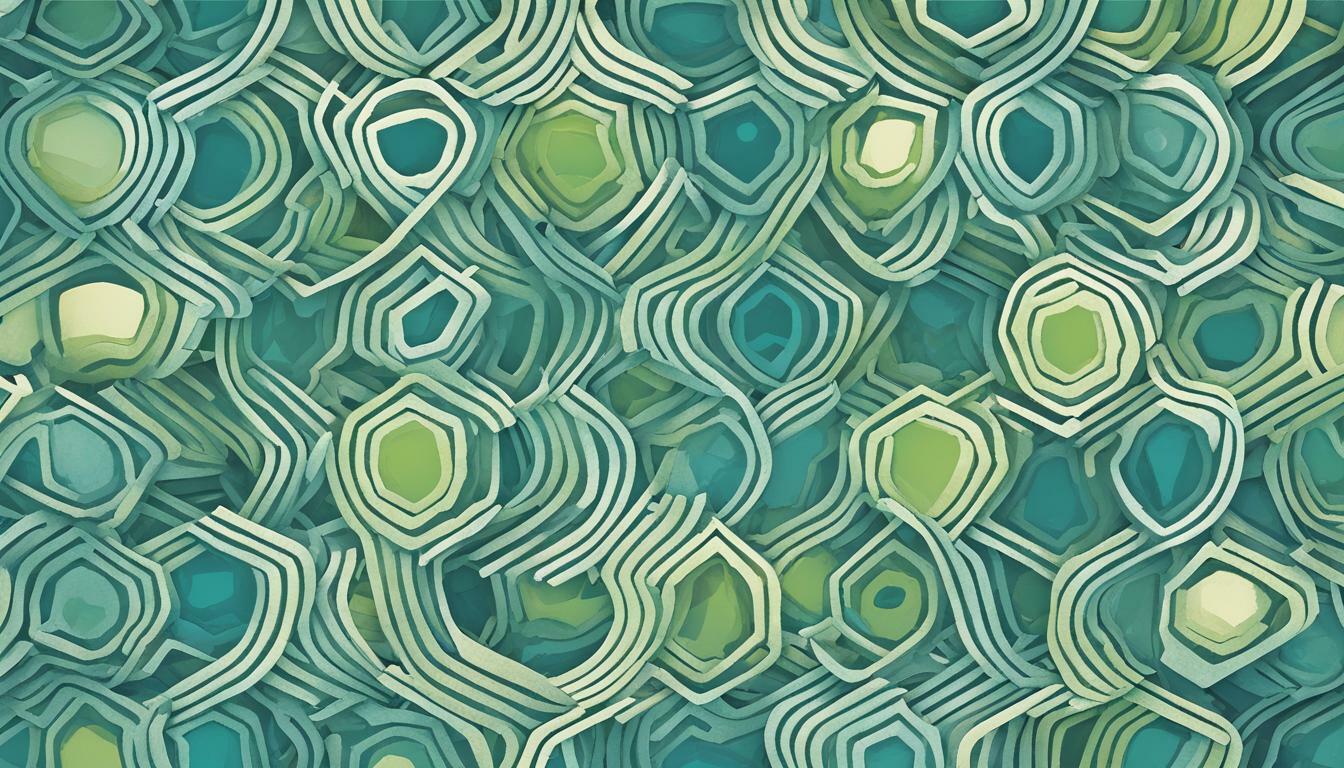
Welcome to our article on adopting standards in .NET APIs for improved consistency and clarity. As any software developer knows, adhering to best practices is crucial for producing high-quality code that is easy to maintain and troubleshoot. By adopting standards, developers can ensure that their code is consistent and that others can easily understand it. In this article, we will explore the benefits of standardization in API development, provide examples of its impact in the real world, and offer best practices for achieving consistency and clarity in .NET coding. Let’s dive in!
The Importance of Standardization in .NET APIs
In the world of API development, adhering to consistent standards is critical for producing high-quality code. Consistency makes code more readable, enhances collaboration among developers, and simplifies maintenance and troubleshooting. With standardized coding practices, developers can create APIs that are well-designed, efficient, and scalable.
In .NET API development, adopting standards is even more crucial. The .NET framework provides a powerful set of tools and libraries that can streamline the development process, but to take full advantage of these features, developers must follow best practices and adhere to established standards.
Adopting standardized practices in .NET coding helps ensure that APIs are built to last. By maintaining consistency throughout the development process, developers can create APIs that are compatible with other software systems and can adapt to changes in technology over time. Standardization also helps ensure that APIs are well-documented, making it easier for other developers to understand and use the code.
Ultimately, the importance of adopting standards in .NET APIs lies in the fact that it helps developers produce high-quality code that performs well, is easy to maintain, and can scale to meet the demands of modern software development.
Benefits of Consistency in .NET APIs
Consistency is essential in .NET API development. It means adhering to standards across all aspects of development, including naming conventions, parameter orders, and return types. This approach leads to improved user experience, reduces errors, and simplifies maintenance and scalability.
Consistency in coding styles and documentation enables developers to comprehend code easier and work collaboratively without confusion. This standardization in .NET API development ensures that developers can build upon each other’s work, thus increasing performance and efficiency.
Furthermore, consistency minimizes errors and reduces the possibility of introducing bugs into the software. When each function has a predictable and consistent output, bugs and errors are easier to detect and fix. This leads to a quicker development cycle and a better user experience.
The importance of consistency in .NET APIs cannot be overstated. By adopting standards in API development, developers ensure that the software they create is robust, streamlined, and easily maintainable.
Achieving Clarity in .NET APIs
In API development, clarity is essential for effective communication between developers and users. Clear and concise code is easier to understand, maintain, and troubleshoot. Adopting standards in .NET coding contributes to the achievement of clarity in APIs.
“Code is read much more often than it is written.” – Guido van Rossum, Python language author
Meaningful naming conventions are key to writing clear code. Names should be concise, descriptive, and self-explanatory. This facilitates ease of understanding and reduces the need for extensive documentation.
var customerName = “John Doe”; // a clear, descriptive name for a variable
Another way to achieve clarity is by providing thorough documentation for the API. Documentation should be comprehensive, organized, and easy to navigate. Additionally, adhering to established design patterns can increase code clarity and readability.
Let’s take a look at an example of clear API communication. The Stripe API follows naming conventions and provides clear documentation, making it easy to understand and use.
Stripe API Method | Description |
---|---|
createCustomer | Create a new customer object. |
deleteCustomer | Delete a customer object. |
The use of meaningful method names and clear descriptions in the Stripe API makes it simple for developers to understand and use the API.
In conclusion, achieving clarity in .NET APIs is important for effective communication and understanding. Adopting standards in .NET coding, such as meaningful naming conventions, thorough documentation, and adherence to established design patterns are essential for achieving clarity in APIs.
Real-World Examples of .NET API Standardization
Adopting standards in .NET APIs has led to significant improvements in consistency and clarity, resulting in more efficient development processes, reduced maintenance effort, and enhanced user satisfaction. Let’s take a look at some real-world examples:
Example One: Code Readability Improvement
A software development team was struggling with maintaining an ASP.NET Web API due to inconsistent code practices. Adopting standardized naming conventions, coding styles, and versioning guidelines led to a significant improvement in code readability. The standardized code reduced the time required for new team members to come up to speed and streamlined the maintenance process.
Example Two: Error Reduction
Another software development team was experiencing an increase in errors and bugs due to inconsistent error handling practices across multiple APIs. Adopting a consistent approach to error handling, including error response formats and HTTP status codes, reduced the number of errors and improved overall API reliability.
Example Three: Scalability Enhancement
A large e-commerce platform had several APIs that were growing at different rates and required frequent updates. Adopting a consistent approach to API design, including versioning and documentation practices, made it easier to scale and maintain the APIs. The platform was able to handle increased traffic and maintain a high level of uptime, resulting in enhanced user satisfaction.
These real-world examples demonstrate the importance of adopting standards in .NET APIs for consistency and clarity. By following best practices and industry standards, software development teams can enhance the user experience, reduce errors, and facilitate scalability.
Best Practices for Adopting .NET API Standards
If you want to achieve consistency and clarity in your .NET APIs, adopting standards is essential. However, simply following standards is not enough. You need to implement them effectively to ensure that your APIs meet their objectives. Here are some best practices for adopting .NET API standards:
- Stick to naming conventions: Use clear and concise names that reflect the functionality of your APIs. Follow a consistent naming convention to ensure that your APIs are easy to understand and use.
- Organize your code: Keep your code organized and easy to navigate. Use logical groupings, such as namespaces and classes, to make it easier for developers to find what they need.
- Handle errors effectively: Error handling is crucial in API development. Use consistent error codes and messages to ensure that developers can easily identify and fix issues.
- Version your APIs: Use versioning to ensure that newer versions of your APIs will not break existing clients. Follow consistent versioning practices to make it easy for developers to determine which version to use.
- Document your code: Provide clear and comprehensive documentation for your APIs. Describe how to use each API method and provide examples to illustrate their usage.
- Follow established design patterns: Use established design patterns to ensure that your APIs are consistent and easy to use. This will make it easier for developers to learn and use your APIs.
- Test your APIs: Thoroughly test your APIs to ensure that they function correctly and meet your requirements. Use automated testing whenever possible to save time and effort.
By following these best practices, you can ensure that your .NET APIs are consistent, clear, and easy to use. This will lead to better collaboration among developers, reduced maintenance effort, and improved user satisfaction.
The Evolution of .NET Standards in API Development
In the rapidly evolving world of software development, it’s essential to keep up with industry trends and embrace new standards to ensure the longevity and compatibility of APIs. The .NET framework is no exception. .NET standards have undergone significant changes over the years as developers have sought to improve the consistency and clarity of their APIs.
In recent years, there has been a shift towards adopting RESTful APIs, which provide a standardized way of communicating with web services. This shift has led to the emergence of new standards such as OpenAPI, which is now widely used to document RESTful APIs.
The .NET community has also made significant contributions to the development of standards. For example, the .NET Standard was introduced to unify the APIs of different .NET implementations.
Moreover, Microsoft has been actively involved in the development of API standards through its participation in organizations such as the .NET Foundation and the OpenAPI Initiative. The .NET Foundation, for example, provides a platform for discussion and collaboration among .NET developers and encourages the development of open-source projects.
In conclusion, the evolution of .NET standards in API development has been driven by a desire to improve consistency, clarity, and compatibility. By staying up to date with industry trends and embracing new standards, developers can ensure the longevity and relevance of their APIs in the rapidly changing world of software development.
Conclusion
In summary, adopting standards in .NET APIs is crucial for achieving consistency and clarity in API development. Best practices such as naming conventions, code organization, error handling, and versioning are essential for achieving standardization.
Consistency leads to a better user experience, reduces errors, and facilitates scalability. Clear and concise code, thorough documentation, and established design patterns enhance code readability, improve collaboration among developers, and simplify maintenance and troubleshooting.
Real-world examples showcase the transformative impact of standardized API development, resulting in more efficient development processes, reduced maintenance effort, and enhanced user satisfaction.
Staying up to date with industry trends and embracing new standards is crucial for ensuring the longevity and compatibility of APIs. Frameworks and libraries play a significant role in achieving standardized coding practices.
In conclusion, adopting standards in .NET APIs is essential for software development success. By following best practices and implementing consistent and clear API design, developers can achieve significant benefits and positively impact the software industry.