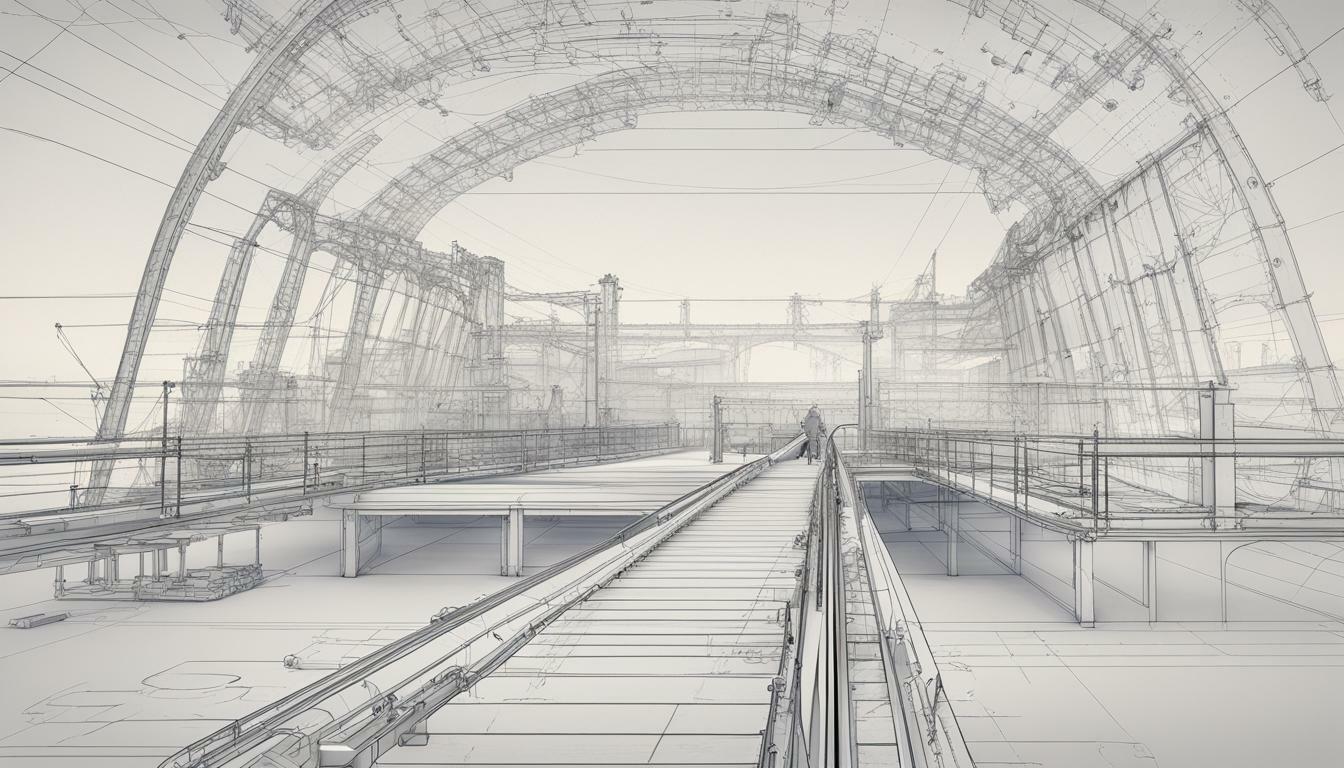
Welcome to our article on structuring your .NET Core API for clarity. Whether you’re a seasoned developer or just starting with .NET Core API development, understanding how to structure your API is crucial for creating a maintainable and scalable system. In this section, we will explore the role of models and controllers in API architecture, and provide guidelines for effective API structuring.
When it comes to API design, clarity is key. By organizing your API in a clear and structured manner, you can improve readability, reduce maintenance costs, and facilitate collaboration among team members. To achieve this goal, it’s important to understand the role of models and controllers in .NET Core API development.
Key Takeaways
- Structuring your .NET Core API for clarity is essential for creating a maintainable and scalable system.
- Models and controllers play a crucial role in API architecture.
- An effective API structure is built on a set of best practices, including organizing project files, naming conventions, and implementing a layered architecture.
Understanding the Role of Models and Controllers in .NET Core API
Controllers are critical components in .NET Core API development. They are responsible for handling the requests and responses between the client and server, determining the appropriate response based on the content of the request. Controllers receive requests from the client, query the necessary data from the data store, and return the result in the requested format.
In contrast, models in .NET Core API development represent the data entities and business logic. Models define the data structure and the relationships between different entities in the API. They interact with the database and handle the CRUD (Create, Read, Update, Delete) operations for each entity in the system.
By understanding and separating the roles of models and controllers, developers can organize their API architecture and improve the API clarity. For instance, developers can organize their codebase into separate folders for models and controllers, making it easier to navigate, modify, and maintain.
Moreover, separating the responsibilities of models and controllers enables developers to write cleaner, more modular code. Writing modular code makes the API more scalable and testable, as different parts of the system can be tested and updated independently.
Overall, understanding the role of models and controllers is crucial for developing a well-structured and maintainable .NET Core API. Separating the concerns of models and controllers and organizing them effectively will lead to an API that is easy to understand, navigate and modify.
Best Practices for API Structuring in .NET Core
When it comes to structuring your .NET Core API, following best practices can make a world of difference in terms of clarity and maintainability. Here are some key guidelines to keep in mind:
Organize Your Project Files
Before you start developing your API, take the time to organize your project files in a logical and consistent manner. This can include grouping related files together, creating separate directories for different components (e.g. controllers, models, views), and using clear and descriptive names for your files and directories. By doing so, you can make it easier to find and modify specific parts of your API code.
Follow Naming Conventions
Consistent naming conventions can play a key role in making your API code more readable and understandable. Consider using standard naming conventions for your classes, methods, variables, and other components, and make sure that your names accurately reflect the purpose and functionality of each component. This can make it easier for other developers to understand your code and to work collaboratively on your API.
Separate Concerns
One important principle of API structuring is the separation of concerns. In other words, you should aim to keep different parts of your API code separate and focused on specific tasks or functionalities. This can involve using different controllers for different endpoints, separating business logic from data access code, and using helper classes or utilities to keep common code separate from specific API functionality. By separating concerns, you can make your API more modular and easier to maintain over time.
Use a Layered Architecture
Another best practice for API structuring is to use a layered architecture. This involves separating your API code into different layers, each with a specific responsibility and set of functionalities. For example, you might have a presentation layer (controllers and views), a business logic layer (models and services), and a data access layer (repositories and database access code). By using a layered architecture, you can improve the flexibility, scalability, and maintainability of your API code.
By following these best practices, you can create a well-structured .NET Core API that is easy to understand, navigate, and maintain. Take the time to plan and organize your API code before you start developing, and make sure to follow consistent naming conventions, separate concerns, and use a layered architecture. With these principles in mind, you can build a high-quality API that meets your needs and those of your users.
Implementing an Optimal, SEO-Friendly Hierarchical Structure
Once you have organized your project files and established an API structure using models and controllers, the next step is to optimize its hierarchical structure for SEO purposes. The goal is to make your API easily discoverable and user-friendly.
Organizing Your Endpoints
Your API endpoints should be organized hierarchically, with related endpoints grouped together. This makes it easier for users to navigate your API and find the endpoints they need. Use clear and concise endpoint names that reflect the actions they perform.
For example, if you have an API for an e-commerce site, you might have endpoints such as /api/products, /api/categories, and /api/orders. By organizing your endpoints hierarchically and following naming conventions, users can easily navigate and understand your API.
Versioning Your API
It’s important to version your API to maintain backwards compatibility and ensure that changes to your API do not break existing applications. Use a versioning scheme that clearly indicates the API version, such as /api/v1/products.
You can also use query strings to indicate the API version, such as /api/products?api-version=1.0. Again, clear and consistent naming conventions will make your API easier to understand and use.
Implementing Proper Routing
Proper routing is essential for a well-structured API. Use attribute routing to define your API routes and endpoints, and use parameter constraints to validate user input. You can also use route prefixes to group related endpoints together.
For example, you might use the route prefix /api/v1 to group all version 1 endpoints together, and use attribute routing to define individual endpoints within that group. By implementing proper routing, you can make your API more efficient and easier to use.
In conclusion, optimizing your API’s hierarchical structure is crucial for improving its usability and discoverability. By organizing your endpoints hierarchically, versioning your API, and implementing proper routing, you can create an API that is well-structured, user-friendly, and optimized for SEO.
Conclusion
Structuring your .NET Core API for clarity is crucial for building an effective and maintainable API. By following the guidelines and best practices outlined in this article, you can create an API that is easy to navigate and understand.
API Clarity is Key
API clarity is key when it comes to .NET Core API development. By organizing your project files, separating concerns, and implementing a layered architecture, you can achieve a clean and maintainable API structure. Remember to also implement an optimal and SEO-friendly hierarchical structure for your endpoints.
Best Practices
Follow best practices for API structuring, such as naming conventions and separating concerns. These practices help create a well-designed API structure that can be easily maintained and scaled.
Remember that models represent the data entities and business logic, while controllers handle the requests and responses. Understanding this relationship is important for structuring your API in a clear and organized manner.
Keep it Clear and Effective
As you develop your .NET Core API, keep the principles of clarity and effectiveness in mind. By creating an API that is easy to understand, navigate, and scale, you can build a valuable tool for your users.
Overall, structuring your .NET Core API for clarity is an essential step in the development process. By following these guidelines, you can build an API that is both effective and maintainable for the long term.
FAQ
Q: Why is structuring my .NET Core API important?
A: Structuring your .NET Core API is important because it improves readability and maintainability. A well-structured API allows developers to easily understand and navigate the codebase, making it easier to maintain and enhance in the future.
Q: What is the role of models and controllers in .NET Core API development?
A: Models represent the data entities and business logic in your .NET Core API, while controllers handle the requests and responses. Models define the structure of your data, while controllers handle the logic and flow of the API, making them essential components of the API architecture.
Q: What are some best practices for structuring my .NET Core API?
A: Some best practices for API structuring in .NET Core include organizing project files, following naming conventions, separating concerns, and implementing a layered architecture. These practices help ensure a clean and maintainable API structure.
Q: How can I implement an optimal hierarchical structure for my .NET Core API?
A: To implement an optimal hierarchical structure for your .NET Core API, you should focus on organizing your endpoints, versioning your API, and implementing proper routing. This helps enhance the discoverability and usability of your API.
Q: Why should I prioritize clarity in my .NET Core API development?
A: Prioritizing clarity in your .NET Core API development is important because it helps ensure that your API is easy to understand, navigate, and scale. With a clear and organized structure, developers can easily work with the API and maintain its functionality over time.