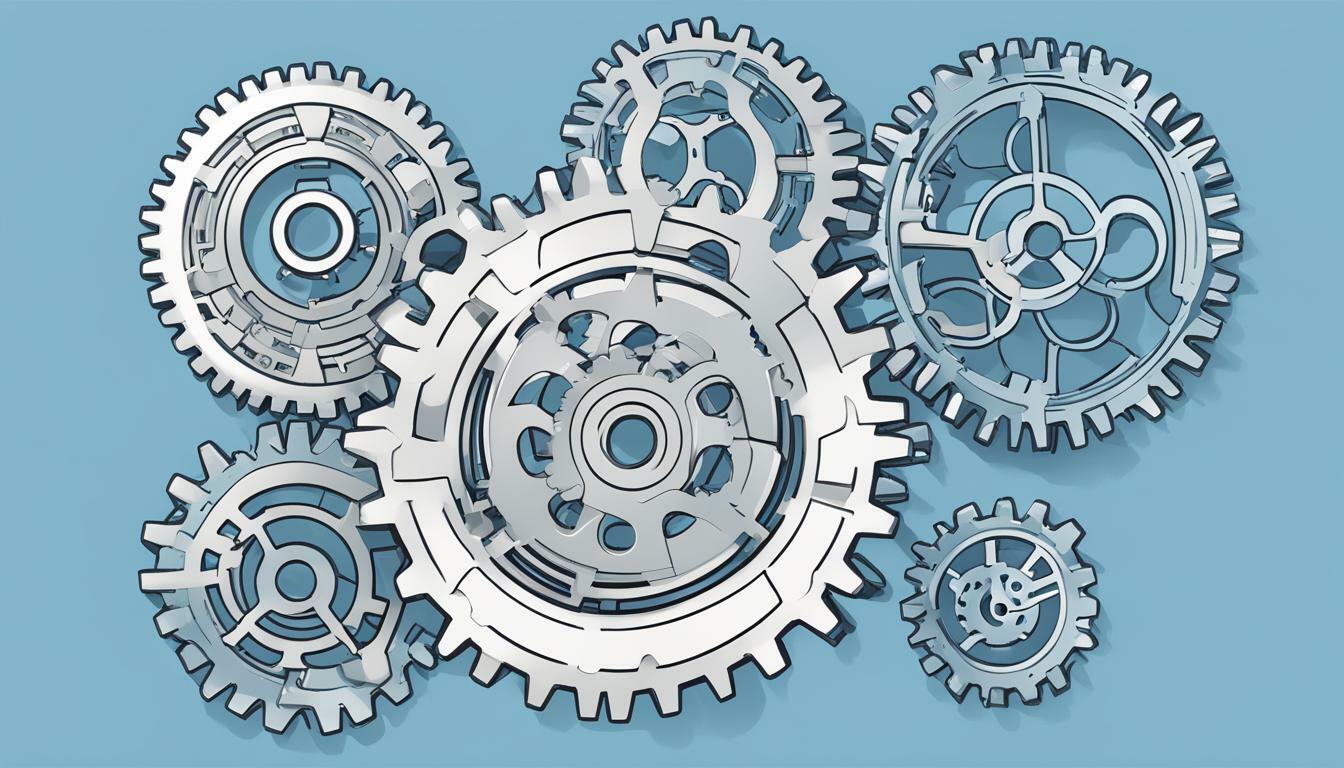
In software development, repeating oneself can be costly and time-consuming. The concept of DRY, or Don’t Repeat Yourself, is a principle that emphasizes the importance of reusability in creating efficient and clean code. By avoiding code duplication, developers can save time, reduce errors, and simplify maintenance.
DRY is a fundamental principle in software development that promotes code efficiency and best practices. It ensures that code is reusable, modular, and scalable, making it easier to maintain and update in the long run. By adhering to DRY principles, developers can optimize their software development processes and achieve better outcomes.
Key Takeaways:
- DRY is a principle that emphasizes the importance of reusability in software development.
- By avoiding code duplication, developers can save time and reduce errors.
- DRY ensures that code is reusable, modular, and scalable, making it easier to maintain and update.
- Adhering to DRY principles can optimize software development processes and achieve better outcomes.
Understanding DRY: Eliminating Redundancy in Code
Don’t Repeat Yourself (DRY) is a programming principle that revolves around code reusability, efficiency, and maintainability. In essence, DRY advocates for avoiding code duplication by consolidating common logic, functions, and routines into reusable components.
When developers repeat the same code block multiple times, it leads to bloated, unmaintainable codebases. With time, the code becomes redundant, error-prone, and difficult to modify or update. The more code duplication there is, the harder it is to fix bugs, add new features, or refactor the codebase.
Therefore, adhering to DRY principles ensures that the code remains clean, concise, and easy to maintain. By consolidating common pieces of code, developers can save time and energy, avoid errors, and enhance the performance of the application.
Benefits of DRY: Code Efficiency and Clean Code
DRY goes beyond code reusability; it also promotes code efficiency and clean code. By keeping the codebase free of redundancies, it minimizes coupling and increases cohesion. With fewer dependencies between classes and modules, the code is easier to comprehend, maintain, and test.
Furthermore, adhering to DRY principles helps to boost code performance and scalability. When the code is modular, and each module performs a specific task or function, it becomes easier to optimize and scale the application. This translates to faster software development cycles, fewer bugs, and optimized resource utilization.
By promoting clean code, DRY principles also make it easier for developers to collaborate and work on projects seamlessly. Consistent, concise code makes it easy to read and understand the codebase, making it easier to onboard new developers into a project.
Building Blocks: Modular Programming for Reusability
One of the fundamental principles of DRY is the use of modular programming to achieve code reusability. Modular programming involves breaking down code into smaller, reusable modules or functions, which can be called upon multiple times throughout the code. This technique not only saves time and effort but also makes the code easier to maintain and update.
The key to successful modular programming lies in designing modules that are cohesive, loosely coupled, and highly cohesive. Cohesiveness means that a module should have a single, specific purpose, and everything within it should work towards achieving that purpose. Loose coupling, on the other hand, refers to modules that are not dependent on other modules. Highly cohesive modules are those that have a clear and consistent function.
To create modules that exhibit these qualities, developers should follow best practices such as avoiding global variables, minimizing side effects, and keeping functions short and concise. Additionally, naming conventions can help clarify the purpose of each module and improve the readability of the code.
Modular programming also enables code testing, as individual modules can be tested independently of the rest of the code, making it easier to identify and fix errors.
Benefits of Modular Programming
Utilizing modules in code development has several advantages, including:
- Code Reusability: Modules can be called upon multiple times throughout the code, saving time and effort.
- Improved Maintenance: Modular code is easier to maintain and update, as changes can be made to a single module rather than throughout the entire codebase.
- Code Testing: Individual modules can be tested independently, making it easier to identify and fix errors.
Best Practices for Modular Programming
When it comes to designing modules, following these best practices will help create code that is more efficient, reusable, and easier to maintain:
- Avoid Global Variables: Global variables can create dependencies between modules and make it difficult to track changes throughout the code.
- Minimize Side Effects: Side effects can create unpredictable results when modules are called upon multiple times throughout the code.
- Keep Functions Short and Concise: Short and concise functions are easier to test and understand, improving code readability and maintainability.
Following these best practices and designing cohesive, loosely coupled, and highly cohesive modules can help developers achieve efficient and reusable code, making DRY principles easier to implement and maintain.
Implementing DRY: Tools and Techniques
Implementing DRY principles in software development requires tools and techniques that aid in achieving reusability and maintaining code efficiency. The following are some practical strategies that can be used:
Code Refactoring
Code refactoring involves restructuring existing code to enhance its readability, maintainability, and extensibility. It eliminates redundancies, improves code clarity, and simplifies logic. It is an effective way of improving code reusability.
“Refactoring is the process of changing a software system in such a way that it does not alter the external behavior of the code yet improves its internal structure. It is a disciplined way to clean up code that minimizes the chances of introducing bugs.” – Martin Fowler
Abstraction
Abstraction involves identifying common features and behaviors among objects or functions and creating a generalized representation of them. It simplifies code by hiding complex details and exposing only the essential features. It enhances code reusability by reducing the need for repetitive code.
Design Patterns
Design patterns are reusable solutions to common problems in software development. They provide a standard framework for creating efficient, scalable, and maintainable code. They enhance code reusability by providing pre-tested, well-documented solutions to common programming problems.
Unit Testing
Unit testing involves testing individual units or modules of code in isolation to ensure their correctness, reliability, and functionality. It is an effective way of identifying and fixing bugs before they cause bigger problems. It enhances code reusability by ensuring that code modules are independent and can be reused without introducing unintended side effects.
By implementing these tools and techniques, developers can create efficient, scalable, and maintainable code that adheres to DRY principles. They can reduce redundancy, minimize code complexity, and enhance code reusability.
Overcoming Challenges: Maintaining DRY Code
While implementing DRY principles can lead to more efficient and reusable code, maintaining DRY codebases can come with its own set of challenges. Here are some common challenges and best practices for handling them:
Handling Dependencies
Creating reusable code modules often involves dependencies. As code changes over time, it’s essential to keep track of dependencies to avoid breaking the code. One way to handle dependencies is by using dependency management tools such as Maven or Gradle. These tools can help manage dependencies and ensure that the codebase remains stable.
Versioning
Versioning is crucial when maintaining DRY codebases. Any change to a reusable code module can have adverse effects on dependent modules. Using a version control system like Git allows developers to manage code changes and track version history effectively. It’s essential to avoid breaking changes to modules without first incrementing the version number.
Code Updates
Updating reusable code modules can be a challenge. While updating a module may fix bugs, it can also break dependent modules. The best practice is to test the updates thoroughly before rolling them out. Testing can help ensure that the updated code is compatible with the dependent modules and that the codebase remains stable.
Documentation and collaboration are also essential when maintaining DRY codebases. Developers should communicate changes to dependent modules and document the changes to avoid confusion and ensure a better understanding of the codebase.
Case Studies: Real-world Examples of DRY in Action
DRY principles have been implemented successfully in various software development projects, resulting in significant improvements in code efficiency and reusability. Let’s explore some real-world examples of how DRY has impacted software development practices.
Case Study 1: Airbnb
Airbnb, the popular online marketplace for lodging and vacation rentals, implemented DRY principles to improve the efficiency of their codebase. By breaking down code into reusable components and modules, Airbnb was able to reduce the number of lines of code and enhance its maintainability. The company also leveraged open-source libraries to promote code reusability and collaboration across different teams. This resulted in faster development cycles and more reliable software releases.
Case Study 2: Netflix
Netflix, the world’s leading streaming service, also actively embraces DRY principles in its software development practices. By breaking down its codebase into smaller, reusable components, Netflix has been able to reduce duplication and promote clean code. The company also invests in tools and technologies that optimize code reuse, such as React, a popular Javascript library that enables developers to build reusable UI components. Netflix reports significant improvements in development efficiency and scalability as a result of adopting DRY principles.
Case Study 3: Google
Google, one of the world’s largest tech companies, has a long history of using modular programming and code reuse in its software development practices. The company’s codebase is broken down into reusable modules and libraries that are shared across different teams and products. Google also invests in creating and maintaining open-source libraries that promote code reusability and collaboration among developers. By embracing DRY principles, Google is able to optimize code efficiency and reduce maintenance costs.
Case Study 4: Shopify
Shopify, the popular e-commerce platform, has made significant efforts to optimize code reusability and efficiency in its software development practices. The company invests in maintaining a library of reusable components that developers can easily integrate into their codebase. Shopify also promotes the use of design patterns and modular programming to enhance code efficiency and scalability. As a result, the company has reported faster development cycles and fewer issues related to code duplication and maintenance.
The Future of Reusability: Advancements and Trends
The software development industry is constantly evolving, and new advancements and trends are emerging to enhance code reusability and efficiency. As developers continue to embrace and implement DRY principles, new technologies are being developed to simplify the process and make it even more effective.
Emerging Technologies
One of the most significant advancements in software development is the rise of containerization technology. Containers enable developers to package and deploy applications in a portable and efficient way, making it easier to build and maintain reusable codebases. Furthermore, serverless computing and function-as-a-service (FaaS) architectures are gaining popularity as they allow developers to focus on writing the code logic rather than deploying and managing infrastructure.
New Frameworks and Practices
Modern front-end frameworks like React, Angular, and Vue.js have made it easier to build modular and reusable user interfaces. These frameworks allow developers to break down UI components into smaller and more manageable pieces, which can be reused across different projects. Additionally, the adoption of agile development methodologies and continuous integration/continuous delivery (CI/CD) pipelines has made it easier to maintain and deploy reusable code.
The Importance of Collaboration
As software development becomes more complex, collaboration between developers and other stakeholders is becoming increasingly important. Tools like GitHub enable developers to work collaboratively on code, allowing them to share and reuse codebases more easily. Additionally, the adoption of DevOps practices has helped bridge the gap between development and operations teams, fostering a culture of collaboration and efficiency.
As the software development industry continues to evolve, there is no doubt that reusability and DRY principles will remain central to creating efficient and maintainable code. By staying up-to-date with emerging technologies, frameworks, and best practices, developers can optimize their workflows and achieve greater productivity.
Conclusion
In conclusion, DRY (Don’t Repeat Yourself) is a powerful principle that promotes reusability and efficiency in software development. By eliminating redundancy and creating modular code structures, developers can improve productivity and maintainability.
Adhering to DRY principles and best practices, such as modular programming and code refactoring, can enhance the quality and cleanliness of code. However, maintaining DRY codebases can be a challenge, especially with dependencies, versioning, and updates.
Documenting and collaborating with team members are essential to maintaining the effectiveness of DRY code.
Real-world examples demonstrate the significant impact of DRY on productivity, maintenance, and scalability. With advancements in emerging technologies, frameworks, and practices, the future holds exciting opportunities for enhancing software reusability even further.
In summary, DRY is a fundamental principle in software development that promotes code efficiency, cleanliness, and reusability. It is essential to embrace this principle and stay updated with emerging trends and advancements to optimize software development processes continually.
FAQ
Q: What is DRY (Don’t Repeat Yourself)?
A: DRY, or Don’t Repeat Yourself, is a software development principle that emphasizes the importance of reusability in code. It promotes the idea of avoiding redundancy by creating modular and reusable code structures.
Q: Why is software reusability important?
A: Software reusability is crucial because it allows developers to save time and effort by leveraging existing code. It promotes code efficiency, reduces maintenance challenges, and enhances the overall quality of software development.
Q: How does DRY contribute to clean code?
A: DRY helps in achieving clean code by eliminating duplicate code segments and promoting a modular and organized code structure. It makes the code easier to understand, maintain, and collaborate on.
Q: What are some best practices for implementing DRY?
A: Some best practices for implementing DRY include creating reusable modules and components, using code refactoring techniques, leveraging abstraction and design patterns, and documenting dependencies and code updates.
Q: What are the common challenges in maintaining DRY code?
A: Some common challenges in maintaining DRY code include handling dependencies, managing versioning, and ensuring proper documentation. Collaboration and communication are also vital to ensure the longevity and effectiveness of DRY codebases.
Q: Can you provide real-world examples of DRY in action?
A: Sure! Real-world examples of DRY include using libraries or frameworks for common functionalities, creating reusable components in web development, and implementing design patterns to solve recurring problems in software projects.
Q: What are some emerging advancements and trends in software development for reusability?
A: Emerging advancements and trends in software development for reusability include the rise of low-code and no-code platforms, the adoption of microservices architecture, and the growing popularity of serverless computing. These technologies promote more efficient and reusable code practices.
Q: Why should developers adopt DRY as a fundamental principle?
A: Developers should adopt DRY as a fundamental principle because it enhances code efficiency, improves productivity, and promotes best practices in software development. By embracing DRY, developers can create cleaner, more maintainable, and scalable codebases.