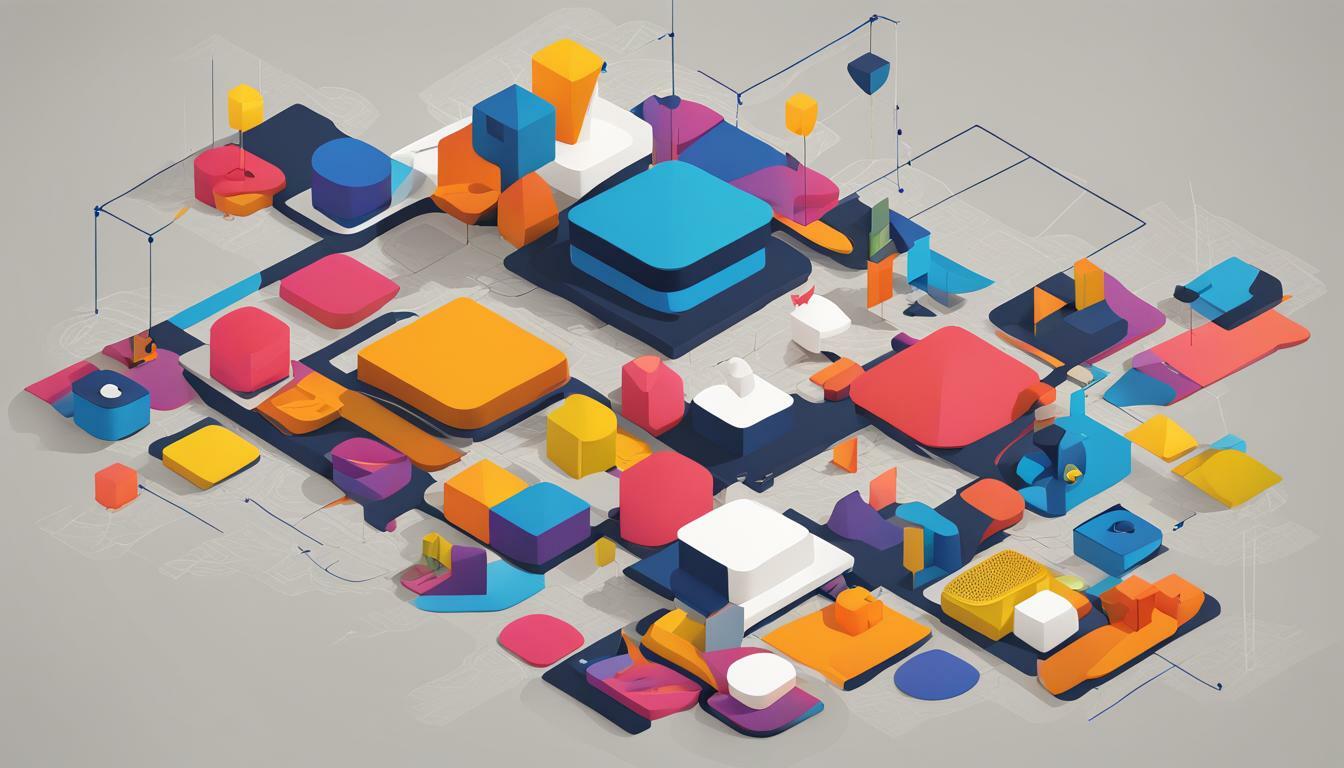
In the world of software development, designers are always looking for ways to simplify coding and create more efficient systems. The Command Pattern is one such tool that allows developers to encapsulate requests as objects, resulting in greater flexibility and scalability in their code. By decoupling the sender of a request from its receiver, the Command Pattern creates a more modular and extensible design.
In this section, we will explore the concept of the Command Pattern in depth, examining its benefits and discussing practical tips for implementing it in software projects. By understanding this pattern and its potential impact, developers can improve their coding skills and create more sophisticated applications.
Key Takeaways
- The Command Pattern encapsulates requests as objects in software development.
- Decoupling the sender of a request from its receiver creates a more flexible and extensible design.
- The Command Pattern simplifies coding and enhances the reusability of code.
Understanding the Command Pattern
As one of the most valuable software design patterns, the Command Pattern is an essential tool for any developer interested in object-oriented design. Essentially, this pattern involves encapsulating requests as objects, creating a more flexible and extensible system.
The Command Pattern is a popular choice for developers who want to decouple the sender of a request from its receiver, making it easier to manage requests and implement changes in the future. By transforming requests into objects, the code becomes more reusable and easier to maintain.
At its core, the Command Pattern is all about separating the responsibilities of different components in a software application. In particular, it helps to reduce coupling between objects, promoting a more modular design.
While the Command Pattern can provide several benefits, it is important to understand how to implement it effectively. When used correctly, this pattern can improve the quality, scalability, and maintainability of software projects.
Command Pattern Explanation
The Command Pattern is a software design pattern that allows developers to encapsulate requests as objects, making it easier to manage and modify requests in the future. Essentially, this pattern involves creating a “command” object that contains all the necessary information to execute a particular request. This object can then be passed between different components of the system, allowing for a more flexible implementation.
The Command Pattern is a valuable tool for developers who want to reduce coupling between objects in their software applications. By encapsulating requests, this pattern allows developers to modify the behavior of their code without changing the underlying structure of the software.
Object-Oriented Design
The Command Pattern is a key component of object-oriented design. As an essential tool for promoting modularity, it helps developers to create software applications that are easier to scale and maintain. By separating the concerns of different components, developers can create a more flexible and extensible system.
Overall, the Command Pattern is a valuable software design pattern that can help developers to create more maintainable, scalable, and flexible software applications. By understanding the fundamental principles of this pattern and its benefits, developers can take advantage of its power and enhance their code quality.
Encapsulating Requests as Objects
In the Command Pattern, encapsulating requests as objects is a crucial aspect that enhances code reusability, flexibility, and maintainability. When implementing this pattern, developers create specific objects that represent individual requests or commands. These objects contain all the necessary information and parameters required to execute the command.
By encapsulating requests as objects, developers can separate the logic for executing a command from the logic for creating it. This separation allows for greater flexibility in modifying the behavior of the command, without impacting the code that uses it. Furthermore, by encapsulating requests, developers can easily create new commands by creating new objects that implement the required interface.
Implementing the encapsulation of requests in the Command Pattern involves creating a command interface or abstract class that defines the contract for executing commands. Developers can then create concrete implementations of this interface or abstract class to represent individual commands. Each command object contains the necessary data to execute its specific command, including references to the object that will execute it – the receiver – and the method that performs the action.
Component | Description |
---|---|
Command Interface/Abstract Class | The contract that defines the execute() method, which encapsulates the logic for executing the command. |
Concrete Command Classes | Concrete implementations of the command interface/abstract class, which contain all the necessary data to execute a specific command. |
Receiver | The object that knows how to execute the command. |
Invoker | The object that holds the command and calls its execute() method. |
Overall, encapsulating requests as objects in the Command Pattern offers many benefits for object-oriented design. By creating distinct objects that encapsulate the behavior of different commands, developers can reduce coupling between objects and improve the organization and modularity of their code. Furthermore, this approach to coding promotes code reuse and makes it easier to create new commands without modifying existing code.
Benefits of the Command Pattern
The Command Pattern offers several benefits when it comes to software design, making it a valuable tool for developers. Let’s explore some of the advantages that come with implementing this pattern.
Improves Code Organization
One of the biggest benefits of the Command Pattern is that it improves code organization and structure. By encapsulating requests as objects, developers can easily group related commands together and keep the codebase clean and organized. This leads to better code clarity and maintainability in the long run.
Enhances Modularity
Another advantage of the Command Pattern is that it enhances modularity in software design. Command objects are self-contained and can be easily added, removed, or modified without affecting other parts of the code. This makes it easier to make changes as the project evolves and reduces the likelihood of introducing bugs into the system.
Promotes Code Reuse
The Command Pattern also promotes code reuse, as command objects can be used across different parts of the system. This means that developers can avoid writing redundant code and instead leverage existing code to accomplish new tasks. This saves time and effort in the development process, leading to faster project delivery and a more efficient workflow overall.
Facilitates Undo/Redo Operations
Finally, the Command Pattern facilitates undo/redo operations in software applications. By keeping track of command objects, developers can easily implement an undo/redo functionality in the system. This can be especially useful in applications that involve user interactions, such as word processors or graphic design tools.
Overall, the Command Pattern is a powerful tool for improving software design and development. By leveraging its benefits, developers can create more maintainable, scalable, and efficient systems that can adapt to changing requirements.
Implementation of the Command Pattern
The Command Pattern is an object-oriented design pattern that encapsulates requests as objects. To effectively implement this pattern, there are specific components that developers need to consider. These components include the command objects, invoker, and receivers.
The command objects are the focal point of the Command Pattern. They encapsulate the request and its relevant parameters. Each command object must have an execute() method that encapsulates the logic of the request.
The invoker is responsible for receiving the command object from the client and initializing it with the appropriate receiver. Once the command object is initialized, the invoker can call its execute() method to execute the request.
The receiver is responsible for performing the actual work of executing the request. The client can create multiple receivers, and each receiver can have a unique implementation of the execute() method.
One of the challenges of implementing the Command Pattern is determining how to pass parameters to the command object. One approach is to pass the parameters as arguments to the execute() method. Another approach is to include the parameters in the command object constructor. The choice of approach depends on the specific requirements of the project.
It is important to note that the Command Pattern can be implemented in various ways. For example, developers can use the Command Pattern with or without undo/redo functionality. They can also choose to use the Command Pattern in combination with other design patterns, such as the Observer Pattern or the Factory Pattern.
Overall, the Command Pattern is a valuable tool for implementing complex requests and decoupling the sender of a request from the receiver. By following best practices and considering the necessary components, developers can successfully implement the Command Pattern in their software projects.
Conclusion
Understanding the Command Pattern is a crucial aspect of Object-Oriented Design and Software Design Patterns. Encapsulating requests as objects provides developers with a flexible and extensible way to decouple the sender of a request from the receiver.
The Command Pattern improves code organization, enhances modularity, and promotes code reuse. By leveraging the benefits of the Command Pattern, developers can create more maintainable and scalable systems.
Implementing the Command Pattern requires understanding its fundamental principles and the necessary components such as command objects, invoker, and receivers. Following best practices and common challenges can help developers successfully apply this pattern in their applications.
In conclusion, by embracing the Command Pattern, developers can simplify coding and create more flexible and maintainable systems. It opens up new possibilities for creating robust and scalable applications.
FAQ
Q: What is the Command Pattern?
A: The Command Pattern is a software design pattern that focuses on encapsulating requests as objects. It provides a way to decouple the sender of a request from the receiver, allowing for a more flexible and extensible design.
Q: Why is the Command Pattern valuable in object-oriented design?
A: The Command Pattern fits into the broader context of object-oriented design by providing a valuable means of encapsulating requests and promoting code reusability. Understanding the core concepts of the Command Pattern can enable developers to implement it effectively in their projects.
Q: What are the benefits of encapsulating requests in the Command Pattern?
A: Encapsulating requests as objects in the Command Pattern offers several benefits, including greater flexibility, maintainability, and testability in code. By transforming requests into objects, developers can simplify the execution of commands and achieve a more modular and scalable system.
Q: How does the Command Pattern improve code organization and modularity?
A: The Command Pattern improves code organization and modularity by separating the sender of a request from the receiver. This separation allows commands to be easily added, modified, or reused without impacting other parts of the system, leading to more maintainable and scalable code.
Q: What are some practical insights for implementing the Command Pattern?
A: When implementing the Command Pattern, it is important to define the necessary components, such as command objects, invokers, and receivers, and understand how they interact with each other. Following best practices and considering common challenges can help ensure a successful implementation of the Command Pattern in software projects.