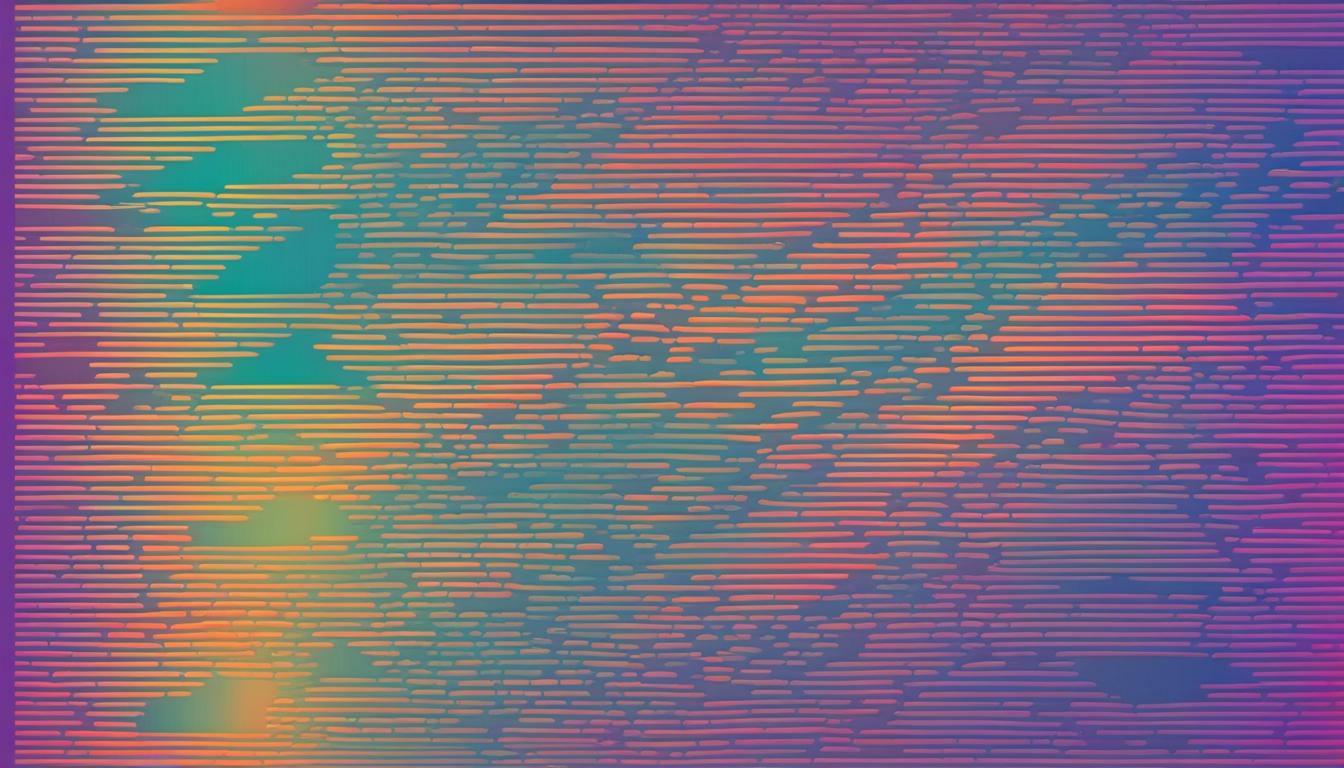
If you’re an aspiring programmer just starting out with Python, you may find yourself struggling to write clean, efficient code. Fortunately, there are plenty of Python code snippets available online that can help you get started.
Python code snippets are small pieces of code that perform specific tasks in Python programming. They are often used as examples of how to use different Python functions and modules, and can be a great way to learn new coding techniques.
In this article, we’ll take a look at some of the most useful Python code snippets for beginners, as well as some more advanced snippets for those looking to take their Python programming to the next level.
Whether you’re just starting out with programming or you’re looking to improve your Python skills, these Python code snippets will help you get the job done.
Python Code Snippets for Basic Syntax
Learning Python syntax is crucial for any aspiring programmer. Here are some Python code snippets to help you master the basics.
Print Statements
The print function is used to display output to the console. Here’s a Python code snippet to display a simple message:
Code | Output |
---|---|
print(“Hello, World!”) | Hello, World! |
You can also use variables in print statements:
Code | Output |
---|---|
name = “Alice” print(“Hello, ” + name + “!”) | Hello, Alice! |
Variable Declaration
Python variables don’t need to be declared with a specific data type. Here’s a Python code snippet to assign a value to a variable:
Code | Output |
---|---|
x = 5 print(x) | 5 |
You can also assign multiple variables on the same line:
Code | Output |
---|---|
x, y, z = “apple”, “banana”, “cherry” print(x) print(y) print(z) | apple banana cherry |
Conditionals
Python uses if/else statements for conditional logic. Here’s a Python code snippet to check if a number is positive or negative:
Code | Output |
---|---|
num = -5 if num < 0: print(“Negative number”) else: print(“Positive number”) | Negative number |
Loops
Python uses for and while loops for iteration. Here’s a Python code snippet to print the numbers 1 to 5:
Code | Output |
---|---|
for i in range(1,6): print(i) | 1 2 3 4 5 |
With these Python code snippets, you can practice and master the basic syntax of Python programming.
Intermediate Python Code Snippets
As an aspiring Python programmer, you may find yourself exploring the vast library of Python modules and packages. Knowing the right tricks and utilizing the available shortcuts can help you become a more efficient developer. Here are some intermediate Python code snippets and tricks that can help you level up your programming game.
1. List Comprehensions
One of the most useful and time-saving features of Python is list comprehensions. These are a concise way to create lists based on an existing list. The new list is generated by applying a given expression to each element of the original list. Here’s an example:
Code Snippet | Output |
---|---|
numbers = [1, 2, 3, 4, 5] | |
squares = [num ** 2 for num in numbers] | [1, 4, 9, 16, 25] |
In this example, we’ve created a new list called squares
by squaring each element of the original list called numbers
. This can replace several lines of code, making your code more concise and readable.
2. Lambda Functions
Lambda functions are anonymous functions that can be defined in a single line of code. They can be useful when you need to pass a small function as an argument to another function. Here’s an example:
Code Snippet | Output |
---|---|
add_numbers = lambda x, y: x + y | |
add_numbers(2, 3) | 5 |
In this example, we’ve defined a lambda function called add_numbers
that takes two arguments and returns their sum. We’ve then called this function with the arguments 2
and 3
.
3. Decorators
Decorators are functions that modify the behavior of another function. They can be used to add functionality to an existing function without changing its code. Here’s an example:
Code Snippet | Output |
---|---|
def decorator_function(original_function): | |
display() | Wrapper function executed this before 'display' function. |
In this example, we’ve defined a decorator function called decorator_function
that takes an original function as an argument and returns a new function called wrapper_function
. The new function adds some functionality to the original function and returns its output. We’ve then used the @decorator_function
syntax to apply this decorator to the display
function.
4. Context Managers
Context managers are used to allocate and release resources automatically. They can be used to simplify your code and ensure that resources are released correctly, even in the case of exceptions. Here’s an example:
Code Snippet | Output |
---|---|
with open('file.txt', 'r') as file: | Contents of file.txt |
In this example, we’ve used a context manager to open a file called file.txt
in read mode and store its contents in a variable called data
. The context manager ensures that the file is closed automatically, even if an exception is raised.
Python Code Snippets for Scripting
If you are looking to automate tedious and repetitive tasks, then Python scripting can be an excellent option. Here are some useful Python code snippets for scripting, which can make your work more efficient and help you save time.
1. Reading and Writing Files
One of the most common tasks in scripting is reading and writing files. The following Python code snippet can be used to read a file:
Code | Description |
---|---|
with open('file.txt', 'r') as file: | Opens a file called “file.txt” in read mode and assigns it to the file variable |
content = file.read() | Reads the entire contents of the file and assigns it to the content variable |
To write to a file, you can use the following Python code snippet:
Code | Description |
---|---|
with open('file.txt', 'w') as file: | Opens a file called “file.txt” in write mode and assigns it to the file variable |
file.write('Hello, World!') | Writes the string “Hello, World!” to the file |
2. Command Line Arguments
Another useful feature in scripting is the ability to accept command line arguments. The following Python code snippet demonstrates how to do this:
Code | Description |
---|---|
import sys | Imports the sys module, which provides access to system-specific parameters and functions |
args = sys.argv | Assigns the command line arguments to the args variable |
You can then use the args
variable to access the command line arguments. For example, if you run the script with the command python script.py arg1 arg2
, then args
will be a list containing ['script.py', 'arg1', 'arg2']
.
3. Regular Expressions
Regular expressions can be extremely useful for manipulating and searching text data. The following Python code snippet demonstrates how to use regular expressions:
Code | Description |
---|---|
import re | Imports the re module, which provides support for regular expressions |
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$' | Defines a regular expression pattern for matching email addresses |
result = re.match(pattern, email) | Applies the regular expression pattern to the email variable and returns a match object if there is a match |
In this example, the regular expression pattern matches a valid email address. You can use regular expressions to perform complex text manipulations and validations.
These Python code snippets for scripting are just a few examples of how Python can be used to automate tasks and make your work more efficient. With a little practice, you can become a master of Python scripting and save yourself countless hours of manual work.
Advanced Python Code Snippets
For experienced Python programmers, there are many shortcuts and coding tricks that can save time and make code more efficient. Here are some advanced Python code snippets to help take your programming to the next level:
1. List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. For example, to create a new list with the squares of the numbers in an existing list:
new_list = [x**2 for x in old_list]
2. Lambda Functions
Lambda functions are small, anonymous functions that can be defined in a single line of code. They are particularly useful for passing as arguments to higher order functions such as map() and filter(). For example, to create a lambda function that squares a number:
square = lambda x: x**2
3. Decorators
Decorators are a way to modify the behavior of a function without changing its source code. They are often used for tasks such as logging and timing. For example, to create a decorator that logs the time a function takes to run:
import time
def timer(func):
def wrapper(*args, **kwargs):
t1 = time.time()
result = func(*args, **kwargs)
t2 = time.time()
print(f”{func.__name__} took {t2-t1} seconds to run”)
return result
return wrapper@timer
def my_function():
# function code here
4. Context Managers
Context managers are a way to manage resources such as files and network connections in a more efficient and safe manner. The with statement is used to define a context manager. For example, to define a context manager that opens and closes a file:
class File:
def __init__(self, filename, mode):
self.filename = filename
self.mode = modedef __enter__(self):
self.file = open(self.filename, self.mode)
return self.filedef __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()with File(‘example.txt’, ‘w’) as f:
f.write(‘Hello, world!’)
5. Threading
Threading is a way to run multiple threads (or tasks) simultaneously within a single program. This can improve performance and responsiveness. For example, to define a function that runs in a separate thread:
import threading
def my_function():
# function code here
t = threading.Thread(target=my_function)
t.start()
These are just a few examples of the many advanced Python code snippets available to experienced programmers. By incorporating these snippets into your code, you can streamline your workflow and improve the efficiency of your programs.
Conclusion:
Python code snippets are a powerful tool that every aspiring programmer should consider learning. With code snippets, you can simplify complex coding procedures and reduce your workload. The snippets can be used to write basic syntax, intermediate code, scripting and advanced features.
Beyond the snippets, Python programming is a great skill to have, as it is one of the most popular programming languages in the world. Therefore, mastering Python will give you an edge in the job market.
Final Thoughts
In conclusion, learning Python code snippets is an effective way to improve your programming skills, increase your productivity and save you time. Continuously practicing coding with snippets will help you to become a better programmer and enhance your understanding of Python programming. Keep practicing and experimenting with the snippets until you become an expert in using them.
Thank you for reading this article on useful Python code snippets for aspiring programmers. We hope you found it useful and informative. Happy coding!