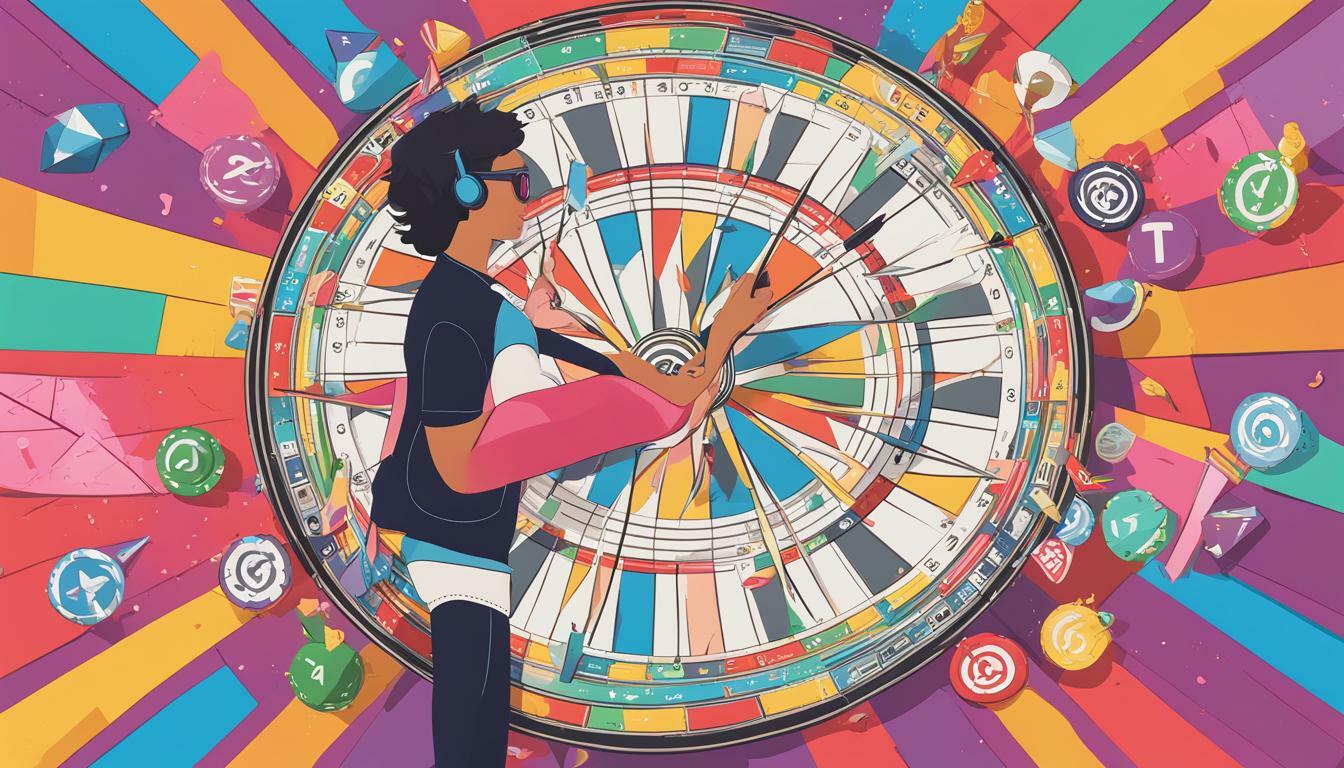
Welcome to the exciting world of Flutter development – where creativity meets technology and innovation. As a Flutter developer, it’s important to stay on top of your game by following Dart’s top development practices. These practices encompass everything from coding guidelines and standards to programming tips and optimization techniques that can help you write cleaner, more efficient code in Flutter apps.
If you’re looking to improve your Flutter development skills and perfect the art of Flutter, then you’re in the right place. In this article, we’ll explore the various best practices for Dart development that every Flutter developer should know. From Flutter coding guidelines to Dart programming tips, we’ll cover it all. We’ll also discuss how to optimize your Dart code for the best possible performance in Flutter apps and provide practical Flutter development tips and tricks to streamline your development process. Finally, we’ll showcase real-world examples of how to apply these practices in your Flutter app development projects.
So let’s get started on our journey to perfecting the art of Flutter development by embracing Dart’s top development practices!
Understanding Dart Development Best Practices
Developers who want to write efficient and maintainable code in Flutter apps should follow the best practices for Dart development. These practices ensure that your code is clean, easy to read, and performs well. Here are some Dart programming tips and coding guidelines that you should keep in mind.
Use Descriptive and Meaningful Names for Variables
When naming variables, use descriptive and meaningful names that reflect the purpose and context of the variable. Avoid using generic names like “temp” or “data,” as they do not provide any useful information for anyone reading the code. Instead, use names that convey the specific use of the variable, like “totalPrice” or “customerName.”
Follow Consistent Formatting and Coding Standards
Consistency in formatting and coding standards can significantly improve the readability and maintainability of your code. Adopt a consistent style and formatting for your codebase, such as how you name variables, where you put braces, or how you use whitespace, among others. Most importantly, ensure all team members are aligned with the coding standards.
Document your Code
Documenting your code is essential to ensure that other developers can understand and maintain your code. Write clear and concise comments that describe the purpose and functionality of the code. Use tools like documentation generators to automate the process.
Use Testing Frameworks for Automated Testing
Testing your code is essential to ensure that it is working correctly and efficiently. Use testing frameworks like Flutter’s built-in testing framework or Dart’s testing package to write automated tests for your code. This will help you catch bugs early, enhance code quality, and speed up the development process.
By following these best practices, you can write clean, efficient, and maintainable code in Dart for Flutter apps. With consistent effort and practice, you can become a proficient and skilled Flutter developer, continuously improving your skills and embracing new technologies.
Optimizing Dart Code for Flutter Performance
Dart is a powerful programming language, and optimizing code is paramount to ensuring smooth performance of Flutter apps. To optimize your Dart code, there are several techniques and strategies that you can use to enhance the overall performance and user experience of your apps.
Dart Code Optimization Techniques
One technique for optimizing your Dart code is to use the const keyword, which allows for the creation of constants at compile-time instead of runtime. This can improve performance by reducing the need for expensive calculations and allocations during app execution.
Another technique is to use the final keyword for variables and functions that will not change during the app’s runtime. This helps to reduce memory usage and improve performance by eliminating unnecessary allocations.
Flutter Performance Techniques
In addition to optimizing your Dart code, there are also several performance techniques that you can use to enhance your Flutter apps. One of these techniques is to use the const constructor in Flutter widgets, which can help reduce the need for widget rebuilds and improve app performance.
Another technique is to use asynchronous programming with Futures and Streams to ensure that your app remains responsive and performs well, even when executing time-consuming tasks.
Summary
Optimizing your Dart code and utilizing performance techniques in Flutter can greatly improve the overall performance and user experience of your apps. By using techniques such as the const and final keywords, as well as asynchronous programming and the const constructor in Flutter widgets, you can create clean and efficient code that runs smoothly and provides a seamless experience for your users.
Utilizing Flutter Development Tips and Tricks
Developing a Flutter app can be a challenging task, but with the right tips and tricks, developers can streamline their workflow and improve their efficiency. Here are some practical tips and guidelines for Flutter development:
1. Follow Flutter Coding Guidelines
Flutter provides a set of coding guidelines that developers should follow to ensure their code is maintainable and easy to read. These guidelines cover areas such as naming conventions, indentation, and spacing. By following these guidelines, developers can make their code more consistent and easier to understand for other developers who may work on the same project.
2. Utilize Flutter’s Built-in Widgets
Flutter provides a wide range of built-in widgets that can help developers build UI components quickly and easily. These widgets cover a range of use cases, from simple buttons to complex layouts and animations. By utilizing these widgets, developers can save time and reduce the amount of code they need to write.
3. Use Flutter Packages
Flutter has a large community of developers who contribute to a wide range of packages and plugins. These packages can provide additional functionality that developers may not find in Flutter’s built-in widgets. By using these packages, developers can save time and focus on building the core functionality of their app.
4. Optimize for Performance
Performance is a critical factor in app development, and Flutter provides a range of features and tools that developers can use to optimize their app’s performance. This includes techniques such as lazy loading, caching, and reducing the number of unnecessary rebuilds. By optimizing for performance, developers can ensure their app runs smoothly and delivers a great user experience.
5. Keep Learning
Flutter is a rapidly evolving platform, and developers should strive to stay updated with the latest developments and best practices. By keeping up to date with the latest news, tutorials, and documentation, developers can ensure they are using Flutter to its full potential and continuously improving their skills.
By following these tips and guidelines, developers can streamline their workflow and improve their efficiency in Flutter app development.
Applying Dart’s Top Development Practices in Real-World Examples
Now that we’ve explored some of the best practices for Dart development and tips for Flutter app development in general, let’s take a look at how we can apply these concepts in real-world examples. By following these practices, we can create cleaner, more efficient, and maintainable code that results in better-performing Flutter apps.
Dart Development Best Practices in Action
One of the key best practices in Dart development is to use typed variables whenever possible. This helps enforce type safety and makes your code more readable. Here’s an example:
// Bad:
var count = 0;
// Good:
int count = 0;
Another best practice is to use named constructors. This makes your code more readable and easier to maintain. Here’s an example:
// Bad:
Person person = new Person("John", 30);
// Good:
Person person = Person.create(name: "John", age: 30);
Flutter Development Tips in Action
When it comes to Flutter app development, one useful tip is to use the built_value package for immutable data models. This can help simplify your code and prevent common errors. Here’s an example:
// Before:
class Person {
String name;
int age;
Person(this.name, this.age);
}
// After:
@BuiltValue(instantiable: false)
abstract class Person implements Built<Person, PersonBuilder> {
String get name;
int get age;
Person._();
factory Person([updates(PersonBuilder b)]) = _\$Person;
}
Another useful tip is to use the Provider package for state management. This can help simplify your code and make it more maintainable. Here’s an example:
// Before:
class Counter extends StatefulWidget {
@override
_CounterState createState() => _CounterState();
}
class _CounterState extends State<Counter> {
int _count = 0;
void _increment() {
setState(() { _count++; });
}
@override
Widget build(BuildContext context) {
return Text('Count: \$_count');
}
}
// After:
class Counter with ChangeNotifier {
int _count = 0;
void increment() {
_count++;
notifyListeners();
}
int get count => _count;
}
class CounterWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Consumer<Counter> (
builder: (context, counter, child) => Text('Count: \${counter.count}'),
);
}
}
Dart Programming Tips in Action
Finally, let’s take a look at a few Dart programming tips in action. One tip is to use extension methods to add functionality to existing classes. This can help simplify your code and make it more readable. Here’s an example:
extension StringExtension on String {
bool get isEmail => ...
}
Another useful tip is to use the cascade operator for chaining method calls. This can help simplify your code and make it more expressive. Here’s an example:
person..setName("John")..setAge(30);
By applying these best practices and tips in real-world examples, we can improve the quality and performance of our Flutter apps. Keep practicing and experimenting with these techniques, and you’ll be well on your way to mastering Dart and Flutter development!
Mastering Dart’s Advanced Features for Flutter Development
While following Dart’s best practices is essential for writing clean and efficient code, mastering its advanced features can take your Flutter development skills to the next level. Here are some tips to help you make the most of Dart’s advanced capabilities:
- Generators: Use generators to efficiently generate potentially infinite sequences of values, such as when reading data from a stream or iterating over large collections.
- Mixins: Mixins allow you to reuse code across multiple classes without having to create complex class hierarchies. Use mixins to simplify your code and make it more reusable.
- Asynchronous programming: Dart provides powerful tools for async programming, such as Futures and the async/await syntax. Use async programming to make your Flutter apps more responsive and efficient.
- Type inference: Dart’s type inference system can automatically deduce the types of variables, allowing you to write more concise and readable code. Use type inference to make your code more efficient and maintainable.
Example: Using Mixins for Code Reuse
Suppose you have multiple classes that need to perform logging. Instead of duplicating the logging code for each class, you can define a mixin that encapsulates the log functionality and apply it to each class:
“`
mixin Loggable {
void log(String message) {
print(‘[${DateTime.now()}] $message’);
}
}class MyClass with Loggable {
void doSomething() {
log(‘Doing something…’);
// …
}
}
“`Now the `MyClass` can perform logging simply by calling the `log()` method from the `Loggable` mixin. This simplifies your code and makes it more reusable.
By mastering these advanced features, you can write more efficient, maintainable, and powerful Flutter apps using Dart. Always keep an eye out for opportunities to leverage these advanced features in your code.
Staying Up-to-Date with Flutter and Dart’s Latest Developments
Keeping up with the latest developments in Flutter and Dart is essential for developers who want to stay ahead of the curve. Here are some tips to help you stay up-to-date:
- Follow official documentation and blogs: The official Flutter and Dart documentation and blogs are a great resource for staying updated. Follow them to learn about new features, updates, and best practices.
- Join online communities: Joining online communities, such as Reddit, Stack Overflow, and Discord, can help you connect with other Flutter and Dart developers. These communities will keep you informed about the latest news, and you can learn from other developers’ experiences.
- Attend conferences and meet-ups: Attending conferences and meet-ups is an excellent way to meet other developers and learn about new technologies. Many conferences have sessions dedicated to Flutter and Dart development.
- Participate in hackathons and challenges: Participating in hackathons and challenges can help you learn new skills and technologies while working with other developers. These events often have prizes and can help you showcase your skills to potential employers.
By staying up-to-date with the latest developments in Flutter and Dart, you can continuously improve your skills and stay ahead of the competition.
Conclusion
Perfecting the art of Flutter development can be achieved by embracing Dart’s top development practices. Applying coding guidelines, standards, and programming tips can result in clean and efficient code, leading to better performance and user experience. In addition, optimizing Dart code specifically for Flutter can enhance the overall app performance.
Utilizing Flutter development tips and tricks can streamline the development process and improve overall efficiency. Applying these tips and techniques can lead to building powerful and feature-rich Flutter apps.
In real-world examples, applying Dart’s top development practices can result in cleaner and more maintainable code. Leveraging Dart’s advanced features can further enhance Flutter app development.
Staying up-to-date with the latest developments in Flutter and Dart is crucial for continuously improving Flutter app development skills. Embracing Dart’s top development practices and continuously learning new techniques can help developers become skilled Flutter developers.
Overall, by following the best practices for Dart development and optimizing code for Flutter, developers can perfect the art of Flutter development and build high-quality Flutter apps. Keep learning and experimenting, and the possibilities are endless.