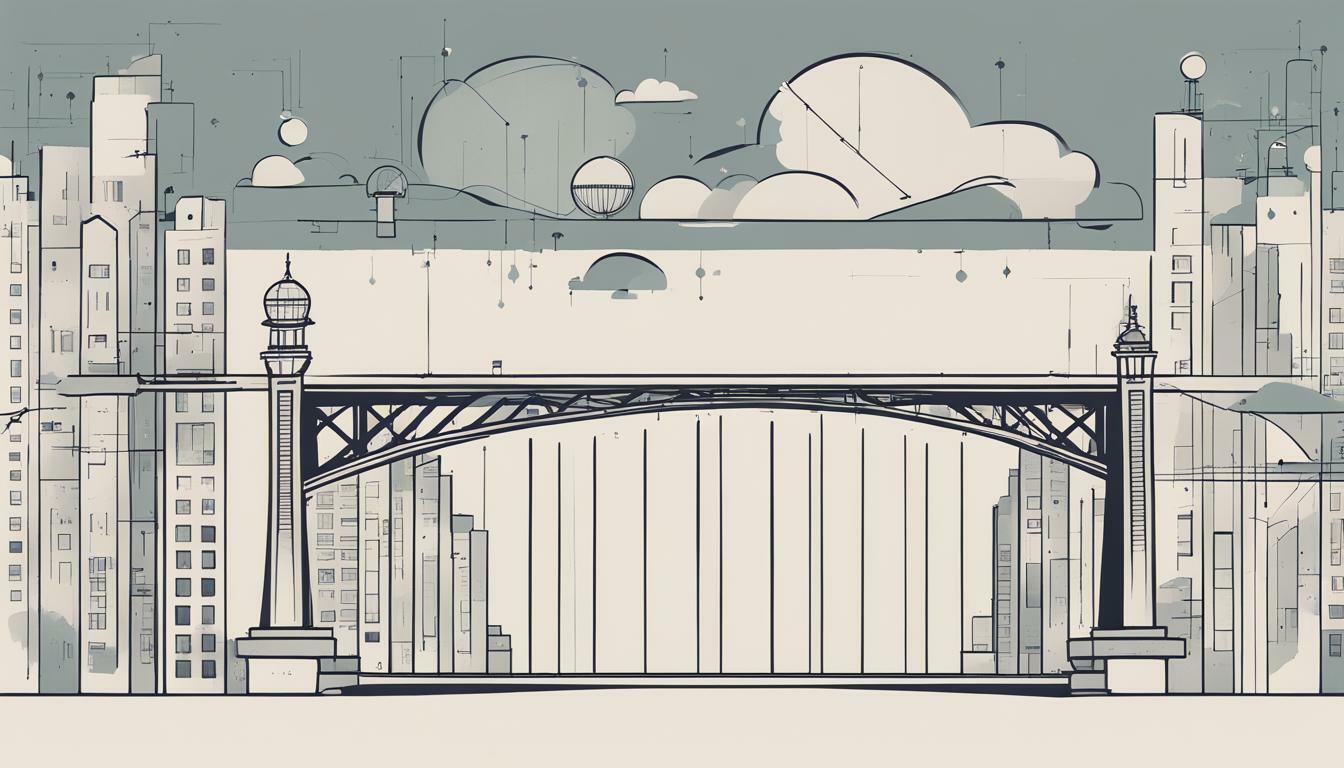
As software engineers, we often come across situations where we need to integrate two systems that have incompatible interfaces. This is where the Adapter Pattern comes in handy. It allows us to create an adapter that acts as a bridge between these incompatible interfaces, enabling them to communicate seamlessly.
In this article, we will explore the Adapter Pattern in detail, starting with its definition and how it works. We will also look at how to implement it in your code, along with some practical use cases.
Key Takeaways:
- The Adapter Pattern bridges the gap between incompatible interfaces.
- It is a design pattern frequently used in Object-Oriented Programming.
Understanding the Adapter Design Pattern
The Adapter Design Pattern is a popular object-oriented programming solution used in software design. It allows for the bridging of incompatible interfaces, making it possible for classes to work together that otherwise cannot due to conflicting interfaces.
The Adapter Pattern acts as an intermediary between two incompatible classes, converting the interface of one class into a form that another class can use. This is particularly useful in software design when pre-existing classes or libraries have incompatible interfaces that cannot be easily modified or changed.
The Adapter Design Pattern is based on the principles of encapsulation and code reusability. As such, it is an excellent tool for developers looking to improve the modularity and scalability of their code. It also allows developers to implement new functionality without having to modify existing code, a key principle of object-oriented programming.
Object-Oriented Programming and the Adapter Pattern
In object-oriented programming, the Adapter Pattern is a fundamental tool for ensuring seamless integration of different classes. By using the Adapter Pattern, developers can build software that is more robust and modular, making it easier to maintain and update over time.
In conclusion, the Adapter Design Pattern is a valuable tool for software developers seeking to improve the compatibility of their code. By allowing for the bridging of incompatible interfaces, the Adapter Pattern facilitates the integration of different classes and libraries, improving the overall modularity, scalability, and flexibility of the software.
Implementing the Adapter Pattern
The Adapter Design Pattern allows developers to use existing code and interface it with incompatible systems, thereby promoting code reusability. This is achieved by creating an adapter class that acts as a bridge between the existing code and the new system. The adapter class modifies the existing code to match the interface of the new system, ensuring interface compatibility.
The key to implementing the Adapter Pattern lies in understanding the design patterns prevalent in object-oriented programming. Design patterns such as inheritance, composition, and polymorphism are essential in creating an adapter class that can communicate seamlessly with both systems.
Creating an adapter class involves identifying the interface of the new system and creating an adapter that matches that interface. This can be done by inheriting the adapter class from a base class or interface and implementing the methods required by the new system.
Once the adapter class has been created, it can be used to interface with the new system, providing a layer of abstraction that ensures compatibility with the existing code. This approach can save developers time, effort, and resources by eliminating the need to rewrite existing code or modify the new system.
Practical Use Cases of the Adapter Pattern
One of the most common scenarios where the Adapter pattern is used is in integrating third-party APIs into existing software systems. Third-party APIs often have different interfaces from the system they are being integrated into, and the adapter pattern allows for the creation of a bridge between the incompatible interfaces.
Another use case for the Adapter pattern is in legacy code systems. When updating or migrating a legacy system, it’s often necessary to integrate new code with old code. However, the old code may have deprecated interfaces that are no longer compatible with the newer code. The adapter pattern can be used to create a bridge between the two interfaces, allowing for seamless integration.
Example 1: Integrating Payment Gateways
Payment gateways often have different interfaces for different payment methods, and integrating them directly with a software system can be a challenge. By using the Adapter pattern, a bridge can be created between the software system and the payment gateway, allowing for seamless payment processing.
Software System Interface | Payment Gateway Interface | Adapter Interface |
---|---|---|
ProcessPayment() | ProcessCreditCardPayment() | ProcessPayment() |
ProcessPayPalPayment() | ProcessPayment() |
In the table above, the software system has a ProcessPayment() method, while the payment gateway has separate methods for processing credit card payments and PayPal payments. The adapter pattern is used to create a bridge between the two interfaces, allowing the software system to process both credit card and PayPal payments using the same ProcessPayment() method.
Example 2: Integrating Third-Party Libraries
When integrating third-party libraries into a software system, it’s often necessary to create an adapter to bridge the gap between the incompatible interfaces. This is particularly true when the third-party library has a different programming language or architecture from the system it’s being integrated into.
For example, a software system written in Java may need to integrate a third-party library written in Python. By creating an adapter that translates the Python interface to the Java interface, the two can be seamlessly integrated.
Example 3: Testing
Another use case for the Adapter pattern is in testing. When testing code, it may be necessary to create mock objects that mimic the behavior of real objects. By using the Adapter pattern to create the mock objects, the testing code can be written without having to worry about the compatibility issues between the mock objects and the real objects.
- Mock Object Interface: mockMethod()
- Real Object Interface: realMethod()
- Adapter Interface: mockMethod() calls realMethod()
In the example above, the mock object has a mockMethod() interface, while the real object has a realMethod() interface. The adapter pattern is used to create an adapter interface that allows the mockMethod() to call the realMethod().
Conclusion
In conclusion, the Adapter Pattern is a valuable tool in bridging the gap between incompatible interfaces. Understanding the principles of the Adapter Design Pattern in Object-Oriented Programming and software design can enhance code reusability and improve interface compatibility. Implementing the Adapter Pattern requires attention to detail and a solid understanding of design patterns.
By providing practical use cases, we hope to have demonstrated the versatility and usefulness of the Adapter Pattern. It can be applied in a variety of scenarios, including legacy code integration, third-party library integration, and interface adaptation for better scalability.
In summary, the Adapter Pattern is a powerful solution to the challenges of interface communication. Its flexibility and adaptability make it a popular choice among developers. When used correctly, it can streamline software development and improve the user experience. Keep the Adapter Pattern in your toolkit and explore its potential in your next project. Thank you for reading!
FAQ
Q: What is the Adapter Pattern?
A: The Adapter Pattern is a design pattern in object-oriented programming that allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces, converting the interface of one class into another interface that clients expect.
Q: How does the Adapter Pattern work?
A: The Adapter Pattern works by creating a class that implements the expected interface and internally uses an object of the class that needs to be adapted. This way, the adapter class can translate the calls from the expected interface into calls to the adapted class.
Q: Why is the Adapter Pattern useful?
A: The Adapter Pattern is useful in situations where we have existing classes with incompatible interfaces and we want them to work together without modifying their source code. It promotes code reusability by allowing the integration of existing classes into new systems.
Q: Can the Adapter Pattern be used with multiple classes?
A: Yes, the Adapter Pattern can be used with multiple classes. Each class would have its own adapter that translates its interface into the expected interface. This allows multiple incompatible classes to work together seamlessly.
Q: Are there any real-world examples of the Adapter Pattern?
A: Yes, there are several real-world examples of the Adapter Pattern. One common example is the use of adapters to connect different electrical devices to power outlets with varying plug types. Another example is the use of adapters in software development to integrate external libraries or APIs.
Q: Is the Adapter Pattern specific to a programming language?
A: No, the Adapter Pattern is a general design pattern that can be implemented in any object-oriented programming language. The concept of adapting incompatible interfaces is applicable across different programming languages.