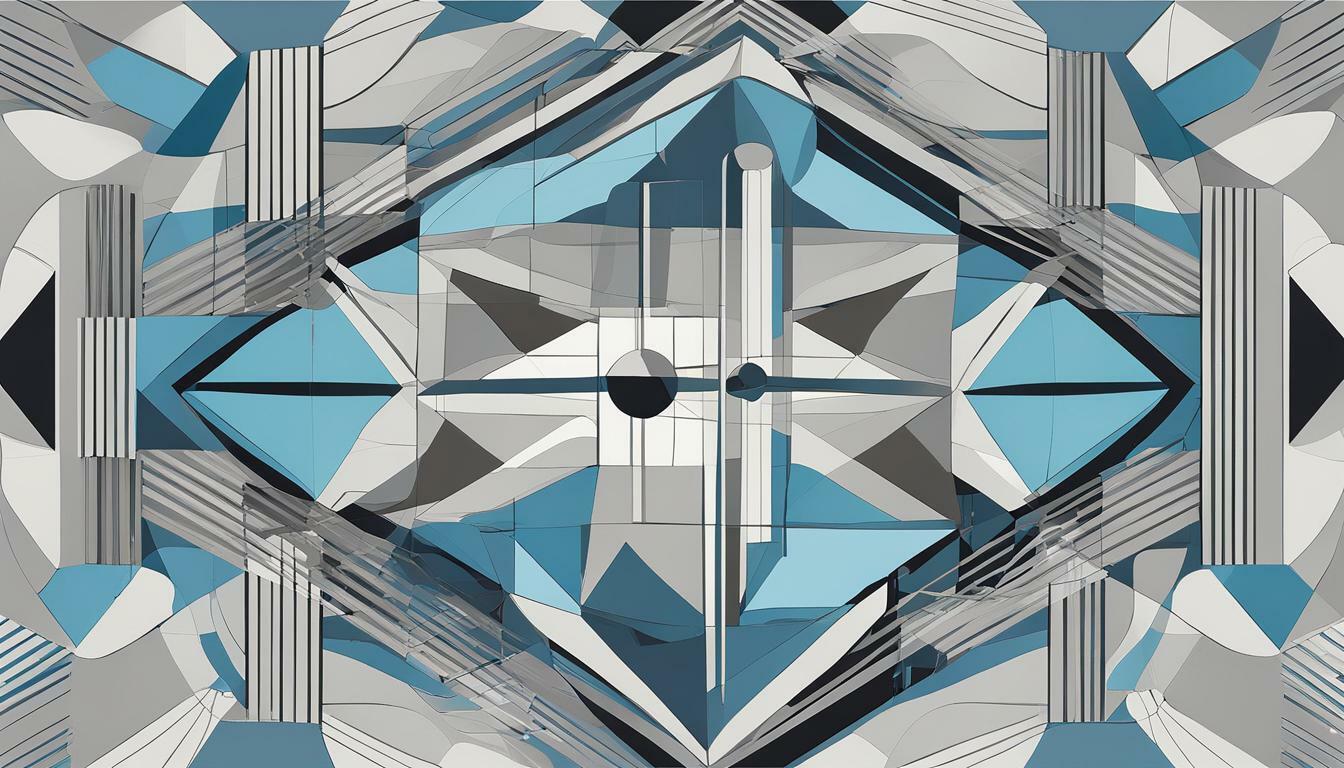
When it comes to software development and object-oriented programming, the Singleton pattern is a popular design pattern that ensures only one instance of an object is created and used throughout the program. This is particularly useful for classes that require encapsulation and a single point of access.
The Singleton pattern involves creating a class that can only be instantiated once, using a private constructor and a static method to access the instance. This ensures that all instances of the class refer to the same object, maintaining consistency and preventing unnecessary duplication.
Key Takeaways:
- The Singleton pattern ensures only one instance of an object is created and used throughout the program.
- It involves creating a class with a private constructor and a static method to access the instance.
- Encapsulation and a single point of access are key benefits of the Singleton pattern.
- To implement the Singleton pattern effectively, best practices should be followed.
Understanding the Singleton Pattern
The Singleton pattern is a popular design pattern in object-oriented programming that ensures the creation of only one instance of a class during runtime. This object instance can then be used across different parts of a software application.
Encapsulation is a key feature of the Singleton pattern, as it ensures that the object instance is hidden from external users and can only be accessed through specific methods or properties. This helps to maintain the integrity of the object and the class it belongs to.
In order to achieve this, the Singleton pattern involves the use of a private constructor, which prevents the creation of additional instances of the class. Instead, a static method is used to access the object instance, which is created only if it does not already exist.
Understanding the Class and Constructor
The Singleton pattern is implemented within a specific class. This class contains the private constructor and a static method that returns the object instance. The constructor is defined as private to ensure that no external code can create an instance of the class.
When the static method is called, it first checks whether an instance of the class already exists. If not, it creates a new instance before returning it to the caller. If an instance already exists, the static method simply returns the existing instance.
This ensures that only one instance of the class is created and used throughout the lifetime of the application.
Implementing the Singleton Pattern in Practice
Now that you understand the concept of the Singleton pattern and its benefits, it’s time to implement it in your software development projects. There are a few best practices to keep in mind when implementing the Singleton pattern.
Firstly, ensure that only one instance of the object exists throughout the application. This can be achieved by controlling the instantiation of the object through the use of a private constructor and a static method to return the instance.
Secondly, consider using lazy instantiation, which means delaying the creation of the object until it’s actually needed. This can help improve performance and optimize code.
It’s important to note that the Singleton pattern should only be used when there’s a genuine need for only one instance of the object. Overusing this pattern can lead to scalability issues and make the codebase harder to maintain.
Lastly, be mindful of thread-safety when implementing the Singleton pattern in multi-threaded applications. You can use synchronization to ensure that only one thread can access the object at a time.
By following these best practices, you can successfully implement the Singleton pattern in your software development projects and reap its benefits.
Benefits of the Singleton Pattern
The Singleton pattern provides a number of benefits that make it a popular choice in software development. One of the main benefits is better performance through optimized code. This is because the Singleton pattern ensures that only one instance of an object exists, reducing the amount of memory and resources used by the application.
By limiting the number of object instances, the Singleton pattern also increases the speed of the application. This is because creating and destroying object instances can be a time-consuming process. With the Singleton pattern, the object is only created once, and subsequent calls to the object retrieve the same instance, making the application faster and more efficient.
The Singleton pattern also promotes better code organization and encapsulation. By controlling the creation of object instances and limiting their access, the Singleton pattern ensures that objects are created and used in a consistent and organized way.
Additionally, the Singleton pattern can help to simplify the debugging process by making it easier to identify and isolate issues related to object creation and usage. This is because with the Singleton pattern, there is only one instance of an object to examine and troubleshoot.
Overall, the Singleton pattern can be an effective tool for improving the performance and organization of your software development projects.
Alternatives to the Singleton Pattern
While the Singleton pattern is a popular and effective design pattern for ensuring there is only one instance of an object, there are alternative patterns that can be used in software development. It is important to consider these alternatives and choose the one that best fits the specific project requirements.
Factory Method Pattern
The Factory Method pattern allows for the creation of objects without specifying their exact class. This can be useful when there are different variations of a class that need to be created based on specific conditions or inputs. The Factory Method pattern can also be used to create a single instance of a class, similar to the Singleton pattern.
However, the Factory Method pattern allows for more flexibility in creating objects since it does not rely on a single static method or variable.
Dependency Injection Pattern
The Dependency Injection pattern involves providing objects with the dependencies or resources they need in order to function correctly. This pattern can be useful for creating objects that rely on other objects or services.
While this pattern does not ensure that there is only one instance of an object, it can improve performance and make code more maintainable.
Builder Pattern
The Builder pattern separates the construction of complex objects from their representation. This can be useful when there are multiple steps involved in creating an object or when there are several variations of an object that need to be created.
While the Builder pattern does not ensure that there is only one instance of an object, it can improve code readability and make it easier to modify the construction process in the future.
Best Practices for Singleton Pattern Usage
While the Singleton pattern has its advantages, it’s important to use it properly to avoid potential pitfalls in your software development. Here are some best practices to keep in mind:
- Ensure thread-safety: Since Singleton objects are shared across the application, it’s important to make sure that they can handle multiple threads accessing them simultaneously. One solution is to make the getInstance() method synchronized, but this can also cause performance issues. Alternatively, you can use the double-checked locking technique or an enum to ensure thread safety.
- Avoid global state: Singletons can easily become a global state in your application, which can lead to problems such as tight coupling and difficulties with testing. It’s best to limit the scope of your Singletons and consider using dependency injection to manage your object instances.
- Follow the Open/Closed Principle: Your Singleton should be closed to modification but open to extension. This means that you should design it in a way that allows you to add new functionality without changing its core implementation. This can be achieved through the use of interfaces and abstract classes.
- Don’t abuse the Singleton pattern: Just because you can use the Singleton pattern, doesn’t mean you always should. It’s important to consider alternative design patterns and approaches that may be more suitable for your specific use case.
By following these best practices, you can ensure that your Singleton pattern implementation is effective, efficient, and maintainable in your software development.
Conclusion
In software development, mastering the Singleton pattern can bring significant benefits in terms of encapsulation and performance optimization. By ensuring only one object instance is created, resources are conserved and unnecessary object creation is avoided.
While there are alternative design patterns available, the Singleton pattern remains a popular and effective choice in many scenarios.
It’s important, however, to follow best practices when implementing the Singleton pattern. For example, lazy instantiation and thread-safety considerations should be taken into account.
By understanding and properly implementing the Singleton pattern, developers can create efficient and effective software solutions. So, keep the Singleton pattern in mind for your future projects and take your object-oriented programming to the next level.
FAQ
Q: What is the Singleton pattern?
A: The Singleton pattern is a design pattern in object-oriented programming that ensures the creation of only one instance of a class. This pattern is commonly used for classes that should have only one object instance throughout the entire runtime of an application.
Q: Why is the Singleton pattern important in software development?
A: The Singleton pattern provides a way to control object creation, ensuring that only one instance exists. This can be useful for scenarios where having multiple instances of a class could cause issues or conflicts.
Q: How is the Singleton pattern implemented?
A: The Singleton pattern is typically implemented by creating a private constructor for the class, preventing direct instantiation. The class then provides a static method that returns the instance of the class, creating it if it doesn’t exist yet.
Q: What are the benefits of using the Singleton pattern?
A: The Singleton pattern can help improve performance by avoiding unnecessary object creation. It also provides a centralized point of access for the instance of the class, making it easy to manage and control.
Q: Are there alternatives to the Singleton pattern?
A: Yes, there are alternative design patterns such as the Factory pattern or Dependency Injection that can be used instead of the Singleton pattern depending on the specific requirements of the application.
Q: What are some best practices for using the Singleton pattern?
A: Some best practices for using the Singleton pattern include making the class thread-safe, avoiding unnecessary use of the pattern, and ensuring proper cleanup and disposal of resources when necessary.