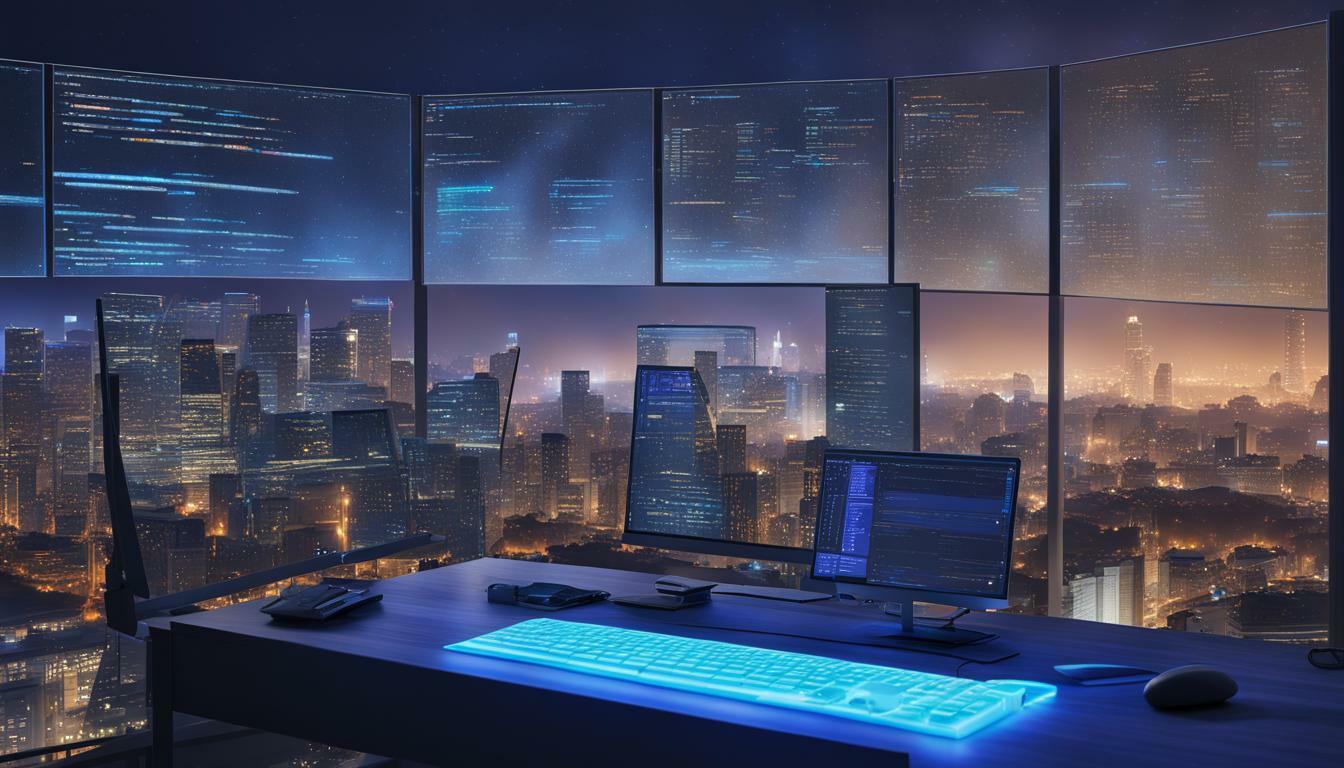
Welcome to our comprehensive guide to mastering .NET API development. In this article, we will cover the essential best practices that are crucial for success in this field. From understanding the fundamentals to designing efficient APIs, implementing security measures, and optimizing performance, we’ll provide you with all the necessary knowledge to excel.
We’ll also include examples to illustrate these best practices, giving you a practical understanding of how to implement them in your own projects. Whether you’re a beginner or an experienced developer, this guide will help you take your .NET API development skills to the next level.
Understanding API Development with .NET
Welcome to section two of our article on .NET API development best practices. In this section, we will dive deeper into the world of API development with .NET and explore the important considerations and best practices specific to this platform.
API development with .NET involves creating and exposing interfaces that allow different software applications to communicate with each other seamlessly. These interfaces can be accessed both internally and externally, making them a crucial aspect of modern software development.
Why Use .NET for API Development?
Using .NET for API development comes with several advantages. Firstly, .NET is a highly versatile platform that supports multiple programming languages, making it accessible to a broader range of developers. Additionally, .NET provides excellent support for developing scalable and secure APIs, which is crucial for large-scale applications.
Best Practices in .NET API Development
When developing APIs with .NET, it is essential to follow best practices to ensure the effectiveness and longevity of the interface. Here are some of the best practices for .NET API development:
- Separate concerns: Ensure that your API separates concerns by separating business logic from data access and presentation layers. This will make your API more maintainable and scalable over time.
- Use HTTP methods: Use appropriate HTTP methods for different API endpoints to improve readability, maintainability and make it easier for developers to understand your API.
- Implement caching: Implement caching to improve API performance and reduce the number of requests to your API server, decreasing response times for repeated requests.
Examples of Best Practices in .NET API Development
Here are some examples of best practices in .NET API development:
Best Practice | Example |
---|---|
Separate concerns | Separating business logic in a separate class to increase maintainability. |
Use HTTP methods | Using GET method for retrieving data and POST method for adding data |
Implement caching | Using Redis Cache for storing API responses and avoiding generating the same response repeatedly. |
By following these best practices, you can build robust, scalable, and secure APIs with .NET that meet the requirements of modern software development.
Designing Efficient APIs with .NET
When it comes to API development, designing efficient APIs is crucial for success. There are several best practices that should be followed when designing APIs, especially in .NET. Here are some top tips for designing efficient APIs with .NET.
1. Keep it simple
One of the most important things to keep in mind when designing APIs is to keep it simple. Your APIs should be easy to understand and use. Use clear and concise names for your endpoints, methods, and parameters. Avoid complex data structures and keep your API responses as simple as possible.
2. Use HTTP Verbs Appropriately
Another best practice for designing APIs with .NET is to use HTTP verbs appropriately. Use GET requests for retrieving data, POST for creating objects, PUT for updating existing objects, and DELETE to remove data. Using the appropriate verb will make your API easier to understand and use.
3. Follow RESTful API standards
RESTful API standards provide a set of guidelines for designing APIs that are easy to use, maintain, and scale. Follow these standards when designing your API with .NET. Use nouns for your endpoint URLs and ensure they represent a resource. Use HTTP response status codes to indicate the status of the request.
4. Version your APIs
Versioning your APIs is critical for maintaining backward compatibility. As you evolve your APIs, you don’t want to break existing client applications that rely on them. By versioning your APIs, you can ensure that changes to your API don’t break existing client applications.
5. Use caching
Caching can significantly improve the performance of your API. Use caching where appropriate to reduce the number of calls to your API. However, be careful not to cache sensitive data or data that frequently changes.
By following these best practices, you can create efficient APIs with .NET. Use these tips as a guide when designing your APIs, and be sure to test your APIs thoroughly to ensure they are efficient and robust.
Implementing Security in .NET API Development
Security is a vital aspect of any API development, and .NET API development is no exception. Implementing strong security measures not only helps to protect data but also instills confidence in end-users. Below are some essential best practices to consider when implementing security in your .NET API development:
Authentication and Authorization
Authentication is the process of verifying a user’s identity, while authorization determines what data or actions a user is allowed to access. In .NET API development, it is good practice to use authentication and authorization mechanisms such as OAuth or JWT tokens.
Here are some .NET API examples of how to implement authentication and authorization:
Technology | Example |
---|---|
ASP.NET Core Identity | Allows for easy integration of authentication and authorization with a web application. |
IdentityServer4 | Provides a comprehensive identity server solution for API authentication and authorization. |
Encryption
Encryption is the process of converting plain text or data into a coded message to prevent unauthorized access. In .NET API development, it is essential to encrypt sensitive data such as passwords or credit card information. .NET offers various encryption libraries such as Cryptography and Bcrypt.
Here are some .NET API examples of how to implement encryption:
- Using Cryptography libraries to encrypt and decrypt sensitive data
- Using Bcrypt to hash passwords for secure storage and authentication
API Key Management
API key management involves issuing and managing API keys that control access to APIs. In .NET API development, API keys can be implemented using various techniques such as API Management and Azure Key Vault.
Here are some .NET API examples of how to implement API key management:
- Using Azure API Management to issue and control access to API keys
- Using Azure Key Vault to store and manage API keys securely
By implementing these essential best practices in your .NET API development, you can create secure and reliable APIs that protect data while maintaining high-performance standards.
Optimizing Performance in .NET API Development
High-performing APIs are critical in today’s fast-paced technological environment. To achieve optimal performance of .NET APIs, it is essential to incorporate the following best practices:
- Use caching: Caching is a powerful technique that can significantly improve API performance. By caching frequently requested data, the load on the API server can be reduced, resulting in faster response times for clients.
- Minimize network trips: Each network trip introduces latency, which can result in slower API performance. To minimize network trips, it is advisable to combine multiple requests into a single call wherever possible. This can be achieved using batch requests or data aggregation techniques, such as GraphQL.
- Optimize database queries: Database queries can be a significant bottleneck in API performance. By optimizing queries and database indexing, the response times of APIs can be significantly improved.
By incorporating these best practices, developers can create high-performing .NET APIs that deliver fast and efficient responses to clients.
Error Handling and Exception Management in .NET API Development
When it comes to robust API development, error handling and exception management are essential components. Knowing the best practices for handling errors and exceptions in .NET API development can help developers create more reliable APIs.
One of the fundamental practices in error handling is to develop a clear understanding of the different types of errors that can occur in an API. Common errors include bad requests, authentication errors, and server errors. By recognizing these errors and their causes, developers can design APIs that respond appropriately to them.
In addition to recognizing errors, creating clear and informative error messages is also crucial. Error messages should provide enough information to help users understand what went wrong and how to fix it. They should also be consistent throughout the API and include error codes for easier debugging.
Exception management is another essential aspect of error handling in .NET API development. Exceptions are runtime errors that may occur during the execution of an API. Handling exceptions effectively can help prevent application crashes and improve the overall stability of the API.
One of the best practices for exception management is to use a global error handler to catch and handle unhandled exceptions. The error handler should log the details of the exception for debugging purposes and return an appropriate error response to the user.
To illustrate these practices, consider the following example of error handling in a .NET API.
Error Type | Error Code | Error Message |
---|---|---|
Bad Request | 400 | “The request could not be understood or was missing required parameters.” |
Authentication Error | 401 | “The request requires authentication credentials.” |
Server Error | 500 | “An unexpected error occurred on the server.” |
In conclusion, understanding the best practices for error handling and exception management in .NET API development is crucial for creating reliable and stable APIs. By recognizing the different types of errors that can occur, creating clear error messages, and effectively managing exceptions, developers can create APIs that are more user-friendly and easier to maintain.
Testing and Documentation in .NET API Development
Proper testing and documentation are vital for successful API development. It is crucial to ensure that the API functions as intended and that all intended functionality is documented. Best practices for testing and documenting .NET APIs are as follows:
- Unit Testing: It is essential to perform unit testing to ensure that each individual function of the API works as intended. This can be achieved using tools such as NUnit or Microsoft’s built-in testing tools.
- Integration Testing: Integration testing should be performed to ensure that all components of the API work together as intended. This can be achieved using tools such as Postman or SoapUI.
- Documentation: Complete documentation of the API is crucial for users to understand its functionality and how to use it. Documentation should include information on all functions, parameters, and return types, as well as any relevant example code.
Real-world examples of testing and documentation in .NET API development include:
“We used NUnit to perform unit testing on all of our API’s functions and verified their results against expected values. We also used Postman to perform integration testing to ensure that all components of the API worked together as intended. All API functions were documented using Swagger, including information on parameters, return types, and example requests and responses.”
By following these best practices for testing and documentation, developers can ensure that their .NET APIs are reliable, efficient, and easy to use.
Conclusion
In this article, we have explored the essential best practices for .NET API development. By following these practices and learning from the examples provided, developers can create efficient and robust APIs using .NET. We have discussed the importance of understanding API development with .NET and the specific considerations and best practices for designing efficient APIs. Additionally, we have covered key aspects of API development such as security, performance optimization, and error handling and exception management.
Proper testing and documentation are crucial for successful API development, and we have provided best practices for these aspects of .NET API development. Overall, this article has provided a comprehensive overview of the best practices in .NET API development, along with real-world examples to illustrate these practices.
Start Developing Efficient APIs with .NET Today
Implementing the best practices described in this article is indispensable to creating efficient and reliable APIs with .NET. Start developing your own APIs with .NET by following these best practices and learning from the examples provided. Remember to prioritize efficient design, security, performance optimization, error handling, and testing and documentation throughout your development process.
By mastering these essential best practices in .NET API development, you can create powerful and effective APIs that will help you achieve your development goals. Good luck and happy developing!