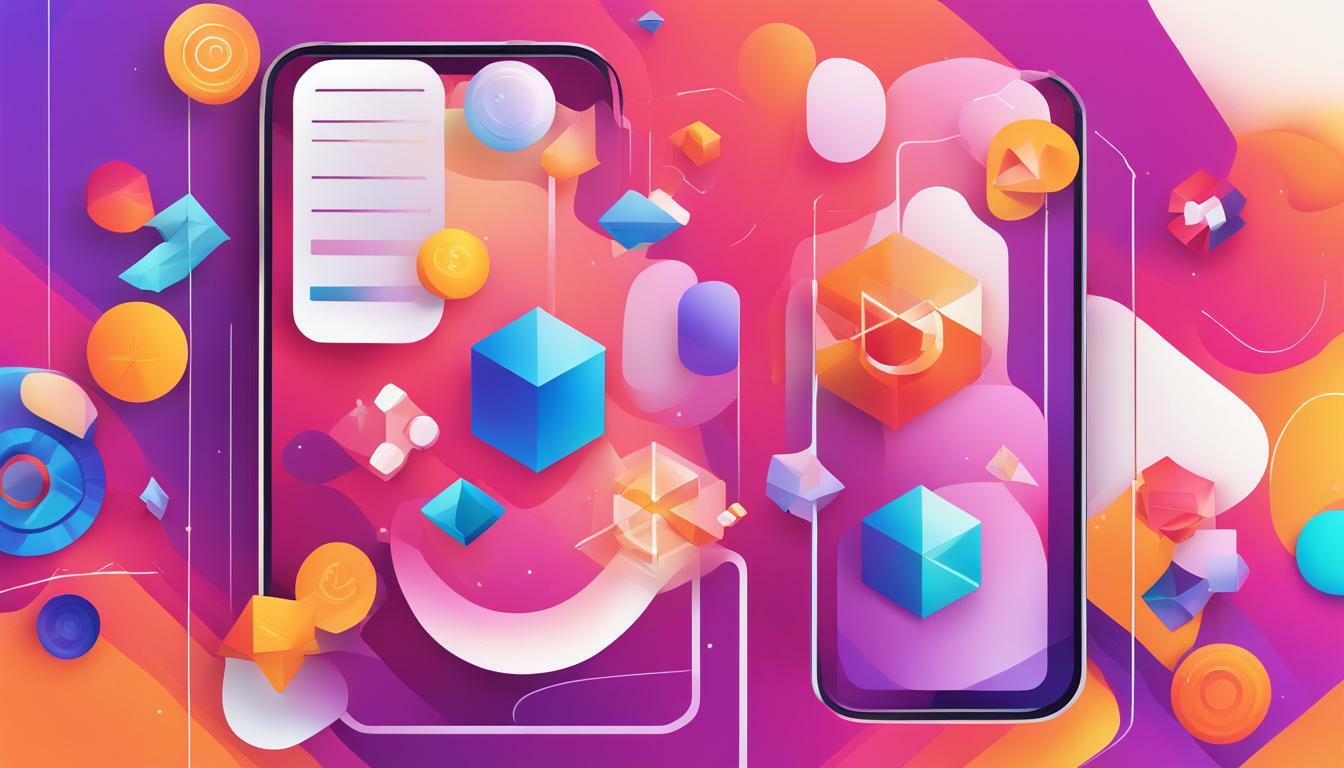
If you’re looking to build high-quality Flutter apps that are both robust and maintainable, look no further than Dart best practices. Dart is the programming language used to develop Flutter, and following best practices when coding can make all the difference in creating efficient and stable applications.
In this section, we will dive into Dart best practices and explore the importance of following these guidelines when building your Flutter apps. By the end of this article, you’ll have a solid understanding of the key benefits of using Dart and the essential best practices to ensure your apps are built the right way.
Understanding Flutter App Development
Flutter app development is becoming increasingly popular due to its ability to create beautiful, high-performance apps for multiple platforms using one codebase. At the core of it is Dart, a programming language developed by Google, which is used to write the logic that powers Flutter apps.
Developing Flutter apps with Dart has several advantages, including:
- Hot-reload: This enables developers to see changes made in the code instantly, making the development process faster and more efficient.
- Expressive and concise syntax: Dart has a clean, easy-to-understand syntax that allows developers to write code that is easy to read and maintain.
- Object-oriented programming: Dart is an object-oriented language, which makes it easier to organize and structure code.
Flutter also allows for easy integration with native features of the device on which the app is running, such as the camera, accelerometer, and GPS, making it a versatile framework for building advanced apps with native-like performance.
Key Dart Best Practices for Flutter App Development
Building a robust and efficient Flutter app requires following Dart best practices for Flutter development. In this section, we will explore the essential coding techniques that every developer should know when building Flutter apps.
Code Organization
Proper code organization is essential for building scalable, maintainable, and reusable code. Here are some helpful tips for organizing your Flutter app’s code:
Technique | Description |
---|---|
Separate concerns | Separate your code into logical layers, such as UI, business logic, data access, and model classes. |
Use meaningful names | Name your classes, methods, and variables using meaningful and descriptive names that clearly indicate their function. |
Keep it simple | Avoid complex class hierarchies or deep nesting of functions, which can make your code hard to understand and maintain. |
Naming Conventions
Consistent naming conventions can make your code more readable and maintainable. Use the following conventions to name your classes, methods, and variables:
- Use camelCase for naming variables and methods.
- Use PascalCase for naming classes.
- Prepend an underscore (_) to name private variables and methods.
Error Handling
Effective error handling is critical for building reliable apps. Here are some tips for handling errors in your Flutter app:
- Always handle exceptions and errors using try-catch blocks.
- Throw custom exceptions to provide meaningful error messages to users.
- Use the Flutter debugger to identify and fix errors quickly.
Performance Optimization
Optimizing the performance of your app is crucial for providing a smooth user experience. Here are some tips for optimizing your Flutter app’s performance:
- Avoid unnecessary rebuilds of the UI by using const constructors, final variables, and StatelessWidget where possible.
- Minimize the size of your app by removing unused resources, compressing images, and using code shrinking and obfuscation.
- Use asynchronous programming techniques, such as Futures and Streams, to keep your app responsive and avoid blocking the UI thread.
By following these best practices, developers can create high-quality Flutter apps that are efficient, maintainable, and scalable.
Implementing Effective Strategies for Flutter App Development
Developing high-quality Flutter apps requires more than just knowing the basics of Dart programming. It’s important to implement effective strategies that will streamline the development process and deliver a polished end product. Here are some essential strategies to consider:
1. Utilizing UI Design Patterns
To create a visually appealing and user-friendly app, it’s important to utilize UI design patterns. These patterns help organize the interface, simplify navigation, and ensure a consistent user experience. Common design patterns include tab bars, floating action buttons, and app bars. By implementing these patterns, developers can save time and ensure the app is easy for users to navigate.
2. Implementing State Management
State management is a critical aspect of developing Flutter apps. It ensures that data is consistent and up-to-date across the app, which is particularly crucial when dealing with complex interfaces and a large amount of data. There are various state management techniques developers can use, such as Provider, BLoC, or MobX, each with its own advantages and disadvantages. By choosing the right state management technique for your specific project, you can ensure your app is efficient and scalable.
3. Testing Methodologies
Effective testing methodologies are essential for creating a robust and bug-free app. Unit testing, integration testing, and widget testing are all important techniques to consider. Unit testing ensures that each individual piece of code is working correctly, while integration testing ensures that different components of the app are functioning together as expected. Widget testing focuses on the UI elements and ensures that they are working as they should. By incorporating testing methodologies into your development process, you can detect and fix issues early on, saving time and resources in the long run.
4. Integration with Third-Party Libraries
Integrating third-party libraries can save developers time and effort by providing pre-built components that can be easily implemented into the app. Popular third-party libraries for Flutter apps include Google Maps, Firebase, and Flutter Image Picker. When integrating these libraries, it’s important to thoroughly research and test them to ensure they are reliable and fit for purpose.
By implementing these effective strategies, developers can create high-quality Flutter apps that are efficient, scalable, and user-friendly. Follow these best practices for effective Flutter app development, and see the difference in the end product.
Optimizing Performance in Flutter Apps
Optimizing performance is key to ensuring the success of your Flutter app. By implementing effective Dart coding techniques for Flutter apps, you can significantly improve the user experience. Here are some tips to help you optimize your app’s performance:
Reduce App Size
The size of your app can greatly affect performance, especially for users with slower internet connections. Use techniques like code minification and tree shaking to reduce the size of your app.
Minimize CPU and Memory Usage
Excessive CPU and memory usage can cause your app to slow down, freeze, or crash. To reduce CPU and memory usage, avoid using expensive operations like large loops and recursion. Instead, use asynchronous programming and apply caching techniques wisely to minimize the amount of data processed.
Optimize Rendering Performance
The rendering performance of your app is critical to ensuring a smooth user experience. Use techniques like the Flutter Layout Explorer to identify and fix performance bottlenecks in your app’s UI. Also, make sure to use optimized images and animations to reduce the load on the GPU.
By following these Dart coding techniques for Flutter apps, you can ensure that your app runs smoothly and efficiently. Remember to test your app on different devices and iterate on performance optimization until you achieve the desired results.
Building Robust and Maintainable Flutter Apps
Building a successful Flutter app requires knowledge of best practices for building and maintaining robust code. Following these best practices is crucial to ensure the longevity and scalability of your app, and to make it easier for other developers to work with your code in the future.
Here are some of the top Dart best practices for building Flutter apps:
Best Practice | Description |
---|---|
Name variables, functions, and classes descriptively | Choose clear and concise names for variables, functions, and classes to make your code more readable and easier to understand. Avoid using abbreviations or acronyms that may be unclear to other developers. |
Use error handling | Implement error handling to handle unexpected errors and exceptions, and provide helpful error messages to users. This will make your app more robust and prevent crashes. |
Follow a consistent code style | Consistency in your code style, such as indentation and bracket placement, can make your code more readable and easier to maintain. Use tools like dartfmt to automatically format your code. |
Use version control | Use version control, such as Git, to track changes to your code and collaborate with other developers. This will make it easier to revert changes or merge code changes. |
By following these best practices, you can ensure that your code is maintainable, scalable, and robust. Additionally, documentation is key to the longevity of your project. Documenting your code and keeping your README file up-to-date is a key best practice for long-term maintenance.
Remember to continuously learn and expand your skills to stay up-to-date with the latest developments in Flutter app development. By following these best practices, you can build high-quality apps with confidence.
Advanced Dart Techniques and Examples
Now that we’ve covered the essential best practices for building Flutter apps with Dart, it’s time to take things up a notch. In this section, we’ll explore some advanced Dart coding techniques and provide practical examples of their implementation in Flutter apps.
Generics
Generics are a powerful feature of Dart that allow developers to write reusable code that can work with multiple types. This is particularly useful for collections, such as lists and maps, where the same code can work with different data types. For example:
List<int> numbers = [1, 2, 3];
In this example, we define a list of integers using the List<int>
syntax. This ensures that only integer values can be added to the list, and the Dart compiler will warn us if we try to add a value of a different type.
Mixins
Mixins are a way to reuse code across multiple classes without using inheritance. They allow developers to add functionality to a class without subclassing or duplicating code. For example:
mixin Loggable {
void log(String message) {
print(message);
}
}class MyClass with Loggable {
void doSomething() {
log('Doing something...');
}
}
In this example, we define a Loggable
mixin that adds a log
method to any class that uses it. We then define a MyClass
class that uses the Loggable
mixin and calls the log
method in its doSomething
method.
Asynchronous Programming
Asynchronous programming is essential for building responsive and performant Flutter apps. Dart provides several features for asynchronous programming, including async/await, futures, and streams. For example:
Future<String> fetchData() async {
var response = await http.get('https://example.com/api/data');
return response.body;
}
In this example, we define a fetchData
function that retrieves data from an API using the http.get
method. We use the async/await syntax to make the function asynchronous and return a future that will eventually contain the response body.
Other Advanced Features
Dart has many other advanced features, such as extension methods, isolates, and reflection. These features can be incredibly powerful when used correctly, but they can also make code more complex and harder to maintain. As always, it’s important to consider the trade-offs when using advanced features and use them judiciously.
By mastering these advanced Dart techniques, developers can take their Flutter app development to the next level and create apps that are more performant, maintainable, and scalable.
Conclusion
In conclusion, building Flutter apps the right way requires developers to follow Dart best practices. By adhering to these practices, developers can create robust and maintainable apps that deliver smooth user experiences.
We hope this article has provided you with a comprehensive dive into Dart best practices for Flutter app development. By understanding the advantages of using Flutter for cross-platform app development and leveraging Dart’s powerful programming capabilities, you can build high-performance apps that meet your business needs.
Remember to always keep learning and refining your skills to stay up-to-date with the latest trends and technologies in app development. With the right mindset and best practices, building Flutter apps the right way with Dart can elevate your app development game to new heights.