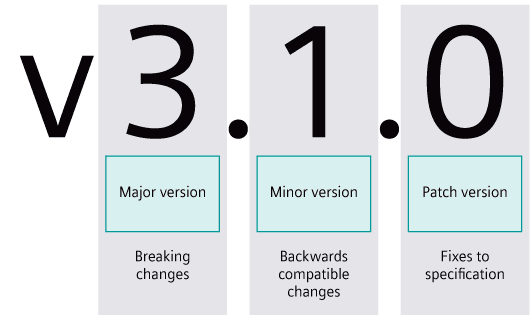
Developers know how key it is to keep and grow our APIs. A good API versioning strategy is vital. It makes sure our .NET Web APIs stay scalable, easy to handle, and simple to grasp.
With a solid API versioning plan, we can handle changes smoothly. This cuts down on service disruptions. It lets developers work better with our APIs, creating a stronger and more dependable system.
We aim to give a detailed, easy-to-follow guide on API versioning in .NET Web API. This will help developers of all levels. Our goal is to share deep, clear programming knowledge with the tech world.
Understanding API Versioning: Why It Matters
API versioning is key for .NET Web API developers. It affects how well the API works and grows. Changes in the API can cause problems if not managed right.
API versioning helps keep the API strong and ready to change. This is important in today’s fast world, where being quick and reliable matters a lot.
The Importance of Version Control
Version control is central to API versioning. It helps track changes and keep different API versions working together. This way, we can make sure updates don’t mess up what’s already working.
Good version control also lets us go back to old versions if needed. This is crucial in live environments, where keeping things running smoothly is essential.
Versioning Benefit | Description | Impact on Clients |
---|---|---|
Backward Compatibility | Ensures that new versions of the API do not break existing integrations. | Minimal disruption; clients can continue using the API without significant changes. |
Change Management | Allows for the tracking and management of changes across different API versions. | Clients can choose when to upgrade to a new version, based on their own development schedules. |
Rollback Capability | Enables the rollback of changes if issues arise with a new API version. | Reduces risk for clients, as problematic versions can be quickly addressed. |
Common Challenges Without Versioning
Without a good API versioning plan, developers face many problems. These include issues with compatibility, trouble rolling back changes, and unclear API updates. This can upset clients and hurt trust in the API.
Some big challenges are:
- Handling many, unversioned API endpoints gets very hard.
- Clients might face problems because of sudden or wrong changes.
- Without a clear versioning plan, adding new API features is harder.
Knowing why API versioning is important helps us see why we need a solid plan for our .NET Web APIs. Next, we’ll look at the main ideas and ways to do API versioning well.
Key Principles of API Versioning in .NET
When we set up API versioning in .NET Web API, we need to follow some key rules. These rules help keep our API system strong and easy to update. It’s important to manage changes well so they don’t mess up what’s already working.
Consistency in API Design
Good API design is all about being consistent, even more so when we’re versioning. Keeping the API structure the same makes it easier for developers to work with. They can use standard HTTP methods, predictable endpoint names, and consistent error handling more smoothly. This helps them get used to new API versions faster.
To keep things consistent, we should:
- Stick to standard naming for endpoints and parameters.
- Keep HTTP status codes and error messages the same.
- Make sure to document all changes and updates clearly.
Backward Compatibility
Backward compatibility is another big deal in API versioning. It lets older API versions keep working right, even as new ones come out. This is key for clients that might not update right away.
To keep things compatible, we can:
- Support many API versions at once.
- Don’t change existing endpoints in ways that break them.
- Use URI or header versioning to tell different versions apart.
By sticking to these rules, we can create a strong API versioning plan in .NET Web API. It meets the need for new features while keeping things stable and reliable.
Different Strategies for API Versioning
The world of API versioning is full of options. Developers can use URI, query string, or header versioning.
When making an API, picking the right versioning strategy is key. It helps keep things working as they should and meets changing client needs. We’ll look at URI, query string, and header versioning.
URI Versioning
URI versioning puts the version number in the API’s URI. It’s simple and clear, making it easy to tell versions apart.
Example: https://api.example.com/v1/users
This method is good because it keeps versions separate and works well with web servers. But, it can make URIs grow a lot.
Query String Versioning
Query string versioning uses a query parameter for the version. It keeps the URI the same for all versions.
Example: https://api.example.com/users?version=1
This is easy to set up and doesn’t change the URI. But, it might not be as clear or RESTful as other ways.
Header Versioning
Header versioning uses a custom HTTP header for the version. It keeps the URI and query string clean and works well for complex scenarios.
Example: Using a header like Accept: application/vnd.example.v1+json
This method is very flexible and doesn’t mess up the URI. But, it’s harder to set up and test, mainly for clients not used to custom headers.
Knowing these strategies helps us pick the best one for our needs. We balance simplicity, flexibility, and what our clients need.
Choosing the Right Versioning Strategy
Choosing the right versioning strategy for your .NET Web API is key. It keeps your API working with old clients and new ones. There are many strategies, each with its own good and bad points.
Before picking a strategy, think about a few important things. These include the changes you’re making, what your clients need, and your API’s design.
Factors to Consider
When looking at versioning strategies, several things matter. These include:
- The frequency of changes to your API
- The diversity of your client base and their needs
- The complexity of your API and its components
- Your organization’s support and maintenance capabilities
Knowing these factors helps you choose the best versioning strategy for your .NET Web API.
Pros and Cons of Each Approach
Now, let’s look at the good and bad of main versioning strategies:
Versioning Strategy | Pros | Cons |
---|---|---|
URI Versioning | Easy to implement, clear separation of versions | Can lead to a large number of URIs, potentially violating the principle of URI opacity |
Query String Versioning | Simple to implement, doesn’t clutter URI space | Can be seen as not RESTful, caching issues |
Header Versioning | Doesn’t clutter URI space, flexible | Can be complex to implement, requires client cooperation |
By considering these points and the pros and cons of each strategy, you can choose wisely. This choice should fit your project’s needs and limits.
Implementing URI Versioning in .NET Web API
URI versioning in .NET Web API is a structured approach to manage API versions. It ensures backward compatibility and clarity. By including the version number in the URI, it’s easy for clients to choose the version they need.
Setting Up Versioned Routes
To set up versioned routes, we modify the routing in our .NET Web API project. We define routes with the version number included. Here’s how:
- Use a route template with the version, like
api/v{version}/{controller}
. - Set up routing for different versions with attribute or conventional routing.
For example, attribute routing can specify the version on controllers or actions:
[ApiVersion("1.0")]
[Route("api/v{version:apiVersion}/[controller]")]
public class MyController : ControllerBase
{
// Actions
}
Handling Multiple Versions
Managing multiple versions is key for a robust API. Here are some tips:
- Version-Specific Controllers: Have separate controllers for each API version. This keeps things organized but might cause duplication.
- Version-Specific Actions: Use different actions in one controller for various versions. This is good for small changes.
- Using a Single Controller with Versioned Services: Use different services for each version in a single controller. This keeps the controller simple and focused on routing.
These strategies help keep our .NET Web API maintainable and flexible for future changes.
Implementing Query String Versioning
Query string versioning is a good way to manage different API versions. It involves adding the version as a query string parameter in the API request.
Modifying Request Parameters
To start with query string versioning, we need to add the version info to the request. This is done by adding a query string parameter, like ?version=1
, to the API URL. For instance, https://api.example.com/users?version=1
tells the server to use version 1.
In .NET Web API, we must change our controllers to handle this version info. We can do this with a custom attribute or by accessing the query string directly in our actions.
Processing Query Strings in .NET
In .NET, we access query string parameters in our controller actions. We use Request.GetQueryNameValuePairs()
to get these parameters and then process them.
Here’s how to handle the version query string in a .NET Web API controller:
public class UsersController : ApiController
{
public IHttpActionResult Get()
{
var queryParams = Request.GetQueryNameValuePairs();
var version = queryParams.FirstOrDefault(kvp => kvp.Key == "version").Value;
if (version == "1")
{
// Return version 1 of the data
}
else if (version == "2")
{
// Return version 2 of the data
}
else
{
return BadRequest("Invalid version");
}
}
}
We can also use a custom attribute for versioning. This involves creating a filter attribute that checks the version and routes the request.
The table below shows the main differences between modifying request parameters and processing query strings in .NET:
Aspect | Modifying Request Parameters | Processing Query Strings in .NET |
---|---|---|
Purpose | Include version information in the request | Retrieve and process version information |
Implementation | Append query string parameter to API endpoint URL | Use Request.GetQueryNameValuePairs() to retrieve query string parameters |
Benefits | Flexible and easy to implement | Allows for dynamic processing of version information |
By following these steps and understanding query string versioning, we can manage API versions in our .NET Web API apps effectively.
Header Versioning: A Deeper Dive
Header versioning is a key method in API versioning. It keeps versioning separate from the URI or query string. This makes the API interface cleaner and more flexible. It uses custom HTTP headers to share version info, making API version management more advanced.
Customizing Headers for Versioning
To use header versioning, developers must add version info to HTTP headers. They can use a header like Accept
or a custom one, like Api-Version
. The server then uses this header to choose the right API version.
For example, a client might send a request with Accept: application/vnd.example.v1+json
. This tells the server to use version 1 of the API. This way, clients can pick their API version without changing the URI or query string.
Advantages of Header Versioning
Header versioning has many benefits:
- Flexibility: It separates versioning from the URI, making it easier to manage different API versions.
- Clean URIs: URIs stay simple because version info is in headers, making the API easier to use.
- Ease of Implementation: If an API already uses content negotiation, adding header versioning is simple.
Here’s a table comparing different versioning methods, including header versioning:
Versioning Strategy | Flexibility | URI Cleanliness | Implementation Ease |
---|---|---|---|
URI Versioning | Low | No | High |
Query String Versioning | Medium | Yes | Medium |
Header Versioning | High | Yes | Medium |
Maintaining Versioned APIs
Keeping APIs up to date is key. It means getting rid of old versions and talking to developers. This is important for the API’s success over time. It keeps current users happy and lets the API grow.
We need to balance old and new versions. This balance helps keep the API working for everyone. It’s all about planning and talking to developers clearly.
Deprecating Older Versions
Getting rid of old API versions is tricky. We must warn users before we do it. This gives them time to switch to a newer version.
To do it well, we should:
- Be clear about when and why we’re getting rid of old versions.
- Help users move to newer versions.
- Let users know when they’re using something that’s going away.
Communication with Developers
Talking to developers is crucial. We must tell them about changes and new stuff. We can use:
- Newsletters or updates.
- Changelogs.
- Forums or community sites.
Being open helps build trust. It makes moving between versions smoother.
Keeping APIs updated is a big job. But with careful planning and good communication, we can make it work. This way, our APIs will keep doing well for a long time.
Best Practices for API Versioning
To keep your .NET Web API working well over time, it’s key to follow best practices for API versioning. A good versioning plan helps keep old services working while adding new ones. This way, you don’t mess up what’s already working.
Documentation and Clarity
Good API versioning starts with clear, detailed documentation. It’s important for developers to know about your API’s versions. They should understand what’s new, what’s old, and how to move between versions.
Key elements of good documentation include:
- Clear version numbering
- Detailed change logs
- Migration guides
- Examples and tutorials
User Feedback and Adaptation
Listening to user feedback is crucial for making your API better. By using feedback to improve your API, you can make users happier. This helps build a strong community around your API.
Strategies for gathering and incorporating user feedback include:
- Conducting surveys and polls
- Monitoring API usage patterns
- Engaging with developers through forums and social media
Let’s look at how different API versioning strategies compare:
Versioning Strategy | Complexity | Flexibility | Maintainability |
---|---|---|---|
URI Versioning | Low | High | Medium |
Query String Versioning | Medium | Medium | High |
Header Versioning | High | Low | Medium |
Testing API Versions Effectively
Testing API versions is a detailed process that needs careful planning and the right tools. We talked about how important versioning is in .NET Web API for managing changes and keeping things backward compatible. Now, we must check how these changes work across different versions.
Tools for API Testing
To test API versions well, we need the right tools. Postman and Swagger are among the most popular for this. They help us create, test, and document our APIs efficiently.
Postman offers a full platform for API testing, with features like automated testing and tools for working together. Swagger provides a strong framework for API documentation and testing. This makes it easier to understand and use our API.
Strategies for Comprehensive Testing
A good testing strategy for API versions includes several steps. First, we must identify the scope of testing. We need to decide which API versions to test and what parts of the API to check.
- Test for backward compatibility to make sure newer versions still work with older functions.
- Check if each version works as expected.
- Do load testing to see if the API can handle expected traffic across versions.
By using these strategies and the right tools, we can keep our .NET Web API stable and reliable across different versions.
Real-world Examples of API Versioning
Let’s look at how top APIs use versioning, with Microsoft Graph API as a key example. By checking out real scenarios, we can learn a lot about making APIs better.
Case Study: Microsoft Graph API
Microsoft Graph API shows how versioning works well. It lets developers pick the API version they want to use. This is done by adding the version to the URL.
The Microsoft Graph API balances old and new features. This keeps a big group of developers happy and working together.
API Version | Release Date | Key Features |
---|---|---|
v1.0 | Initial Release | Core functionality for accessing Microsoft services |
beta | Ongoing | Preview of upcoming features and enhancements |
v2.0 | Future Release | Enhanced security and additional service integrations |
Lessons from Public APIs
Public APIs like Microsoft Graph teach us a lot. One important thing is to have clear documentation and talk to developers about changes.
Another lesson is to have a flexible versioning strategy. This means being ready to change how you version your API as needs shift.
- Plan for backward compatibility to support existing clients.
- Communicate version changes and deprecations clearly to developers.
- Use a flexible versioning strategy that can adapt to changing needs.
By learning from these examples, developers can make APIs that are strong, flexible, and easy for others to use.
Conclusion and Next Steps
Exploring API versioning in .NET Web API shows its importance. A structured approach is key for a strong and growing API. Developers can pick the best strategy for their project by understanding the options.
Key Takeaways
We’ve looked at different ways to version APIs, like URI, query string, and header versioning. Each method has its advantages and disadvantages. The right choice depends on the API’s complexity, who it’s for, and the team’s skills.
Resources for Further Learning
.NET developers can learn more about API versioning from Microsoft’s ASP.NET Core documentation. Also, checking out GitHub projects and developer forums can help. They offer great tips and advice on handling API versions well.