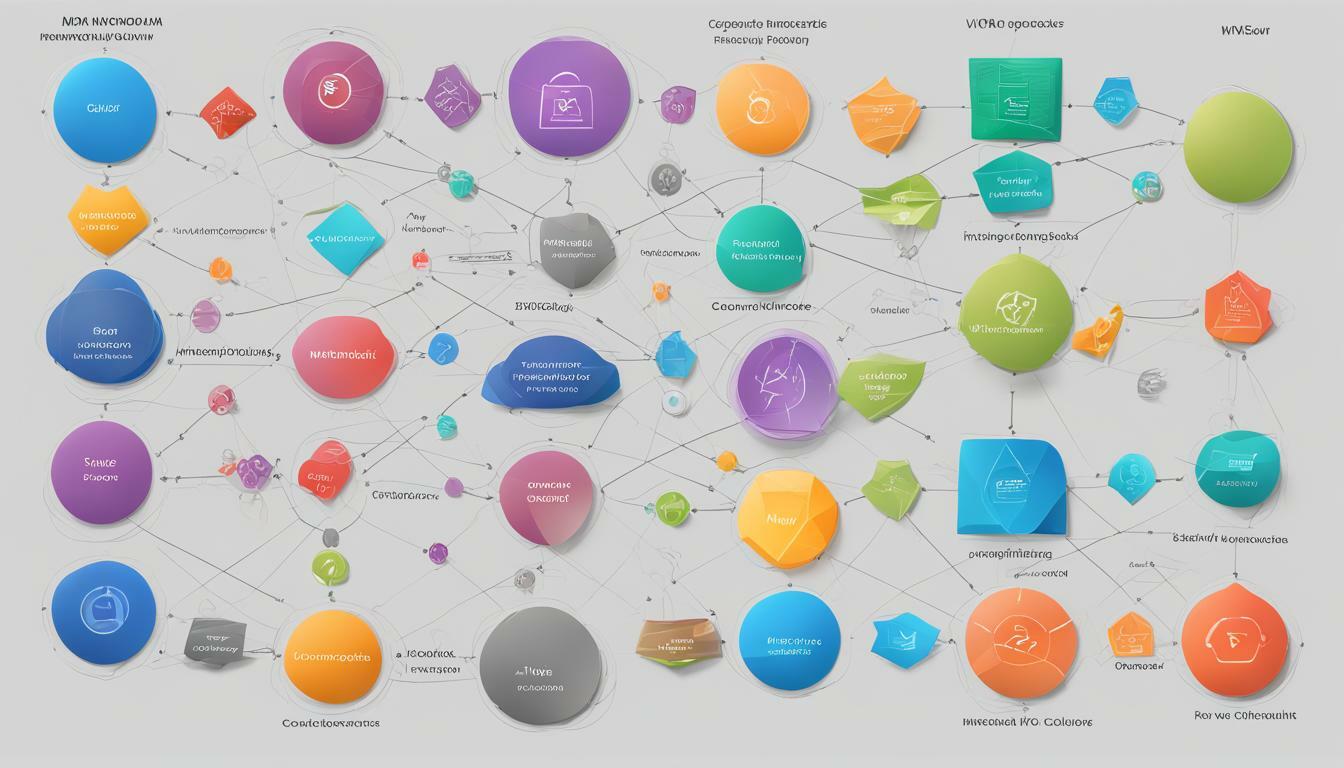
As a software developer, it’s essential to have a sound understanding of the various architectural patterns and design principles used in software development. One such architecture that has gained popularity in recent years is the Model-View-ViewModel (MVVM) pattern. The MVVM pattern is a software architecture that separates the model, view, and view model components, making development more organized and efficient.
In this comprehensive guide, we will provide a detailed overview of the MVVM pattern, its architecture, design principles, and benefits. We’ll also explore how to implement and use the MVVM framework in real-world applications, including some best practices for MVVM development.
Key Takeaways:
- The MVVM pattern is a software architecture that separates the model, view, and view model components.
- Understanding the MVVM pattern is essential for efficient, organized software development.
- In this comprehensive guide, we will provide an overview of the MVVM pattern, its architecture, and benefits. We’ll also cover implementation, best practices, and real-world applications of MVVM.
What is MVVM?
The Model-View-ViewModel (MVVM) pattern is a software architecture that focuses on separating the presentation layer (the View) from the business logic (the Model) by introducing an intermediary component called the ViewModel. The ViewModel is responsible for bridging the gap between the View and the Model, thus forming the core of the MVVM pattern.
MVVM is an extension of the Model-View-Controller (MVC) pattern but replaces the Controller with the ViewModel. It addresses the challenges with traditional MVC by providing a more clear separation of concerns and a better-defined structure for the presentation layer.
Understanding the Core Concepts of MVVM
In MVVM, the Model represents the data and logic of the application. It includes the data that the application works with and the operations that can be performed on that data.
The View is the visual representation of the data and logic provided by the Model. It includes the user interface and the various controls used to interact with the application.
The ViewModel is the intermediary component that connects the View and the Model. It exposes the data and operations provided by the Model to the View in a way that is easily consumable.
How MVVM Works
The ViewModel communicates with the Model by retrieving and updating data through data binding. Data binding is a programming technique that connects the properties of two objects together, allowing them to stay in sync.
The ViewModel also communicates with the View through data binding. This allows the ViewModel to pass data to the View and receive user input from the View.
One of the key advantages of MVVM is that it allows for the separation of concerns between the different components. This improves code maintainability, testability, and scalability, making it an ideal option for large projects.
Overall, MVVM is a powerful pattern that provides a structured and efficient way to design and develop complex software applications. Understanding its core concepts is essential for any developer looking to improve their software architecture skills.
Advantages of MVVM
Adopting the Model-View-ViewModel (MVVM) pattern in software development can provide numerous benefits and advantages that improve the overall development process.
Improved Code Maintainability: MVVM promotes the separation of concerns between the model, view, and view model, making it easier to maintain and update code. This allows developers to modify code quickly and efficiently, without causing disruptions in other areas of the software.
Enhanced Testability: By separating the logic from the UI, MVVM enables easier automated testing of the code. This means that developers can ensure that the code is working as expected and meeting all functional requirements before deploying it to the end-users.
Increased Reusability: The MVVM pattern encourages the creation of reusable components, making it easier to update software and increase overall efficiency. This reduces the time and effort spent on duplicating code, ultimately resulting in quicker development time and cost savings.
Efficient Development Process: MVVM provides a clear separation of responsibilities between the model, view, and view model, which makes it easier to develop, test, and maintain software. This results in a more organized and efficient development process, leading to better quality software and increased productivity.
Implementing MVVM
Implementing the Model-View-ViewModel (MVVM) pattern involves incorporating specific components that enable data binding, commands, and dependency injection. In this MVVM tutorial, we will go through the step-by-step process of implementing MVVM in software projects.
Data Binding
One of the core principles of MVVM is data binding, where the view and view model are connected through data properties. To implement data binding, use the binding expression syntax to bind properties in the view to properties in the view model. This allows for automatic updates of the view when the view model data changes, and vice versa.
Commands
Commands in MVVM are used to bind user actions in the view to methods in the view model. To implement commands, create a command object in the view model and bind it to a control in the view, such as a button. This enables the execution of specific tasks in the view model when the user interacts with the control in the view.
Dependency Injection
Dependency injection is used in MVVM to provide a separation of concerns between components. To implement dependency injection, register dependencies in the application container and inject them into the view model constructor. This allows the view model to have access to services and dependencies without having to create or manage them.
Practical Examples
Let’s take a look at some practical examples of implementation of MVVM. In a mobile app, the view may consist of an activity or fragment, the view model would contain the business logic and data binding, and the model would be responsible for data storage. In desktop software, the view may be a window or dialog, the view model would contain the logic for handling user input and events, and the model would interact with the database or storage system.
In conclusion, implementing MVVM requires a clear understanding of the necessary components, such as data binding, commands, and dependency injection. As demonstrated in this MVVM tutorial, by implementing these components, developers can take advantage of the benefits of the MVVM architecture, such as improved code maintainability and testability.
MVVM Best Practices
Implementing the MVVM pattern in software development requires adherence to certain best practices to maximize its benefits. Here are some essential guidelines to follow:
- Naming conventions: Use clear and concise names for classes, properties, and methods. Follow naming conventions that reflect the purpose and context of each component.
- View model design: Keep view models simple and focused on their specific responsibilities. Avoid cluttering them with complex logic or excessive dependencies.
- Handling navigation: Use a dedicated navigation service to decouple view models from view navigation. This promotes better code maintainability and testability.
- Data validation: Implement data validation on both the model and view model levels to ensure data integrity and consistency.
- Unit testing: Test each component of the MVVM pattern separately to ensure robust code coverage. Use a reliable unit testing framework to automate testing and reduce the risk of bugs.
By following these best practices, developers can optimize their use of the MVVM pattern and achieve better software development outcomes.
MVVM in Real-world Applications
Model-View-ViewModel (MVVM) has been widely adopted in real-world software applications due to its versatility and scalability. Let’s explore some examples of where MVVM has been successfully implemented.
Mobile App Development
MVVM is a popular choice for developing mobile apps. It provides a clear separation between the user interface and the underlying business logic, making it easier to maintain and test applications. In addition, MVVM’s data binding capabilities are particularly well-suited for mobile development, where screen sizes and orientations can vary widely.
For example, the popular mobile app Tinder uses the MVVM architecture to achieve a sleek and responsive interface that provides a smooth user experience.
Web Applications
Web applications also benefit from the structure and organization provided by MVVM. The separation of concerns between the view, model, and view model makes it easier to isolate and debug issues. The ability to bind data directly to the view eliminates the need for repetitive code and reduces development time.
One successful implementation of MVVM in web applications is the online marketplace Etsy. The platform uses MVVM to manage complex user interfaces while maintaining the performance and scalability needed to handle a large number of simultaneous users.
Desktop Software
The MVVM pattern is not limited to mobile and web applications. It is also a popular choice for desktop software development, particularly with the rise of cross-platform development frameworks like Xamarin and .NET Core. These frameworks enable developers to apply MVVM principles to desktop applications, creating software that is flexible, maintainable, and easy to scale.
For example, the popular graphic design software Adobe Photoshop uses MVVM to create a smooth and intuitive user experience, while still providing flexibility for complex operations and customization.
MVVM vs. Other Architectural Patterns
When it comes to software development, there are several popular architectural patterns, including Model-View-Controller (MVC), Model-View-Presenter (MVP), and of course, Model-View-ViewModel (MVVM). Each pattern has its own strengths and weaknesses, and selecting the appropriate one for a particular project requires careful consideration of various factors.
MVC vs. MVVM
MVC is one of the most widely used architectural patterns in software development, particularly for web applications. In MVC, the controller acts as an intermediary between the view and the model, whereas in MVVM, the view model handles the communication between the view and the model. MVVM separates the view’s state and behavior from its layout and appearance, making it easier to test and maintain the codebase.
Another significant difference between MVC and MVVM is the level of independence between components. In MVC, the view and the model are more tightly coupled, whereas in MVVM, the view model acts as a mediator, allowing for better separation of concerns.
MVP vs. MVVM
MVP is another architectural pattern that separates the concerns of the user interface and the business logic. In MVP, the presenter acts as an intermediary between the view and the model, just like in MVC. However, in MVP, the presenter handles the user input and updates the view. In contrast, in MVVM, the view model exposes properties and commands that the view can bind to, making it more flexible and easier to test.
One of the main benefits of MVVM over MVP is the reduced coupling between the view and the view model. This separation of concerns results in improved flexibility and maintainability of the codebase over time.
Choosing the Right Pattern
Ultimately, the choice between these architectural patterns comes down to the specific needs and requirements of the project. For instance, if the project involves web development, MVC might be the most appropriate choice. On the other hand, if the project involves mobile app development, MVVM might be a better fit due to its flexibility and modularity.
It’s also important to consider the skill level of the development team and the available resources when selecting an architectural pattern. Teams with more experience in one pattern may find it easier to implement and maintain, while the availability of existing frameworks and tools can also influence the decision.
In conclusion, understanding the similarities and differences between MVVM and other architectural patterns can help developers make informed decisions when selecting the most appropriate pattern for their projects.
Overcoming Challenges in MVVM Development
While the MVVM pattern offers numerous benefits, developers may face some challenges when implementing it in software projects. Here are some common challenges and strategies to overcome them:
Handling Complex Data Bindings
One of the challenges in MVVM development is handling complex data bindings between the view and view model. This can be especially difficult in large-scale projects with multiple components.
A solution to this challenge is to break down the bindings into smaller, manageable pieces. This can be achieved by creating separate view models for each component and using a mediator or event aggregator to handle communication between them. The use of dependency injection can also simplify data binding by reducing the need for manual binding code.
Managing View Model State
Another challenge in MVVM development is managing the state of view models. This involves ensuring that view models are updated with the latest data and that changes are propagated to the view in a timely manner.
A solution to this challenge is to use a framework that supports automatic state management, such as React or Angular. These frameworks can handle the state updates and ensure that view models and views remain in sync. Alternatively, developers can implement their own state management system using observable properties and event handlers.
Dealing with Communication Between Components
Communication between components can be a challenge in MVVM development, especially when different components have different dependencies and requirements.
A solution to this challenge is to use a mediator or event aggregator to handle communication between components. This allows components to remain loosely coupled and reduces the chances of dependencies between components. The use of dependency injection can also simplify communication between components by providing a standardized interface for communication.
By following these strategies, developers can overcome common challenges in MVVM development and ensure successful implementation of the MVVM pattern in their software projects.
MVVM in the Future of Software Development
The MVVM architecture has already shown its potential advantages in terms of code maintainability, testability, and reusability. As software development continues to evolve, there are several emerging trends and technologies that could enhance the MVVM pattern.
One promising development is the growing popularity of reactive programming, which allows for responsive and efficient UI updates. Reactive programming involves the use of observable sequences, where data changes trigger events that propagate throughout the application. This methodology aligns well with the MVVM pattern, as the view model can serve as the intermediary between observables and the view.
Another trend that could impact MVVM development is the increasing use of functional programming languages and concepts. Functional programming emphasizes the use of immutable data and pure functions, which can simplify the design and testing of software applications. MVVM can benefit from functional programming by promoting a clear separation of concerns between the model, view, and view model.
Overall, the MVVM architecture is well-positioned to adapt and evolve with the changing landscape of software development. As new technologies and methodologies emerge, MVVM will likely continue to provide a solid foundation for building robust, scalable software applications.
MVVM Case Studies
Implementing the MVVM architecture has resulted in successful projects across various industries. Let’s take a look at a few case studies:
Case Study 1: Mobile Banking Application
Challenge | Solution | Outcome |
---|---|---|
The development team faced challenges in keeping the user interface responsive while retrieving data from the server. | Implementing the MVVM pattern helped the team separate the UI logic from the data retrieval logic. The ViewModel was responsible for handling data requests, while the View displayed the data. The data was retrieved asynchronously, ensuring the UI remained responsive. | The mobile banking application was rated highly by users for its responsiveness and was able to handle a large user base. |
Case Study 2: E-commerce Website
Challenge | Solution | Outcome |
---|---|---|
The development team faced challenges in maintaining the website’s performance while handling a large number of product listings. | Implementing the MVVM pattern helped the team separate the concerns of the Views and ViewModels. The ViewModel handled the business logic, and the View was responsible for displaying the data. The data binding feature helped to update the View automatically when the ViewModel changed, and caching was used to improve the application’s performance. | The website’s load time was significantly reduced, making it faster and more efficient, resulting in increased user engagement and sales. |
Case Study 3: Healthcare Management System
Challenge | Solution | Outcome |
---|---|---|
The development team struggled to manage the complex data associated with patients’ medical records. | Implementing the MVVM pattern allowed the team to separate the data and logic concerns. The Model represented the data structure, the ViewModel handled the data processing and communication with the Model, and the View displayed the data. The team used data binding to automatically update the View when the ViewModel changed, and the Command feature helped in processing user input. | The healthcare management system was successfully implemented, and the data was managed efficiently, resulting in improved patient care. |
The above case studies demonstrate that the MVVM architecture can be applied to projects across various industries and can help teams overcome development challenges. It provides better code maintainability, testability, and reusability, making it a valuable architecture pattern for software development.
Conclusion
The Model-View-ViewModel (MVVM) pattern is an essential aspect of software development that enables developers to build maintainable, testable, and scalable applications. Throughout this comprehensive guide, we have explored the core concepts and principles of MVVM, its advantages, implementation strategies, best practices, and real-world applications.
It is clear from our discussion that MVVM is versatile and can be used in a wide range of contexts, including mobile app development, web applications, and desktop software. We have also seen how MVVM compares to other popular architectural patterns and the potential impact it may have on future software development practices.
Undoubtedly, MVVM presents some challenges for developers, but our guide has provided solutions and strategies to overcome these challenges. We have also presented case studies of successful projects that have implemented the MVVM pattern, demonstrating its effectiveness in real-world scenarios.
In conclusion, understanding and implementing the MVVM pattern is crucial for building high-quality and maintainable software. As software development continues to evolve, MVVM is expected to play an increasingly important role in shaping the future of application development.
FAQ
Q: What is the Model-View-ViewModel (MVVM) pattern?
A: The MVVM pattern is a software architecture that separates the user interface (view) from the business logic (model) and uses a mediator component called the view model to communicate between the two. This separation allows for better code organization and testability.
Q: What are the advantages of using MVVM?
A: MVVM offers several benefits, including improved code maintainability, testability, and reusability. It promotes a more efficient development process by separating concerns and providing a clear structure for the UI and business logic.
Q: How do I implement MVVM in my software project?
A: To implement MVVM, you need to separate your UI into views, which are responsible for displaying the data, and view models, which handle the business logic and expose the data to the views. You can use data binding and commands to connect the view and view model, and dependency injection to provide dependencies to the view model.
Q: What are some best practices for working with MVVM?
A: When working with MVVM, it’s important to follow naming conventions, properly design your view models, and handle navigation between views. Additionally, it’s recommended to use a design pattern like the repository pattern for data access and consider using a framework that supports MVVM.
Q: How is MVVM different from other architectural patterns?
A: MVVM differs from other architectural patterns, such as MVC and MVP, in how it separates the view and the view model. In MVVM, the view and view model have a one-to-one relationship, while in MVC and MVP, the view interacts directly with the controller or presenter.
Q: What are some common challenges in MVVM development?
A: Some common challenges in MVVM development include managing complex data bindings, handling view model state, and facilitating communication between components. These challenges can be overcome by using proper design patterns, utilizing frameworks that support MVVM, and following best practices.
Q: How is the MVVM pattern expected to evolve in the future?
A: The MVVM pattern is expected to evolve alongside emerging trends and technologies in software development. It may incorporate advancements in UI frameworks, data binding libraries, and state management techniques to provide even more efficient and robust solutions.
Q: Can you provide case studies of successful MVVM implementations?
A: Yes, there are several successful projects that have implemented the MVVM pattern. These case studies showcase the benefits and effectiveness of MVVM in various contexts, such as mobile app development, web applications, and desktop software.