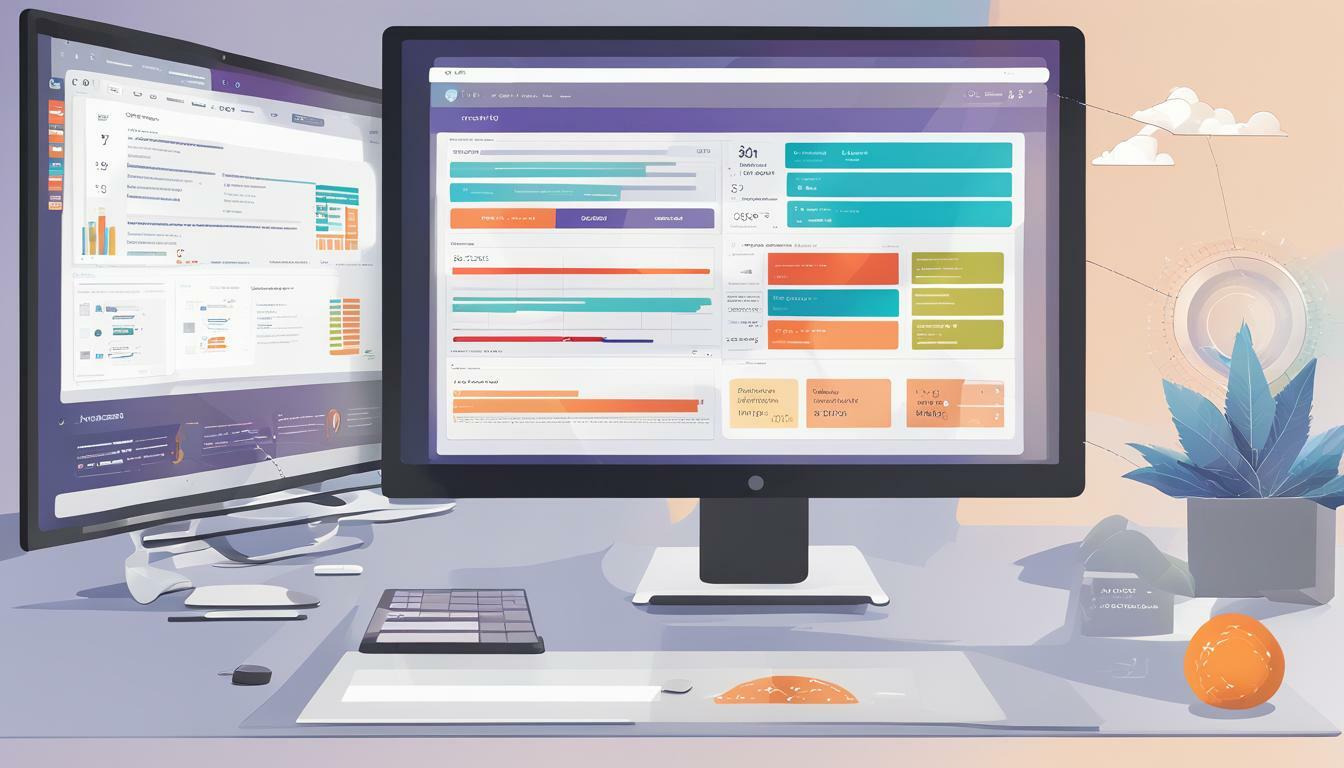
RESTful APIs are an essential aspect of modern web development architecture and .NET Core is the preferred framework for building such APIs. Implementing best practices is key to building efficient and scalable APIs that provide optimal performance. In this article, we will explore the best practices for building RESTful APIs with .NET Core and developing efficient endpoints.
Key Takeaways
- RESTful APIs are an essential aspect of modern web development architecture.
- .NET Core is the preferred framework for building RESTful APIs.
- Implementing best practices is key to building efficient and scalable APIs.
Understanding RESTful API Design Principles
Before diving into building efficient endpoints with .NET Core, it’s crucial to understand the principles behind RESTful API design. REST (Representational State Transfer) is an architectural style that defines a set of constraints to be used when creating web services. It relies on the HTTP protocol for communication and uses a uniform interface that separates the client and server implementation.
At the heart of RESTful architecture is the resource, which is any piece of information that can be identified by a unique URI (Uniform Resource Identifier). Resources are manipulated using a standardized set of HTTP verbs, such as GET, POST, PUT, and DELETE, which map to the CRUD (Create, Read, Update, Delete) operations commonly associated with databases.
When designing RESTful APIs, it’s important to follow certain best practices to ensure consistency, scalability, and maintainability. In .NET Core, this can be achieved by adhering to the SOLID principles and using middleware to handle cross-cutting concerns, such as authentication, caching, and logging.
To achieve a well-designed RESTful API, it’s important to focus on the following aspects:
- Resources: Identify the resources to be exposed by the API and their corresponding URIs. Use nouns instead of verbs and make the URIs hierarchical.
- Representation: Determine the format in which the resources will be represented, typically JSON or XML. Provide metadata about the representation, such as data types and links to related resources.
- Actions: Define the actions that can be performed on each resource using the appropriate HTTP verbs. Handle errors and exceptions consistently.
- Security: Protect the API against unauthorized access by implementing authentication and authorization mechanisms.
- Caching: Use caching to improve performance and reduce network traffic by returning cached responses for unchanged resources.
By following these best practices, you can create well-designed RESTful APIs that are easy to use, scalable, and maintainable. In the next section, we’ll see how to implement efficient endpoints using .NET Core.
Implementing Efficient API Endpoints with .NET Core
Building efficient endpoints is crucial to creating a successful RESTful API. To achieve this, it is essential to follow several .NET Core best practices and web API best practices.
Choose the Right Framework
The .NET Core provides various tools and libraries that offer developers flexibility and speed in building APIs. By choosing the appropriate framework, developers can simplify the development process and facilitate maintenance. Ensure the framework offers a range of features that support efficient API endpoint building and maintenance.
Use Caching
Using caching is a common technique developers use to improve API performance by reducing network traffic and response times. Caching stores frequently requested API responses in memory for faster access, providing faster response times and reducing server load. .NET Core has an in-built caching feature, allowing developers to easily cache responses without third-party tools.
Implement Pagination
To improve API response times when retrieving significant amounts of data, pagination is recommended to limit query results. Pagination splits data into pages, limiting the amount of data delivered from each request and facilitating faster response times. It is essential to set an appropriate page size, so the pagination doesn’t negatively affect user experience.
Optimize Database Queries
Efficient database querying is crucial in reducing response times and server load when using APIs. Use techniques such as indexing frequently queried columns, avoiding table scans, and minimizing join operations. By using Entity Framework and .NET Core’s LINQ, developers can write efficient database queries with ease.
Versioning Your API
Versioning is crucial in API development to ensure backward compatibility, maintainability, and user satisfaction. By versioning your API, changes and updates are made to specific API versions, minimizing the risk of breaking changes. .NET Core provides various tools and libraries to achieve this, making API versioning a simple process.
Testing and Securing RESTful APIs
Once you have built your RESTful API using .NET Core and implemented efficient endpoints, the next step is to ensure that your API is secure and functioning optimally. Proper testing and security measures are crucial for API development best practices.
There are several tools and techniques you can use to test your RESTful API. One popular tool is Postman, which allows you to easily test your API by sending requests and examining responses. You can also use unit testing frameworks like xUnit or NUnit to ensure that specific parts of your API are functioning correctly.
In addition to testing, it is important to implement security measures to protect your API and the data it handles. You can use techniques like rate limiting and authentication to prevent unauthorized access and reduce the risk of attacks like SQL injection. .NET Core provides several security features, such as built-in support for OAuth 2.0 and OpenID Connect, that can help you implement these measures.
Another important security measure is to ensure that your API is always up-to-date with the latest security patches and updates. This means regularly monitoring for security vulnerabilities and implementing fixes as soon as they become available.
By following RESTful API best practices, leveraging the power of .NET Core, and implementing proper testing and security measures, you can ensure that your API is efficient, scalable, and secure.
Conclusion
Mastering RESTful API best practices with .NET Core is essential for building efficient endpoints that deliver optimal performance. By following the design principles of RESTful architecture, developers can create web APIs that are scalable, reliable, and easy to maintain.
Implementing efficient API endpoints with .NET Core requires careful planning and attention to detail. Developers should focus on optimizing database queries and minimizing network overhead to achieve maximum performance. Additionally, testing and securing RESTful APIs is critical for ensuring their reliability and preventing security breaches.
By following the best practices outlined in this article, developers can create RESTful APIs that are robust, scalable, and secure. Whether you are developing a new API or improving an existing one, .NET Core provides the tools and resources you need to build efficient endpoints that meet your organization’s unique needs.
FAQ
Q: What are RESTful API Best Practices?
A: RESTful API Best Practices are a set of guidelines and principles that help developers design and implement efficient and scalable REST APIs. These best practices ensure that the API is well-structured, easy to understand, and follows industry standards.
Q: How does .NET Core contribute to building efficient endpoints?
A: .NET Core provides a powerful framework for building efficient API endpoints. With its lightweight and modular architecture, .NET Core enables developers to create high-performance APIs that can handle a large number of requests. It also provides various features and tools that make it easier to optimize APIs for performance and scalability.
Q: What is the importance of RESTful API design principles?
A: RESTful API design principles are crucial for creating APIs that are easy to use, understand, and maintain. These principles ensure that APIs are consistent, standardized, and follow industry best practices. By adhering to these principles, developers can create APIs that are well-documented, self-descriptive, and provide a consistent user experience.
Q: How can .NET Core help in implementing efficient API endpoints?
A: .NET Core offers a wide range of features and tools that make it easier to implement efficient API endpoints. These include caching mechanisms, asynchronous programming models, and optimized algorithms. By leveraging these features and following best practices, developers can create APIs that are performant, scalable, and responsive.
Q: What are some best practices for testing and securing RESTful APIs?
A: Testing and securing RESTful APIs is essential to ensure the integrity and security of the data being transmitted. Some best practices for testing include writing unit tests, integration tests, and load tests to validate the functionality and performance of the API. For security, implementing authentication and authorization mechanisms, using HTTPS, and validating user input are recommended.