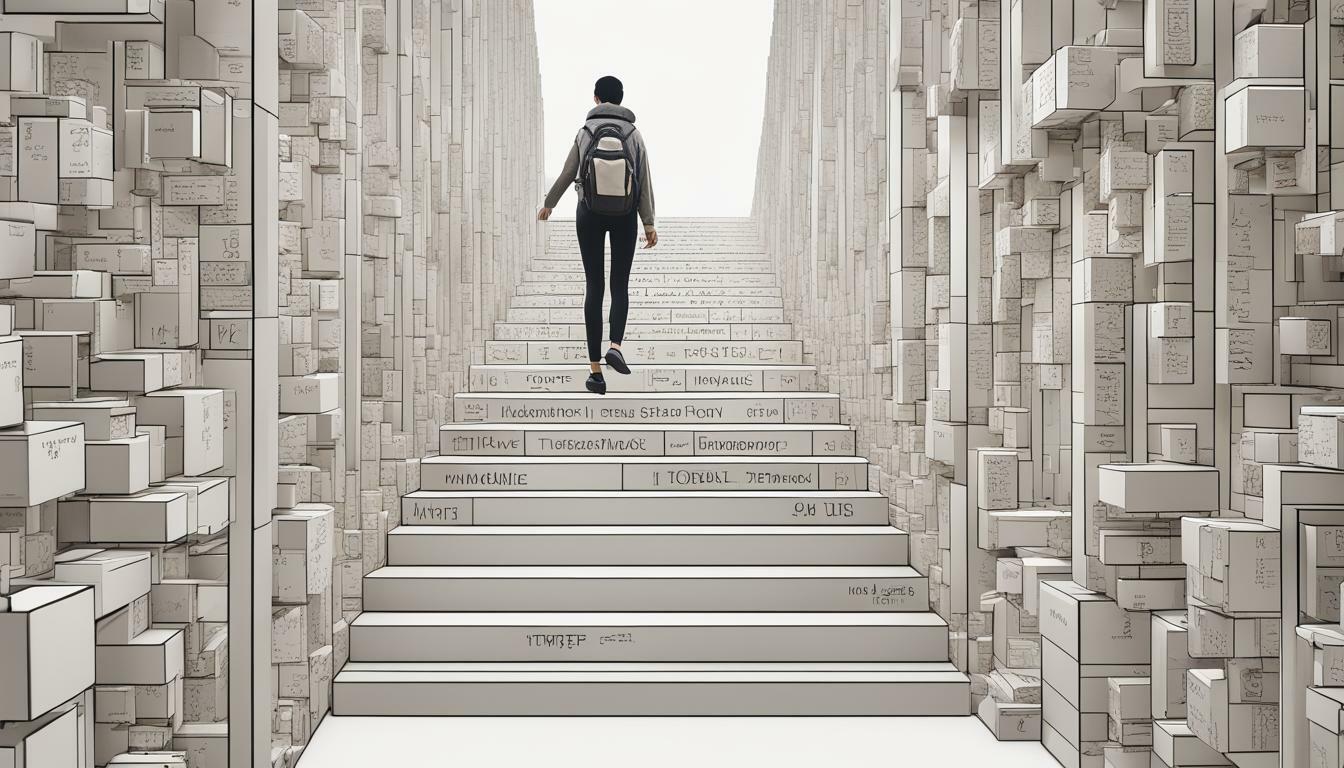
Functional programming is a programming paradigm that emphasizes the use of functions to build software applications. It is a popular approach among developers due to its efficiency, scalability, and maintainability. This article will provide a comprehensive guide to mastering functional programming, covering the basic concepts, benefits, and popular languages and paradigms. We will also compare functional programming with imperative programming and provide real-world examples of functional code.
If you are new to functional programming or looking to improve your existing skills, this guide is for you. By the end of this article, you will have a solid understanding of functional programming and the tools to implement it in your projects.
Key Takeaways:
- Functional programming emphasizes the use of functions to build software applications.
- It is efficient, scalable, and maintainable.
- In this article, we will cover the basic concepts, benefits, and popular languages and paradigms of functional programming.
- By the end of this guide, you will have the skills to implement functional programming in your projects.
Understanding Functional Programming Concepts
Functional programming is a programming paradigm that focuses on the evaluation of functions rather than the execution of commands. This section will explain some of the core concepts that make functional programming unique and powerful.
Immutability
In functional programming, data is immutable, which means that once a value is assigned, it cannot be changed. Instead of modifying existing values, functional programming emphasizes the creation of new values based on existing ones. This approach leads to more predictable and transparent code.
Pure Functions
Pure functions are functions that always return the same output given the same input, and have no side effects beyond their return value. This means that they do not modify any outside state, making them predictable and easy to reason about. Pure functions are a cornerstone of functional programming, as they can be composed and reused without causing unexpected behavior.
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments, or return functions as their output. This allows for powerful abstractions and composability in functional programming. For example, map, filter, and reduce are common higher-order functions used in functional programming.
Recursion
Recursion is a technique that involves defining a function in terms of itself. In functional programming, recursion is often used as a replacement for loops, as it provides a more declarative and concise way of iterating over data structures. However, care must be taken to avoid infinite recursion.
These are just a few of the core concepts that make functional programming a unique and powerful approach to programming. Understanding these concepts can help developers write more efficient, scalable, and maintainable code.
Benefits of Functional Programming
Functional programming offers numerous benefits that can revolutionize the way software is developed. Here are some of the key advantages:
- Improved code readability: Functional programming emphasizes on writing code that is easy to understand and follow. By using pure functions and avoiding side effects, functional code becomes highly readable and modular.
- Better maintainability: The modular nature of functional programming makes it easier to maintain, debug, and modify code. Changes made to one part of the program do not affect other parts, reducing the chances of introducing bugs.
- Enhanced scalability: Functional programming encourages writing code that can scale easily, making it a perfect choice for large-scale applications that need to handle a lot of data and users.
- Easier parallelization: In functional programming, since functions do not have side effects and share no state, they can be easily parallelized. This means that multiple computations can be done simultaneously, improving performance and efficiency.
Functional programming is not just better in terms of code quality, but it also offers a more efficient and reliable way to develop software. By embracing functional programming principles, developers can create high-quality, maintainable software that is easy to scale and adapt to changing requirements.
Functional Programming vs. Imperative Programming
Functional programming and imperative programming are two different approaches to coding that have their own unique strengths and weaknesses. While imperative programming focuses on changing the state of a program, functional programming emphasizes immutability and working with functions.
One of the key differences between these two approaches is mutability. Imperative programming relies on mutable variables and objects, which can lead to bugs and other issues if not managed properly. In contrast, functional programming emphasizes immutability, meaning that values once assigned cannot be changed. This reduces the likelihood of errors and makes code easier to reason about.
Another significant difference is the use of side effects. Imperative programming frequently relies on side effects, which can cause issues with dependencies and make debugging more difficult. Functional programming, on the other hand, avoids side effects in favor of pure functions that only take in input and return output without modifying any external state. This can make code easier to test and simpler to maintain.
Functional programming and imperative programming also have different programming styles. Imperative programming is often more procedural, with code that is written in a step-by-step manner. Functional programming, on the other hand, is more declarative, with code that describes what is being done rather than how it is being done.
So when and why should you use functional programming over imperative programming? Functional programming is often a better choice for tasks that require complex data transformations, parallel processing, or other tasks that rely heavily on mathematical functions. Additionally, functional programming can often produce more concise and readable code than imperative programming, making it easier to maintain and scale.
Principles of Functional Programming
Functional programming is built on a set of principles that ensure code efficiency and maintainability. Here are some essential functional programming principles:
Referential Transparency
Referential transparency refers to the property of functions that ensures they always return the same output for a given input. This enables easier code testing and optimization, as it eliminates side effects and mutable state.
Composability
Composability is the ability of functions to be combined and reused in different contexts. This principle ensures modularity and flexibility in code design, making it easier to maintain and scale.
Declarative Programming
Declarative programming emphasizes the WHAT rather than the HOW in coding. This means that functions should focus on describing the desired outcome, rather than specifying the steps to achieve it. This results in more readable and concise code, with fewer bugs and dependencies.
By adhering to these principles, functional programming allows developers to build reliable, scalable, and efficient software applications.
Popular Functional Programming Paradigms
Functional programming offers a variety of paradigms that enable developers to write efficient and maintainable code. Here are some popular paradigms:
Pure Functional Programming:
A paradigm that emphasizes immutability, pure functions, and referential transparency. This approach avoids side effects, making the code more predictable and easier to test.
Object-functional Programming:
A hybrid paradigm that combines the principles of object-oriented programming with functional programming. This approach makes use of higher-order functions and immutable data structures while also allowing for encapsulation and polymorphism.
Concurrent Programming:
A paradigm that enables developers to write code that can execute multiple tasks simultaneously. This approach is useful for applications that require high-performance or real-time data processing.
The choice of paradigm depends on the specific requirements of the application, but understanding the advantages and limitations of each can inform the development process and lead to more efficient and scalable code.
Functional Programming Languages: A Comprehensive List
If you’re looking to explore the world of functional programming, you’ll need to start by selecting a language that aligns with your goals and needs. Here is a list of popular functional programming languages that you can consider:
Language | Features | Common Use Cases |
---|---|---|
Haskell | Lazy evaluation, type inference, purity, and strong static typing | Web development, finance, scientific research |
Scala | Object-oriented and functional, strong static typing, interoperability with Java | Big data processing, web development, finance |
Clojure | Lisp-based, immutable data structures, concurrency, JVM compatibility | Web development, data analysis, concurrency |
Erlang | Concurrency, fault tolerance, pattern matching, distribution | Telecommunications, messaging systems, web servers |
F# | Interoperability with .NET Framework, async programming, type inference | Web development, finance, machine learning |
Elm | Browser-based, strong static typing, reactive programming | Web development, UI design |
Of course, this is not an exhaustive list, and new functional programming languages are emerging all the time. However, these languages are a great starting point for both beginners and experienced developers.
Examples of Functional Programming
Functional programming offers several techniques that can be used to write concise and efficient code. Let’s explore some common examples of functional programming concepts:
-
Map: The map function is used to apply a function to each element of a list or collection, returning a new list with the transformed elements. For example:
const numbers = [1, 2, 3, 4, 5]; const doubledNumbers = numbers.map(num => num * 2); console.log(doubledNumbers); // [2, 4, 6, 8, 10]
-
Filter: The filter function is used to select elements from a list that pass a certain condition, returning a new list with the selected elements. For example:
const numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter(num => num % 2 === 0); console.log(evenNumbers); // [2, 4]
-
Reduce: The reduce function is used to combine and aggregate elements of a list into a single value, using an accumulator and a combining function. For example:
const numbers = [1, 2, 3, 4, 5]; const sum = numbers.reduce((acc, num) => acc + num, 0); console.log(sum); // 15
-
Recursion: In functional programming, recursion is often preferred over traditional loop constructs. A function calls itself repeatedly, with a modified argument, until it reaches a base case. For example:
function factorial(n) { if (n === 0) { return 1; } return n * factorial(n - 1); } console.log(factorial(5)); // 120
By using these functional programming techniques, you can write code that is more concise, modular, and reusable. These concepts are particularly useful when working with large datasets or complex systems, where performance and scalability are critical.
Best Practices for Functional Programming
Functional programming can be challenging to master, but following best practices can make it easier to write clean, maintainable, and efficient code. Here are some best practices to consider:
- Write pure functions. Pure functions are functions that always return the same output for a given input, without any side effects. This makes them easier to reason about, test, and maintain.
- Avoid mutable state. Functional programming encourages immutability, which means avoiding changing the state of variables or objects. Immutability makes code more predictable and easier to parallelize.
- Use higher-order functions. Higher-order functions are functions that take other functions as arguments or return them as outputs. They can help make code more modular and reusable.
- Minimize side effects. Side effects are changes to the state of the program that are not reflected in the return value of a function. Functional programming aims to minimize side effects, as they make code harder to reason about.
- Use recursion. Recursion is a core concept in functional programming that allows functions to call themselves. It can be used to solve many problems more elegantly and efficiently than iterative loops.
- Compose functions. Functional programming encourages function composition, which means combining small, focused functions to create more complex behavior. This can make code more modular, reusable, and easier to reason about.
- Handle errors gracefully. Functional programming encourages proper error handling, such as using option or Either types to handle null or error values. This can make code more robust and prevent unexpected crashes.
- Write testable code. Functional programming can make it easier to write unit tests, as it encourages pure functions and minimizes side effects. Writing testable code can help catch bugs early and make code more reliable.
By following these best practices, developers can write more efficient, maintainable, and reliable functional code. However, it’s important to remember that best practices are not always absolute rules, and should be adapted to fit each project’s unique needs and constraints.
Tips for Transitioning to Functional Programming
Transitioning to functional programming can feel overwhelming, but don’t worry – you’re not alone. Here are some tips to guide you through the process:
- Start with the basics: It can be tempting to dive right into complex functional programming concepts, but it’s important to build a solid foundation first. Make sure you understand the core principles of functional programming, such as pure functions and immutability.
- Practice, practice, practice: Like anything in coding, the more you practice, the better you become. Start small by implementing functional programming techniques in simpler projects or specific functions. Once you feel comfortable, gradually incorporate these techniques into larger projects.
- Learn from others: Join online developer communities or attend coding events where you can learn from other functional programming enthusiasts. Ask questions, seek feedback, and collaborate on projects. This can also help you stay motivated and accountable.
- Use the right tools: There are many resources and tools available to help you learn and practice functional programming. Look for online tutorials, textbooks, or video courses that cater to your learning style. Additionally, consider using functional programming languages or frameworks that support your development goals.
Remember that transitioning to functional programming is a journey, not a destination. Be patient with yourself and take your time to fully grasp the concepts and techniques. With consistency and persistence, you’ll soon be on your way to mastering functional programming.
Conclusion
Mastering functional programming is essential for modern software development. By understanding the core concepts of functional programming, developers can write more efficient and scalable code. The benefits of functional programming, such as improved readability, maintainability, and scalability, make it a valuable addition to any coder’s toolkit.
While functional programming may seem daunting at first, there are many resources and tools available to help developers transition to this paradigm. Embracing functional programming principles and best practices can lead to cleaner, more modular code and optimize software development.
Whether you are a seasoned programmer or just starting, incorporating functional programming techniques can enhance your coding abilities and lead to better software development. So why not start exploring and implementing functional programming concepts today?
FAQ
Q: What is functional programming?
A: Functional programming is a programming paradigm that emphasizes immutability, pure functions, and higher-order functions. It focuses on the evaluation of functions and avoids changing state or mutable data.
Q: How does functional programming differ from imperative programming?
A: Functional programming differs from imperative programming in that it avoids mutable data and focuses on the evaluation of functions. Imperative programming, on the other hand, is centered around changing the program’s state through a series of commands or instructions.
Q: What are the benefits of functional programming?
A: Functional programming offers various benefits, including improved code readability, better maintainability, enhanced scalability, and easier parallelization. It can also lead to more efficient and bug-free code.
Q: What are some popular functional programming languages?
A: Popular functional programming languages include Haskell, Scala, Clojure, and Elm. These languages provide built-in support for functional programming concepts and are widely used in the development community.
Q: How can I transition from imperative programming to functional programming?
A: Transitioning to functional programming can be facilitated by understanding the core concepts, practicing functional programming techniques, and utilizing available learning resources. It is recommended to start with small projects and gradually incorporate functional programming principles into your coding practices.