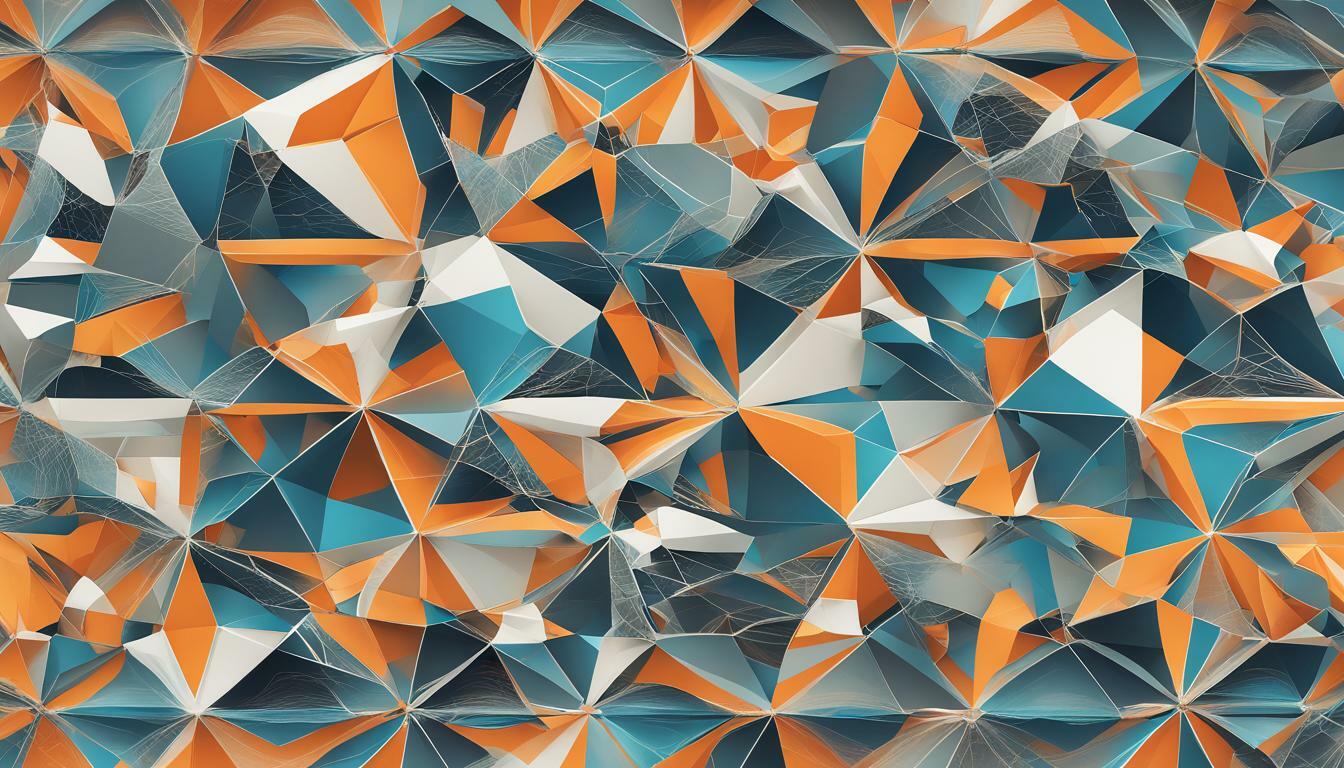
As the world becomes more and more digital, APIs are becoming a crucial part of modern software development. APIs are the backbone of many applications, providing a way for different systems to communicate with each other. However, APIs are only as good as their performance, security, and maintainability. This is where .NET API Craftsmanship comes in.
In this article, we will explore the importance of .NET API Craftsmanship, with a specific focus on performance, security, and maintainability. We will provide readers with practical examples and best practices to help them enhance their API development skills. By the end of this article, readers will have a better understanding of how to develop efficient, secure, and maintainable APIs in .NET.
If you are a software developer looking to improve your API development skills, or if you are new to API development and want to learn more, then this article is for you. So, let’s dive into the wonderful world of .NET API Craftsmanship!
Throughout the article, we will use relevant keywords such as .NET API Craftsmanship, Performance, Security, Maintainability, and Examples to help readers quickly identify the topics covered.
Enhancing Performance in .NET API Development
Developing high-performance APIs is crucial for ensuring a great user experience and efficient resource utilization. In this section, we will discuss the best practices for enhancing performance in .NET API development.
Code Optimization
Code optimization is a crucial step in enhancing API performance. It involves identifying and eliminating any bottlenecks that can slow down your API. One way to optimize your code is by minimizing the number of database queries. This can be achieved by using caching to store frequently accessed data. Another way is by minimizing the use of loops and replacing them with more efficient code constructs.
Efficient Coding Practices
Using efficient coding practices can help improve the performance of your API. One way is by using asynchronous programming wherever possible. Asynchronous programming can help improve scalability and performance by allowing the API to handle multiple requests simultaneously. Another way is by minimizing the use of external libraries and frameworks, as they can add unnecessary overhead to your API.
Performance Testing
Performance testing is critical in ensuring that your API meets the required performance standards. It involves testing the API’s response time, throughput, and resource utilization. Performance testing can help you identify and isolate performance issues, enabling you to optimize your API for better performance.
By following the above practices, you can optimize your code, improve your API’s performance, and deliver a great user experience.
Ensuring Security in .NET API Craftsmanship
When it comes to API development, security should always be a top priority. An API security breach can have devastating consequences, from unauthorized access to sensitive data to compromised user accounts. Therefore, ensuring a secure API is crucial.
One important aspect of API security is secure coding practices. This involves implementing measures such as input validation, user authentication and authorization, and protection against common security threats, such as cross-site scripting (XSS) and SQL injection attacks.
Secure Coding Practices | Description |
---|---|
Input Validation | Input validation is the process of checking user input to ensure that it is safe and conforms to expected formats. This helps prevent common security vulnerabilities such as buffer overflow and injection attacks. |
User Authentication and Authorization | Authentication is the process of verifying that a user is who they claim to be, while authorization is the process of determining what rights and privileges a user has. Implementing strong authentication and authorization mechanisms can help prevent unauthorized access to sensitive data. |
Protection Against Common Security Threats | Common security threats such as cross-site scripting (XSS) and SQL injection attacks can be prevented through proper input validation and other secure coding practices. |
Another important aspect of API security is staying up to date with the latest security threats and vulnerabilities. This involves regularly reviewing and testing your API for potential vulnerabilities and patching any security holes that are discovered.
By focusing on security in your .NET API Craftsmanship, you can help prevent security breaches and protect your users’ sensitive data. Don’t neglect this crucial aspect of API development.
Maintaining .NET APIs for Long-Term Success
One of the most critical aspects of API development is maintainability. Ensuring that your code is easy to read, modify, and scale over time is vital for the long-term success of your API. This section will cover some efficient coding practices and strategies to improve API maintainability.
Code Organization
Organizing your code in a logical and consistent way can make it easier to navigate and understand. Consider breaking up your code into logical modules or components, grouping related functions, and ensuring proper naming conventions. Following a consistent code structure can make it easier for developers to jump in and make changes or additions to the API.
Documentation
Documenting your code is essential for maintaining its readability and ensuring that future developers can understand its functionality. Consider using comments to describe what each line or block of code does, and include documentation for any API endpoints or data structures. Providing clear and comprehensive documentation can make it easier for new developers to work with your API and can save time and effort in the long run.
Versioning
As your API evolves and new features are added, it’s important to maintain backward compatibility with existing clients. Versioning your API can help ensure that changes to the API don’t break existing client code. Consider using a clear and consistent versioning scheme, such as Semantic Versioning, to communicate changes to clients and make it easier for them to update their code accordingly.
Handling Changes and Updates
Finally, it’s important to have a strategy in place for handling changes and updates to your API. This may involve using version control software like Git, setting up automated testing and deployment pipelines, and having a clear process for reviewing and approving changes. By having a well-defined process in place, you can ensure that changes to your API are well-managed and don’t introduce unintended bugs or issues.
By following these best practices for improving code maintainability, you can help ensure the long-term success of your .NET API. By making your code easy to read, modify, and scale, you can save time and effort down the line and make it easier for other developers to work with your code.
Practical Examples of .NET API Craftsmanship
Let’s take a look at some practical examples of .NET API Craftsmanship. These examples will showcase the concepts and techniques discussed in the previous sections, and provide you with a better understanding of how to implement best practices in your own API development projects.
Example 1: Optimizing API Performance
One common performance issue in API development is the overuse of database calls. In this example, we’ll demonstrate how to optimize API performance by reducing the number of database calls.
Non-optimized code: | Optimized code: |
---|---|
|
|
In the non-optimized code, the API makes separate database calls for each order’s customer and products, resulting in poor performance when dealing with large amounts of data. In the optimized code, the API uses the .Include() method to optimize the query and retrieve all the necessary data in a single database call, significantly improving performance.
Example 2: Implementing API Security
Security is a crucial aspect of API development, and in this example, we’ll demonstrate how to implement secure APIs by using proper authentication and authorization techniques.
Insecure code: | Secure code: |
---|---|
|
|
In the insecure code, the API accepts a username and password as parameters and performs a simple database lookup to validate the user’s credentials. This approach is vulnerable to SQL injection attacks and password cracking. In the secure code, the API uses password hashing and salting techniques to securely store user passwords in the database, and verifies the password against the hashed version. This provides a much more secure API.
Example 3: Improving API Maintainability
Maintainability is key to the long-term success of any API. In this example, we’ll demonstrate how to improve API maintainability by organizing code into logical units and properly documenting it.
Unorganized code: | Organized code: |
---|---|
|
|
In the unorganized code, all the methods are implemented in a single class, making it difficult to navigate and maintain the code as it grows. In the organized code, the code is organized into logical units using attributes, making it easier to navigate and maintain the code as it grows. Additionally, the constructor is used to inject the required dependencies, and the code is properly documented.
These examples demonstrate how to implement best practices in .NET API Craftsmanship. By following these techniques and strategies, you can create high-performing, secure, and maintainable APIs that will stand the test of time.
Conclusion
In conclusion, .NET API Craftsmanship is a crucial aspect of API development that should focus on performance, security, and maintainability. By implementing efficient coding practices, conducting performance testing, and prioritizing security measures like authentication and authorization, input validation, and protection against common security threats, developers can create secure and reliable APIs that are easy to maintain for the long-term.
Apply Best Practices
By applying the best practices discussed in this article, developers can ensure that their APIs not only meet the needs of their users but also remain relevant and effective in the future. Remember to prioritize performance, security, and maintainability, and to continuously monitor and optimize your code to ensure it is running at peak efficiency.
Keep Learning
With new technologies and best practices emerging all the time, it’s important to keep learning and staying up-to-date with the latest developments in API Craftsmanship. By reading articles, attending workshops and conferences, and engaging with the wider API development community, you can continue to fine-tune your skills and stay ahead of the curve.
Thank you for reading, and happy crafting!