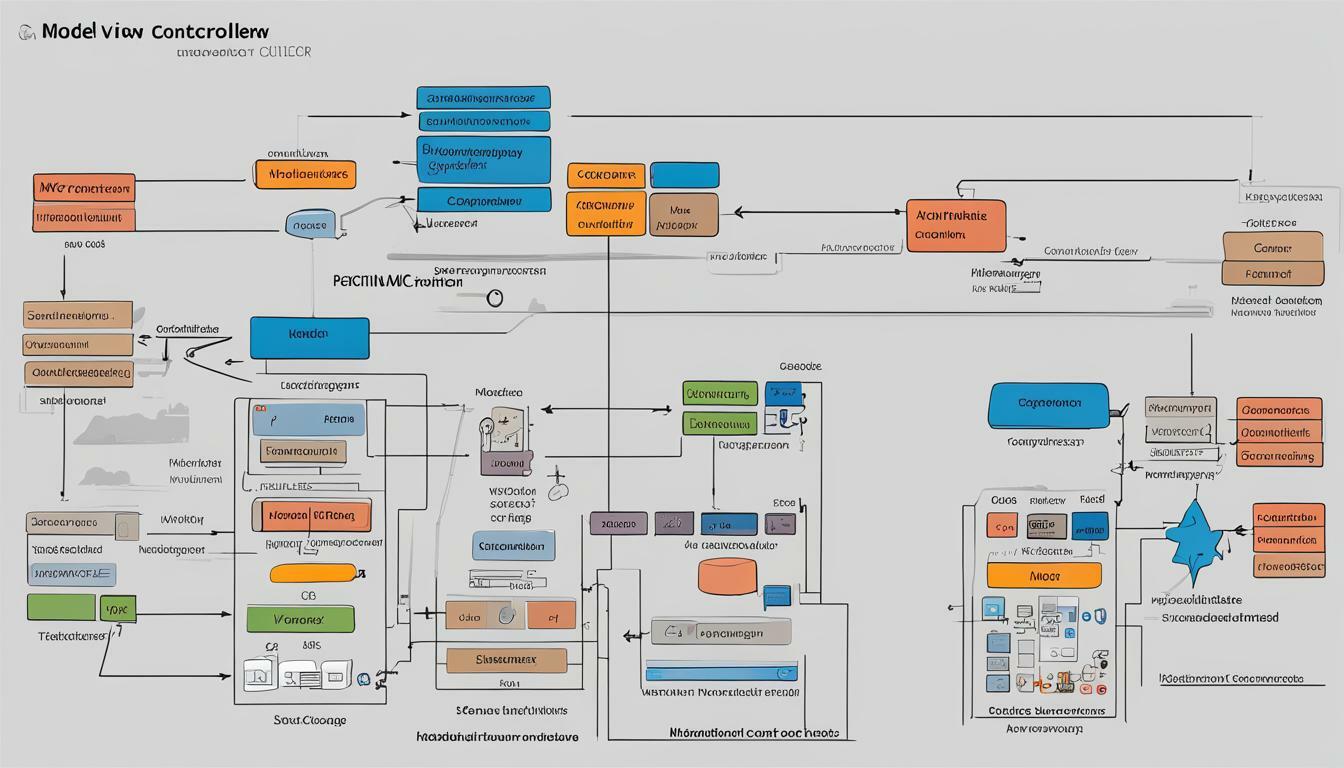
Welcome to our comprehensive guide to Model-View-Controller (MVC)! As more and more software developers turn to this popular framework, it’s essential to have a solid understanding of what it is, how it works, and its benefits and drawbacks. In this article, we’ll take a deep dive into the MVC pattern, explore its architecture and code structure, and provide best practices for implementation. We’ll also address common misconceptions and offer real-world examples of how MVC is used in various software applications.
Key Takeaways:
- Model-View-Controller (MVC) is a widely used framework in software development
- MVC architecture separates an application into three primary components – model, view, and controller
- Implementing MVC can provide benefits such as code reusability, modularity, and improved maintainability
- Potential drawbacks of MVC include increased complexity, potential performance overhead, and potential difficulties in testing
- Understanding and implementing MVC best practices is crucial for successful development
What is Model-View-Controller (MVC)?
Model-View-Controller, commonly referred to as MVC, is a framework used in software development for building and organizing code. It is a design pattern that separates an application into three interconnected components: the model, view, and controller.
The MVC architecture is based on the concept of “separation of concerns,” which means that each component is responsible for a specific part of the application’s functionality. This allows for increased modularity and easier maintenance of the codebase.
The model component represents the data and logic of the application. It interacts with the database and provides the necessary information to the view component. The view component is responsible for presenting the data to the user in an understandable format. It interacts with the model to display the necessary information. Finally, the controller component acts as an intermediary between the model and view, accepting user input and handling interactions between the two components.
The MVC pattern is widely used in modern software development due to its clear separation of concerns and ability to scale to complex applications. The framework provides a clear code structure and allows for better collaboration between developers.
Understanding the MVC Architecture
Model-View-Controller (MVC) is an architectural pattern used in software engineering to separate an application’s data, user interface, and control logic into distinct components. The MVC architecture is designed to address the need for a flexible and modular approach to software development.
The MVC pattern consists of three main components:
- The Model: Represents the data and its related logic. It encapsulates the data access, validation, and business logic components of the application.
- The View: Displays the data to the user and handles user input. The view is responsible for presenting the data in a way that is meaningful and intuitive to the user.
- The Controller: Acts as an intermediary between the model and the view. It handles user input and initiates changes to the state of the model. The controller also updates the view in response to changes in the model.
These three components work together to form the backbone of the MVC pattern. The model is responsible for managing the data, the view is responsible for displaying the data, and the controller is responsible for managing the interaction between the two.
The MVC pattern is a popular design pattern in software engineering due to its flexibility and modularity. It allows developers to work on different components of an application independently, making it easier to maintain and update the code. The MVC architecture can be applied to a wide range of software applications, from small desktop applications to large-scale enterprise systems.
Breaking Down the MVC Components
The Model-View-Controller (MVC) pattern separates an application into three interconnected components: the model, view, and controller. Each component plays a specific role in the overall structure of the application.
The Model
The model represents the core of the application. It contains the data, business logic, and rules required to operate the application. Whenever the state of the data changes, the model updates the view so that the user interface reflects the current state of the application. The model is also responsible for validating user input before making any changes to the data.
The View
The view is responsible for presenting the data to the user. It displays the current state of the application, receives user input, and sends that input to the controller for processing. The view does not contain any business logic or data processing code; instead, it relies on the model and controller to perform those tasks.
The Controller
The controller acts as the intermediary between the model and view components. It receives user input from the view, processes that input using the appropriate business logic from the model, and updates the view accordingly. The controller also handles any communication between the model and view components.
The code structure in an MVC application is organized according to these three components. The model, view, and controller each have their own folders and files, and all communication between components is done through well-defined interfaces. This structure makes it easy to maintain and extend the application over time.
Best Practices for Implementing MVC
When implementing Model-View-Controller (MVC) in software development, it’s important to follow best practices to ensure the code is organized, easy to maintain, and scalable. Here are some key MVC best practices to keep in mind:
- Separation of concerns: Keep each component focused on its specific task, and avoid mixing functionality between them. The model should handle data storage and retrieval, the view should handle the presentation layer, and the controller should handle user input and mediate communication between the model and view.
- Modularity: Break down code into modular components that can be easily reused and tested. Components should be designed to work independently, with clearly defined interfaces.
- Dependency management: Avoid tight coupling between components by using a dependency injection framework or other techniques to manage dependencies. This ensures that changes to one component don’t have unintended consequences on others.
- Use of design patterns: Implementing common design patterns such as factory, builder, or observer can help simplify code and make it easier to maintain and scale.
- Testing: Write unit tests for each component to ensure that it’s working as expected. Use integration tests to verify that all components are properly integrated and working together.
Following these best practices can help ensure that your MVC code is well-organized, modular, and easy to maintain. By keeping a focus on separation of concerns and modularity, you can ensure that changes to one component won’t have unintended consequences on others. Proper dependency management and use of design patterns can help simplify code and reduce complexity. Finally, testing is critical to ensuring that your code is working as expected and that any issues are caught before they become problems.
Advantages of Model-View-Controller (MVC)
Implementing Model-View-Controller (MVC) in software development offers a range of advantages that make it a popular framework for many developers today.
Code reusability: The MVC pattern promotes code reusability, allowing developers to reuse code modules and eliminate redundant code. This not only saves time but also simplifies the development process.
Modularity: The MVC architecture enhances modularity by separating the code into individual components. This makes the code easier to understand, test, and maintain.
Improved maintainability: The separation of concerns achieved through MVC makes it easier to maintain the codebase. It also makes it easier to change one component of the code without affecting the others.
Better collaboration: The separation of concerns facilitated by MVC also enables teams of developers to work more efficiently. With each team member responsible for one component of the code, collaboration becomes easier, and the progress of the team is enhanced.
Flexibility: The MVC pattern can be adapted to suit different software projects and development needs, making it a flexible framework for developers.
Disadvantages of Model-View-Controller (MVC)
While Model-View-Controller (MVC) has many advantages, it also has some potential drawbacks that developers should keep in mind.
Increased complexity: One of the main challenges of using MVC is that it can add complexity to a project. The separation of concerns between the model, view, and controller components requires careful planning and implementation to ensure that the code remains organized and maintainable.
Potential performance overhead: Because MVC requires additional code to manage the relationships between the components, it can also introduce a performance overhead. This is especially true if the code is not optimized for efficiency, which can cause delays or other issues.
Difficulties in testing: Another potential issue with MVC is that it can make testing more difficult. With multiple components interacting with each other, it may be challenging to isolate individual modules for testing purposes. This means that developers must pay close attention to testing and quality assurance to ensure that their code is working as intended.
Despite these challenges, many developers still find value in using Model-View-Controller (MVC) because of its many benefits. However, it is important to understand the potential disadvantages and plan accordingly to ensure that the code remains organized, efficient, and maintainable.
Common Misconceptions about MVC
Model-View-Controller (MVC) is a widely used, proven design pattern that offers numerous benefits in software development. However, there are some misconceptions that surround it. Understanding these misconceptions can help developers make informed decisions when implementing the MVC framework.
Misconception #1: MVC is a framework
While MVC is often referred to as a framework, it is actually a design pattern. A design pattern is a reusable solution to a common problem. In contrast, a framework is a specific implementation of a design pattern that provides additional functionality and support.
Misconception #2: MVC causes unnecessary complexity
Some developers consider the MVC pattern to be overly complex. However, when implemented correctly, MVC can actually simplify the development process by separating concerns and improving code organization. It may require a bit more effort upfront, but the long-term benefits are worth it.
Misconception #3: MVC is only for large-scale projects
While MVC is commonly associated with large-scale projects, it can be used in any software application, regardless of size. In fact, smaller projects may benefit even more from the organization and structure provided by MVC.
Misconception #4: MVC is only for web development
MVC is often associated with web development, but it can be applied to any type of software development project. MVC’s separation of concerns and clear code structure make it an excellent choice for desktop, mobile, and other applications.
Misconception #5: MVC is a one-size-fits-all solution
While MVC can benefit many software development projects, it is not a one-size-fits-all solution. Each project has unique requirements that may require a different approach. Developers should always evaluate the specific needs of their project before deciding to use MVC.
By understanding and debunking these common misconceptions, developers can make informed choices when implementing the Model-View-Controller (MVC) pattern. While it may not be suitable for every project, MVC is a widely-used and proven design pattern with many benefits for modern software development.
Applying MVC: Real-World Examples
Model-View-Controller (MVC) is a versatile framework that can be applied across various software applications. Let’s explore some real-world examples of how MVC is used.
Example 1: Web Applications
In web development, MVC is commonly used to create dynamic and interactive user interfaces. The model represents the data storage and retrieval logic, the view is responsible for rendering the data to the user, and the controller handles user input and updates the model and view accordingly.
For instance, popular web applications such as Facebook, Twitter, and LinkedIn all utilize MVC architecture to manage the complexity of their websites.
Example 2: Mobile Applications
MVC is also applicable in the realm of mobile app development. Here, the model component handles the app’s data and logic, the view component manages the user interface, and the controller component handles user input and updates the view and model accordingly.
As a concrete example, consider the popular mobile game Candy Crush Saga. The game mechanics and data are handled by the model, the visuals and animations are handled by the view, and the controller manages the user’s interactions with the app.
Example 3: Desktop Applications
MVC can also be used in desktop application development. In this case, the model component would manage the application’s data and logic, the view component would handle user interface rendering, and the controller component would manage user input and update the view and model accordingly.
For instance, popular desktop applications such as Adobe Photoshop and Microsoft Excel utilize MVC architecture to create complex yet user-friendly software.
Application | MVC Component |
---|---|
Web Application | |
Candy Crush Saga | Mobile Application |
Adobe Photoshop | Desktop Application |
As you can see, Model-View-Controller (MVC) architecture can be applied in different contexts, making it a valuable framework for software development. By separating concerns and organizing code into distinct components, MVC promotes maintainability, modularity, and reusability.
Conclusion
Understanding Model-View-Controller (MVC) is an essential aspect of modern software development. It provides a structured approach to designing and organizing code, allowing for greater flexibility, maintainability, and scalability. In this article, we have explored the key components and principles of MVC, as well as its advantages and disadvantages.
By implementing MVC, developers can ensure that their code is modular and organized, with clear separation of concerns. This can lead to improved code reusability, easier maintenance, and better collaboration among team members. Additionally, following best practices for implementing MVC can help to avoid potential pitfalls and complexities.
One of the most significant advantages of using MVC is its versatility. It can be applied in a variety of software development contexts, from web applications to desktop applications and beyond. Real-world examples of MVC have demonstrated its practicality and effectiveness in various scenarios.
While MVC may not be suitable for every project, it remains a popular and widely-used framework in the software development industry. By understanding its key principles and best practices, developers can leverage the benefits of MVC to create powerful and efficient applications.
So, what’s next?
Now that you have a fundamental understanding of Model-View-Controller (MVC), it’s time to put it into practice. Whether you are building a new project or refactoring an existing one, MVC can help you to create more efficient and maintainable code. Don’t be afraid to experiment with different approaches and best practices to find what works best for your specific project.
Thanks for reading, and happy coding!
FAQ
Q: What is Model-View-Controller (MVC)?
A: Model-View-Controller (MVC) is a software design pattern that separates the application logic into three interconnected components: the model, view, and controller. The model represents the data and business logic, the view is responsible for the user interface, and the controller manages the interaction between the model and view.
Q: How does the MVC architecture work?
A: In the MVC architecture, the model component handles the data storage and manipulation, the view component handles the presentation of the data to the user, and the controller component manages the flow of data between the model and view. By separating these responsibilities, MVC promotes code reusability, modularity, and maintainability.
Q: What are the advantages of using MVC?
A: Some advantages of using MVC include improved code organization, easier maintenance and updates, better separation of concerns, and enhanced code reusability. MVC also allows for parallel development by enabling different teams to work on different components simultaneously.
Q: What are the disadvantages of using MVC?
A: While MVC offers many benefits, it can introduce added complexity, especially for smaller projects. Additionally, the use of MVC may result in potential performance overhead due to the interactions between components. Testing can also become more challenging when using MVC.
Q: Are there any best practices for implementing MVC?
A: Yes, there are several best practices for implementing MVC. These include organizing code into separate directories for each component, minimizing dependencies between components, and ensuring that each component has a clear and specific role within the architecture. It is also important to maintain separation of concerns and follow coding conventions and standards.
Q: What are some misconceptions about MVC?
A: Common misconceptions about MVC include thinking that it is a specific framework or that it can only be used for web development. In reality, MVC is a design pattern that can be applied to various programming languages and platforms. It is a flexible and widely used architectural pattern in software development.
Q: Can you provide real-world examples of MVC?
A: MVC is used in a wide range of software applications. Some examples include web frameworks like Ruby on Rails and Laravel, desktop applications built with Java Swing, and mobile apps developed using frameworks such as React Native. These examples demonstrate the versatility and effectiveness of MVC in different contexts.