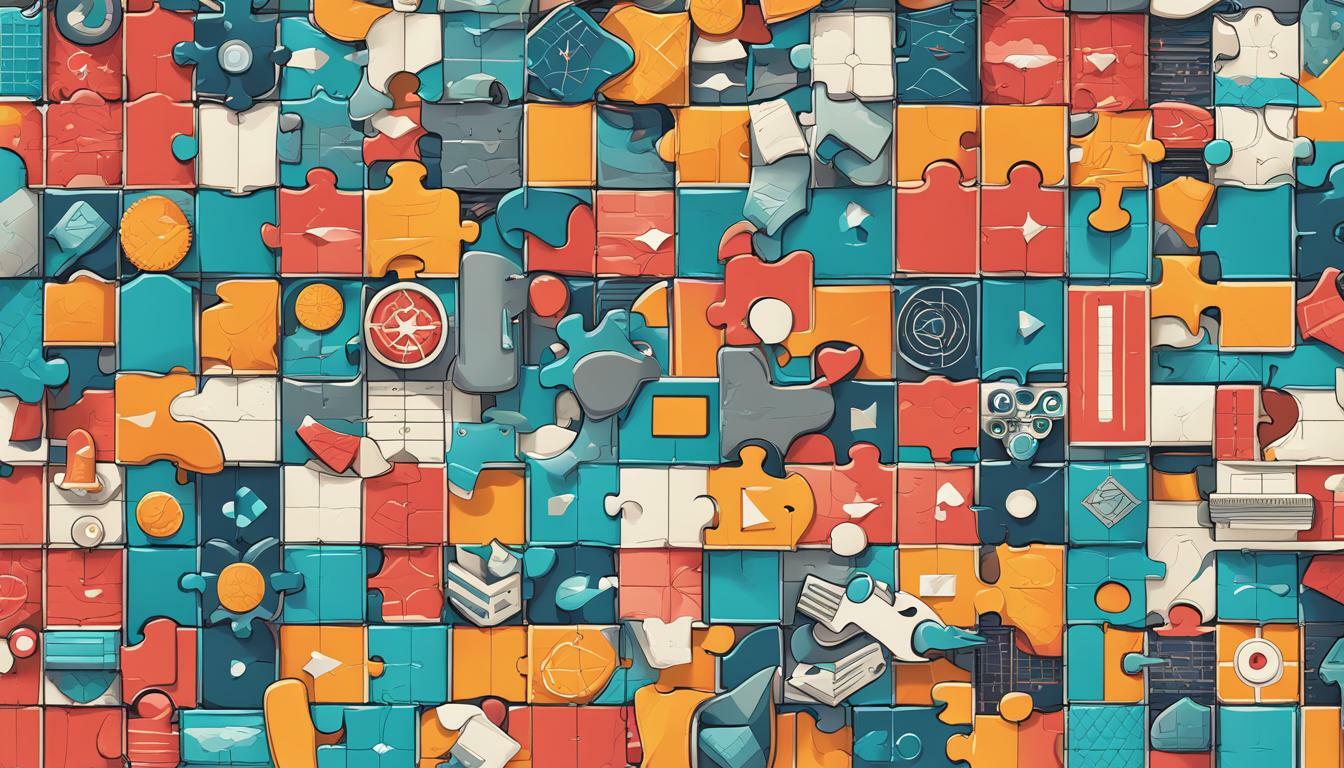
Software design patterns are essential for building robust and maintainable software. They are repeatable solutions to common software design problems that have been tested and proven over time. By incorporating design patterns in your software development workflow, you can improve code quality, increase reusability, and save time and effort in the long run.
In this section, we will provide an introduction to software design patterns, exploring the basics and why mastering them is crucial for software development.
Key Takeaways:
- Software design patterns are repeatable solutions to common software design problems.
- Mastering the basics of software design patterns is crucial for building robust and maintainable software.
- Incorporating design patterns in your software development workflow can improve code quality and increase reusability.
Understanding Software Design Patterns
Software design patterns are essential building blocks for software development. They provide reusable solutions to common programming problems and are integral to building maintainable, efficient, and scalable software. Mastering software design patterns requires an in-depth understanding of their purpose and implementation.
Learning software design patterns is not just about memorizing syntax or code snippets. It is about understanding the bigger picture of software development and designing software with a strategic approach. By mastering software design patterns, you can elevate your skills as a software developer and improve the quality of your code.
What are software design patterns?
Software design patterns are solutions to recurring software development problems, presented in a reusable format. These patterns can be applied to different programming languages and platforms and can address issues related to code structure, architecture, and functionality. Software design patterns are divided into three categories: creational, structural, and behavioral.
Why mastering software design patterns matters?
Mastering software design patterns is crucial for building robust and maintainable software. By understanding the different types of software design patterns, you will be able to apply them effectively in your own projects, improving code quality and reusability. Knowing how to implement software design patterns can also help you avoid common pitfalls in software development, such as spaghetti code or unmaintainable software. Additionally, gaining expertise in software design patterns can help you work more efficiently in a team environment, as you can better communicate and collaborate with other developers.
How to master software design patterns?
Learning software design patterns involves both theoretical knowledge and practical experience. Start by understanding the basics of software design patterns and their different types. Then, explore real-world scenarios where different software design patterns are used and study their implementation. By continuously practicing and applying software design patterns in your own projects, you will become more confident in using them.
The Importance of a Solid Foundation
Mastering software design patterns requires a solid understanding of the basics. It is crucial to establish a strong foundation before attempting to apply more advanced patterns. By starting with the basics of software design patterns, you will gain a clear understanding of the principles and patterns that are fundamental to software development.
Without a strong foundation, attempting to use more advanced patterns can lead to confusion and errors in software development projects. This can result in code that is difficult to maintain and improve. By mastering the basics of software design patterns, you will avoid common pitfalls and be better equipped to tackle complex design challenges.
Learning the basics of software design patterns is an ongoing process. It requires continuous practice and exploration to build a solid foundation. By being patient and persistent in your efforts to master software design patterns, you will elevate your skills as a software developer, and be able to create robust and maintainable software.
Common Software Design Patterns
Software design patterns are reusable solutions to common software design problems. By applying these patterns, developers can create software that is more modular, flexible, and maintainable. In this section, we will discuss some of the most commonly used software design patterns and their applications.
Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to that instance. This pattern is useful when you need to restrict the instantiation of a class to a single object, such as a database connection.
Advantages | Disadvantages |
---|---|
– Provides a global point of access to a single instance | – Can lead to tight coupling between classes |
– Reduces the number of instances created | – Can make debugging more difficult |
Factory Method Pattern
The Factory Method Pattern defines an interface for creating objects, but allows subclasses to decide which class to instantiate. This pattern is useful when you need to delegate the responsibility of object creation to a subclass, while still ensuring that the parent class remains abstract.
“The Factory Method pattern is analogous to a real-world factory, where raw materials go in one end and finished products come out the other. The factory itself doesn’t care about the specifics of the products being produced – it just knows how to create them.”
Observer Pattern
The Observer Pattern defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically. This pattern is useful when you need to maintain consistency across multiple objects that depend on a single object.
Advantages | Disadvantages |
---|---|
– Reduces coupling between objects | – Can result in complex communication between objects |
– Supports broadcast communication between objects | – Can result in performance issues with large numbers of observers |
Decorator Pattern
The Decorator Pattern attaches additional responsibilities to an object dynamically, without changing its original structure. This pattern is useful when you need to add functionality to an object at runtime, without modifying the original object.
- Define a component interface that all classes must implement.
- Create a concrete component class that implements the component interface.
- Create one or more decorator classes that also implement the component interface.
- Each decorator class contains an instance of the concrete component and adds its own functionality.
- Clients can create objects of the concrete component and “wrap” them with one or more decorators.
By familiarizing yourself with these common software design patterns, you will be able to make informed decisions when designing your software. Additionally, implementing these patterns in your own code will improve the quality, flexibility, and maintainability of your software.
Implementing Software Design Patterns
Now that you have a solid understanding of software design patterns, it’s time to put that knowledge into practice. Implementing design patterns in your code can be challenging, but it’s a valuable skill that will make your code more maintainable and robust.
Best Practices
When implementing software design patterns, it’s essential to follow best practices to ensure that your code is clean, modular, and easy to understand. Here are some tips to keep in mind:
- Choose the right pattern for the job: Make sure you’re selecting the pattern that best fits your problem.
- Follow the pattern’s structure: Stick to the pattern’s structure and conventions to maintain consistency.
- Keep it simple: Don’t overcomplicate your implementation – simplicity leads to maintainable code.
- Test your code: Test your implementation thoroughly to ensure that it works as intended.
Potential Challenges
When implementing software design patterns, you may encounter some challenges. Here are a few common ones:
- Overuse: Using too many design patterns can make your code overly complex and difficult to maintain.
- Learning curve: Some design patterns may have a steeper learning curve, but with practice and experience, you’ll become more comfortable with them.
- Team collaboration: It’s essential to ensure that all team members have a solid understanding of the patterns being used to avoid confusion and inconsistency.
Tips for Effective Implementation
Implementing software design patterns effectively can be challenging, but with some tips, you can ensure that your code is clean and maintainable:
“Always strive to use the simplest pattern that will get the job done – don’t overcomplicate your implementation.”
- Start with the basics: Begin by implementing the simpler design patterns and gradually move on to the more complex ones.
- Regular practice: The more frequently you use design patterns, the more comfortable you’ll become with them.
- Document your code: Always document your implementation clearly, so other team members can easily understand it.
By following these tips, you’ll create solid and effective software designs that are easier to maintain and expand.
Conclusion
In conclusion, software design patterns are an essential aspect of software development. By mastering the basics of software design patterns, you can build robust and maintainable software that meets requirements and delivers value. Understanding the different types of software design patterns, such as creational, structural, and behavioral patterns, is fundamental to effective implementation in your code. Establishing a solid foundation in the basics is crucial for effectively applying more advanced design patterns and avoiding common pitfalls.
Implementing software design patterns can be challenging, but following best practices and tips can help you apply them effectively in your projects. By gaining hands-on experience in implementing design patterns, you will solidify your understanding and be confident in applying them in your projects.
Overall, software design patterns are a valuable tool to make your software development process more efficient and effective. Therefore, it is imperative to continue exploring and practicing software design patterns to advance your skills as a software developer. Start with an introduction to software design patterns, and you will soon be on your way to mastering them!
FAQ
Q: What are software design patterns?
A: Software design patterns are proven solutions to common problems that occur during software development. They are reusable templates that help developers design software that is robust, maintainable, and scalable.
Q: Why are software design patterns important?
A: Software design patterns are important because they provide a structured approach to solving common software development problems. They promote code reusability, improve maintainability, and enhance software scalability.
Q: How can mastering software design patterns benefit me?
A: Mastering software design patterns can benefit you by improving your software development skills, making your code more efficient and easier to maintain, and enhancing your problem-solving abilities in software design.
Q: What is the difference between learning and mastering software design patterns?
A: Learning software design patterns involves understanding the different patterns and how they are used. Mastering software design patterns goes beyond that, as it involves applying the patterns effectively, adapting them to specific project requirements, and avoiding common pitfalls.
Q: How can I apply software design patterns in my own projects?
A: To apply software design patterns in your projects, you need to first understand the specific pattern that suits your project needs. Then, you can implement the pattern by following best practices and guidelines associated with that pattern.
Q: What are some common software design patterns?
A: Some common software design patterns include the Singleton pattern, Factory pattern, Observer pattern, and Composite pattern. These patterns address different software development scenarios and provide solutions to specific problems.
Q: What challenges can I expect when implementing software design patterns?
A: When implementing software design patterns, you may face challenges such as selecting the right pattern for your project, integrating the pattern with existing code, and ensuring that the pattern doesn’t introduce unnecessary complexity. However, by following best practices and seeking guidance from experienced developers, you can overcome these challenges.
Q: How can I gain hands-on experience with implementing software design patterns?
A: To gain hands-on experience with implementing software design patterns, you can start with small projects and gradually introduce patterns into your code. You can also participate in coding exercises and collaborate with other developers to learn from their experiences and insights.