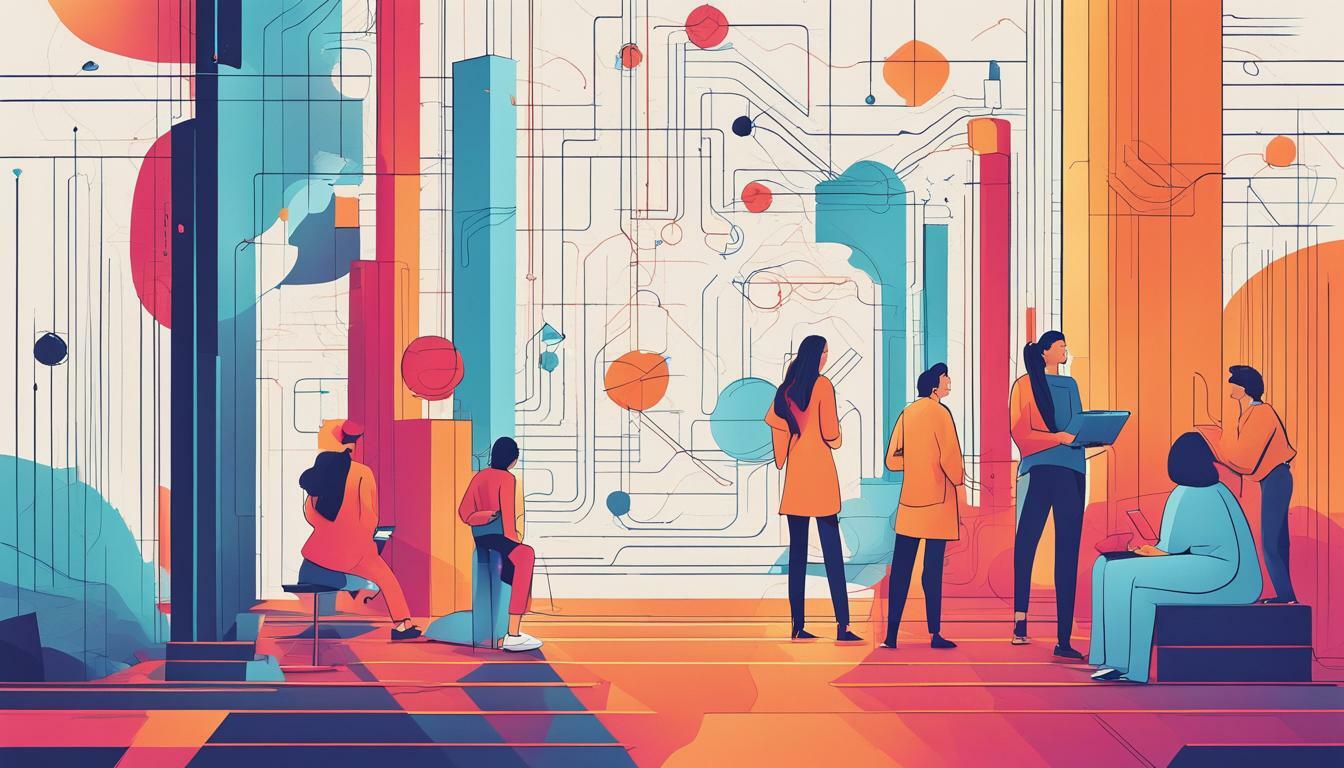
Software design is an integral part of software development, focusing on the organization and structure of software systems. To build robust and maintainable software, developers need to follow the principles of software design. These principles are often referred to as the pillars of software design, and they provide a framework for developing software that is scalable, flexible, and extensible.
In this article, we will explore the pillars of software design and introduce the concept of Solid Principles. We will discuss why it is essential to follow these principles and how they can help create better software. By understanding Solid Principles, developers can enhance the quality, flexibility, and extensibility of their software designs.
Key Takeaways
- The pillars of software design provide a framework for developing software that is robust and maintainable.
- Solid Principles are a set of software design principles that promote software stability, maintainability, and scalability.
- By following Solid Principles, developers can create software that is more reliable, adaptable, and less prone to errors.
Understanding Solid Principles
In software design, Solid Principles are considered best practices for creating maintainable and extensible code. These principles were introduced by Robert C. Martin in his 2000 book, “Agile Software Development, Principles, Patterns, and Practices,” and have since become a fundamental concept in software architecture. By understanding these principles, developers can improve the quality and flexibility of their software design.
What are Solid Principles?
Solid is an acronym for the five principles:
- Single Responsibility Principle (SRP): A class should have only one reason to change. It should only have one job or responsibility.
- Open-Closed Principle (OCP): Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that code should be written in a way that new functionality can be added without altering existing code.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types. This means that objects of a superclass should be able to be replaced with objects of a subclass without affecting the correctness of the program.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use. This means that software should be broken down into smaller, more focused interfaces.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions. This means that code should be written in a way that dependencies are abstracted, allowing for easier changes and testing.
Why are Solid Principles important?
Adhering to Solid Principles leads to cleaner code, easier maintenance, and better overall software architecture. By following these principles, developers can create code that is scalable, adaptable, and less error-prone. Furthermore, by ensuring code is easier to maintain, it can lead to quicker and more efficient updates and improvements over time.
Best Practices for Implementing Solid Principles
When implementing Solid Principles, it is best to start with simple examples and expand on them gradually. By breaking down the concepts into smaller pieces, it is easier to understand and apply them effectively to larger projects. Additionally, it is important to test code frequently to ensure that it is adhering to the principles and achieving the desired results. Finally, it is important to stay up-to-date on the latest software design patterns and practices to ensure continued success and improvement.
The Importance of Solid Principles in Software Design
Software development is a complex process that requires careful attention to detail. Adhering to best practices such as solid principles is essential for developing software that can withstand the test of time. In this section, we will delve deeper into the significance of solid principles in software design, explain what they are, and how they can enhance software architecture.
What are Solid Principles?
Solid Principles are a set of software design principles that were introduced by Robert C. Martin (also known as Uncle Bob) in his book “Agile Software Development, Principles, Patterns, and Practices” in 2003. The five principles are:
- The Single Responsibility Principle (SRP)
- The Open-Closed Principle (OCP)
- The Liskov Substitution Principle (LSP)
- The Interface Segregation Principle (ISP)
- The Dependency Inversion Principle (DIP)
The Significance of Solid Principles in Software Design
When developers follow solid principles, they are more likely to produce software that is easy to understand, debug, and maintain. Adhering to these best practices can lead to more scalable, adaptable, and flexible software architectures. By breaking software down into smaller, more manageable pieces, and making each piece as independent as possible, the codebase is more resilient to change, easier to modify, and less prone to errors.
Moreover, solid principles can help developers avoid common pitfalls and errors that plague software development. For example, the Single Responsibility Principle states that a class should do one thing and do it well. This principle helps to prevent classes from becoming too complex or interdependent, leading to cleaner and more readable code.
Explaining Solid Principles
The Single Responsibility Principle (SRP) suggests that a class should have only one responsibility. By assigning responsibilities to specific classes, developers can ensure that each class contains only the necessary code to perform its function. This makes it easier to maintain and modify code, as well as to reuse code in other parts of the software architecture.
The Open-Closed Principle (OCP) states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that developers should be able to extend the behavior of software entities without modifying their source code. By doing so, developers can ensure that their code is more flexible, modular, and reusable.
The Liskov Substitution Principle (LSP) suggests that objects of a superclass should be replaceable by objects of its subclasses without causing any unexpected behavior. By following this principle, developers can ensure that their code is more adaptable, maintainable, and reliable.
The Interface Segregation Principle (ISP) states that clients should not be forced to depend on interfaces that they do not use. By following this principle, developers can avoid tight coupling and increase modularity. This makes it easier to modify and maintain code, as well as to reuse code in other parts of the software architecture.
The Dependency Inversion Principle (DIP) suggests that high-level modules should not depend on low-level modules, but both should depend on abstractions. By following this principle, developers can avoid tight coupling and increase modularity. This makes it easier to modify and maintain code, as well as to reuse code in other parts of the software architecture.
In conclusion, Solid Principles are essential for producing software that is more maintainable, adaptable, and scalable. By adhering to these best practices, developers can achieve cleaner, easier-to-read code that is less prone to bugs and errors. We hope that this section has clarified the significance of solid principles in software design and motivated you to apply them in your own work.
Applying Solid Principles in Real-world Scenarios
Now that we have discussed the Solid Principles in detail, let’s explore how these principles can be applied in real-world scenarios, improving the overall software architecture.
One common scenario is when developers are faced with a complex codebase that lacks clarity and structure. By applying the Single Responsibility Principle, developers can break down the codebase into smaller, more manageable components, making it easier to understand and maintain.
Another scenario where Solid Principles can be applied is when scaling the software system. Dependency Inversion Principle can be used to decouple the software components, making the system more adaptable to change and easier to maintain. In addition, Interface Segregation Principle can be used to create modular components that can be reused across the system, reducing the overall complexity of the software architecture.
Finally, applying the Open-Closed Principle can make the software system more flexible and extensible. By designing the software system in modules, developers can add new features or functionality without modifying the existing codebase. This makes the system less prone to errors and easier to maintain.
In summary, applying Solid Principles in real-world scenarios can lead to cleaner code, better maintainability, and more scalable software architecture. By following the best practices of Solid Principles, developers can ensure that their software systems are robust, flexible, and better suited to adapt to future changes.
NLP for Software Design: Enhancing Solid Principles
Incorporating Natural Language Processing (NLP) techniques into software design can enhance the application of Solid Principles. NLP can help developers by improving code readability, speeding up the development process, and optimizing the use of Solid Principles.
One way that NLP can help is by identifying patterns and commonalities in software code. By using NLP algorithms to analyze code, developers can find areas where they can apply Solid Principles more effectively. This can help to increase code quality and reduce the likelihood of bugs or errors.
NLP can also help to improve code readability. By using NLP to analyze code and provide suggestions for clearer naming conventions, developers can make their code more understandable to other team members. This can help to improve collaboration and reduce the time spent on code reviews.
NLP can also assist with generating software documentation. By using NLP algorithms to analyze code comments and generate documentation automatically, developers can save time and ensure that documentation is consistent and up to date.
Overall, NLP techniques can complement Solid Principles and provide new avenues for optimizing software design. By leveraging the power of NLP, developers can take their understanding and application of Solid Principles to the next level.
Best Practices for Incorporating Solid Principles
Implementing Solid Principles in software design can be a daunting task, but by following some best practices and guidelines, developers can ensure that their software systems are built with robustness and flexibility in mind. Here are some tips:
Refactor existing codebases
If you are working with an existing codebase, it’s essential to refactor it to incorporate Solid Principles gradually. Refactoring means modifying the code without changing its functionality. Developers can start by identifying classes or methods with more than one responsibility and break them into smaller, more focused classes or methods. This practice adheres to the Single Responsibility Principle and makes the code more maintainable.
Design with Solid Principles in mind
If you are starting a new project, it’s best to design the software system with Solid Principles in mind. Developers should focus on creating a modular and extensible design that adheres to the Open-Closed Principle. This approach ensures that the software architecture can accommodate future changes without needing significant modification.
Apply the Dependency Inversion Principle
The Dependency Inversion Principle is critical in software design since it decouples higher-level modules from lower-level modules. This decoupling ensures that a change in one module does not affect other modules in the system. Developers should use dependency injection to implement this principle effectively.
Write clear and concise code
Clean Code is essential when incorporating Solid Principles. Developers should write meaningful comments and use descriptive names for methods, classes, and variables. They should avoid using abbreviations, and the code should be concise and easy to read.
Use automated testing
Automated testing is a crucial aspect of software development. Developers should use automated testing tools to ensure that their software systems are reliable and maintainable. By testing the code regularly, developers can identify and fix bugs early on in the development process, adhering to the SOLID principles.
By incorporating these best practices, developers can ensure that their software systems adhere to Solid Principles. By following the SOLID principles, software architects can create maintainable, robust, and scalable software architecture. This approach can save time and money in the long run and make software systems more adaptable to future changes.
Conclusion
In conclusion, understanding and applying Solid Principles in software design is essential for building robust and maintainable software systems. Adhering to these principles leads to cleaner code, improved scalability, and easier testing. By incorporating best practices and guidelines for Solid Principles, developers can ensure that their software designs are both flexible and adaptable.
The practical examples and scenarios discussed in this article provide valuable insights into how to effectively implement Solid Principles in software architecture. Furthermore, leveraging Natural Language Processing (NLP) techniques can enhance the application of Solid Principles and improve code readability and developer productivity.
Overall, Solid Principles are critical for creating software systems that are scalable, adaptable, and less prone to bugs or errors. By embracing these principles, developers can ensure that their software designs are reliable and maintainable, ultimately leading to greater success for their projects.
FAQ
Q: What are the pillars of software design?
A: The pillars of software design are principles that serve as guidelines for creating robust and maintainable software systems. They include the Single Responsibility Principle, Open-Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle.
Q: Why are Solid Principles important in software architecture?
A: Solid Principles are important in software architecture because they help ensure that software designs are scalable, adaptable, and less prone to bugs or errors. By following these principles, developers can create cleaner code, improve maintainability, facilitate easier testing, and enhance overall software architecture.
Q: How can developers apply Solid Principles in real-world scenarios?
A: Developers can apply Solid Principles in real-world scenarios by using practical examples and demonstrating how these principles can effectively solve common software design challenges. By understanding and implementing Solid Principles, developers can improve their software architecture and create more robust and flexible systems.
Q: How can Natural Language Processing (NLP) enhance Solid Principles in software design?
A: Natural Language Processing (NLP) techniques can complement Solid Principles in software design by improving code readability, increasing developer productivity, and optimizing the application of these principles. By leveraging NLP, developers can further enhance their understanding and application of Solid Principles in their software designs.
Q: What are the best practices for incorporating Solid Principles in software design?
A: The best practices for incorporating Solid Principles in software design include refactoring existing codebases to adhere to the principles and designing new software systems with these principles in mind. By following these best practices, developers can ensure that their software designs are both robust and flexible.
Q: Why are Solid Principles important in software design?
A: Solid Principles are important in software design because they help ensure that software systems are reliable, maintainable, and scalable. By adhering to these principles, developers can create software that is easier to understand, modify, and test, leading to more efficient and effective development processes.