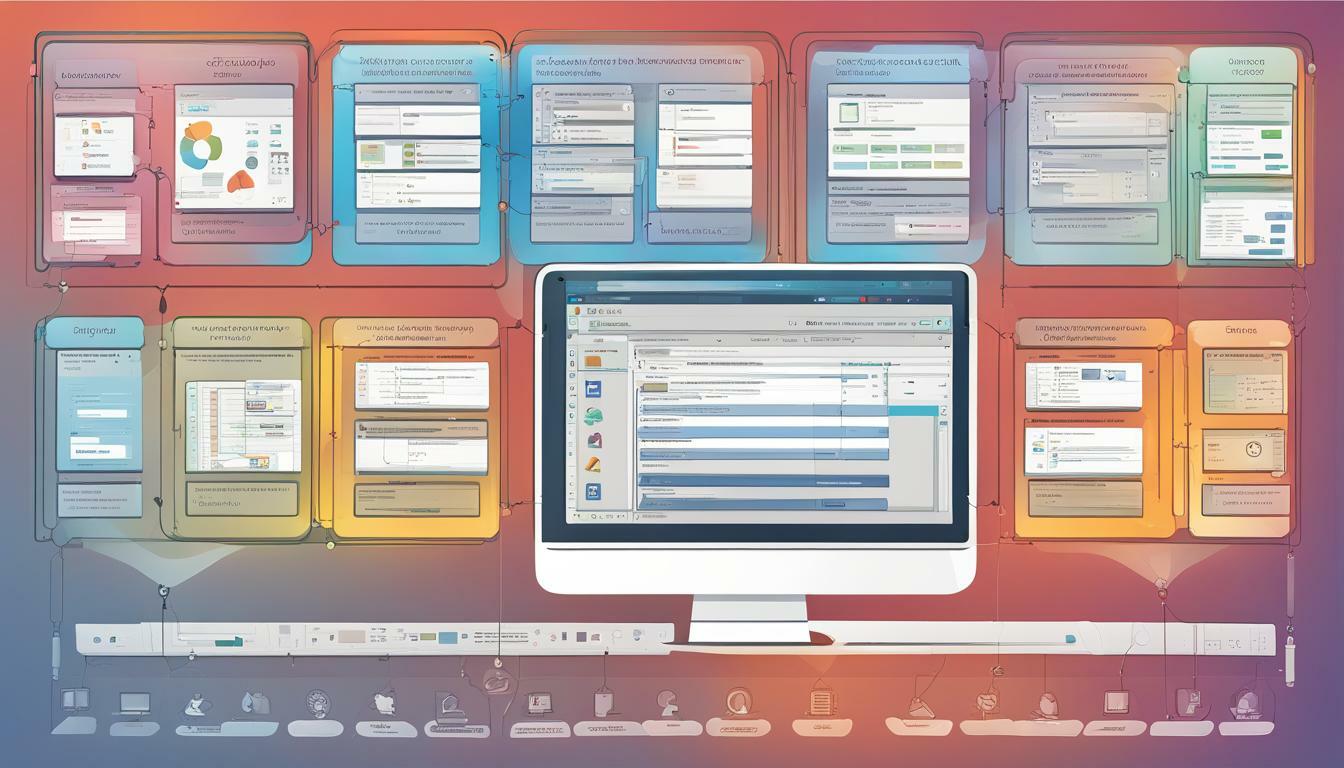
Software development is a complex process that requires careful consideration to ensure optimal results. Separation of concerns, which involves dividing responsibilities and streamlining software development, is a key concept that can greatly enhance the software design process. By following software design principles and adopting best practices, developers can create code that is easier to understand, maintain, and extend.
In this section, we will explore the concept of separation of concerns in-depth. We will discuss how it can lead to better software design and highlight the key principles involved. Additionally, we’ll highlight its importance in streamlining software development by dividing responsibilities effectively.
Key Takeaways:
- Separation of concerns involves dividing responsibilities to streamline software development.
- Adopting software design principles and best practices can lead to better software design.
- Streamlining software development can improve code organization, scalability, and collaboration among teams.
Understanding Software Architecture and Code Organization
Software architecture and code organization are crucial aspects of separation of concerns in software development. By utilizing these concepts, developers can create software that is both easy to maintain and scalable.
Software Architecture
Software architecture refers to the high-level design principles that govern the overall structure of the software system. It defines how different components of the software interact and communicate with each other to fulfill the requirements of the end-user. Good software architecture facilitates clear separation of concerns and allows developers to focus on specific areas of functionality without interfering with other parts of the system.
Code Organization
Code organization involves structuring the codebase into manageable units that can be easily maintained and updated over time. This is achieved through the use of components and modules that serve different functions within the software system. By dividing the code into smaller units, developers can implement separation of concerns effectively and prevent unwanted dependencies between different areas of the system.
Components | Modules |
---|---|
A component is a self-contained unit of functionality, typically implemented in a single file or folder. | A module is a set of related components that work together to achieve a particular goal. |
Components can be easily shared between different parts of the system, making them ideal for reuse and maintainability. | Modules provide a higher-level abstraction over components, allowing developers to reason about the system’s behavior in a more intuitive way. |
Effective code organization also involves enforcing clear boundaries between different areas of the system. This ensures that changes made in one area do not have unintended consequences elsewhere in the codebase.
Best Practices
When it comes to software architecture and code organization, there are several best practices that developers should follow:
- Adopt a modular approach to programming to promote separation of concerns
- Ensure that each component or module has a well-defined responsibility
- Use consistent naming conventions to make the codebase more readable
- Enforce clear boundaries between different areas of the system to prevent unwanted dependencies
- Implement code reviews to ensure that all code adheres to established design principles
By following these best practices, developers can optimize their software for maintainability and scalability, ultimately leading to a better user experience.
Exploring Design Patterns for Separation of Concerns
Design patterns are recurring solutions to common software design problems, and they can play a crucial role in achieving separation of concerns. By implementing these patterns, developers can divide responsibilities among software components and modules more effectively.
Model-View-Controller (MVC)
The Model-View-Controller (MVC) design pattern is widely used in software development to achieve separation of concerns. In this pattern, the application is divided into three distinct components: the Model, which represents the data and business logic; the View, which handles the presentation layer; and the Controller, which manages user interaction and acts as a mediator between the Model and the View.
The MVC pattern helps to achieve separation of concerns by ensuring that each component is responsible for a specific aspect of the application. This makes it easier to modify individual components without affecting the others, improving flexibility and maintainability.
Observer Pattern
The Observer pattern is another popular design pattern that can be used to implement separation of concerns. In this pattern, an object maintains a list of its dependents, also known as observers, and notifies them automatically of any changes to its state.
The Observer pattern promotes loose coupling between objects, allowing components to communicate without becoming dependent on each other. This is particularly useful in software development, as it allows developers to modify components independently without affecting the broader application.
Dependency Injection
The Dependency Injection pattern is a technique for achieving separation of concerns by allowing objects to receive dependencies from an external source, rather than creating them themselves. This approach reduces coupling between components and helps to ensure that each component is responsible for a specific task.
By using Dependency Injection, developers can improve the reusability of code and make it easier to test individual components in isolation. This pattern is widely used in modern software development frameworks and is considered a best practice for achieving separation of concerns.
The Benefits of Separation of Concerns in Software Development
Applying separation of concerns is a vital software development best practice. By dividing responsibilities effectively, developers can create code that is more modular, testable, and reusable. Below are some of the key benefits of implementing separation of concerns in software development:
- Improved modularity: Separating concerns leads to code that is easier to understand and modify. It’s easier to add and remove features without affecting the rest of the system.
- Enhanced testability: Separating concerns makes it easier to test individual components in isolation. This leads to more accurate and reliable testing and improved code quality.
- Increased reusability: Separating concerns leads to code that is more reusable across different projects or modules. This reduces development time and improves productivity.
Real-world examples showcase how separation of concerns has led to significant gains in productivity and flexibility. Some well-known examples include the Model-View-Controller (MVC) design pattern, which separates the concerns of user interface, data, and application logic, and Dependency Injection, which separates the concerns of object creation and dependency management.
In summary, implementing separation of concerns leads to better code organization, improved scalability, and enhanced collaboration among teams. Following software development best practices like these ensures that developers produce software that is easier to understand, maintain, and extend, and achieve long-term success in the fast-paced world of technology.
Implementing Separation of Concerns in Practice
Now that we understand the benefits of separation of concerns and the principles behind it, let’s discuss how to implement it in practice. Follow these steps to streamline software development:
Identify different concerns
Start by identifying different responsibilities in your software. For example, you could separate business logic from UI code or database access code from application logic. By doing this, you can isolate changes to specific areas, making testing easier and reducing the likelihood of bugs.
Use modular programming
Modular programming is an essential practice for implementing separation of concerns. Break your software down into small, reusable modules that perform specific tasks. This will simplify your codebase, make it easier to test, and enable you to reuse code across different projects.
Choose appropriate design patterns
Design patterns such as Model-View-Controller (MVC), Observer, and Dependency Injection can help achieve separation of concerns. MVC, for example, separates UI code from business logic and data storage, while Dependency Injection helps with loose coupling and testability. Choose patterns that fit your specific requirements.
Structure your codebase
Structure your codebase to achieve maximum modularity and maintainability. Group related modules together into separate directories or packages and use descriptive names to make it easy to find specific modules. Implement code reviews to ensure consistency and avoid disparate coding styles.
By following these best practices, you can ensure that your software is well-organized, maintainable, and scalable. Separating concerns will make it easier to debug and test code, and will ensure the longevity and flexibility of your software in the long run.
Overcoming Challenges and Common Pitfalls
While separation of concerns is a powerful tool for streamlining software development and improving code organization, it can present challenges and potential pitfalls for developers. Here are some strategies for overcoming these obstacles:
Dealing with Complex Dependencies
One of the main challenges when implementing separation of concerns is managing complex dependencies between different modules or components. It is important to identify these dependencies early on and find ways to reduce their complexity. One approach is to use a dependency injection framework that can manage dependencies automatically, making it easier to test and maintain code.
Managing Communication Between Components
Another challenge is managing communication between different components, especially when they are located in different parts of the system. One approach is to use a message-passing system that allows components to communicate asynchronously. This can help reduce coupling between components and improve overall system performance.
Mitigating Potential Performance Impacts
While separation of concerns can improve the modularization and testability of code, it can also have potential performance impacts. One approach to mitigating these impacts is to use lazy loading, which allows components to be loaded only when they are needed. Another approach is to use caching, which can improve system performance by reducing the need for repeated calculations or data access.
By understanding and addressing these challenges, developers can effectively implement separation of concerns in software development projects while avoiding common pitfalls. Embracing separation of concerns is a best practice that can lead to better software design and improved productivity.
Conclusion
Separation of concerns is a powerful concept that can streamline software development by dividing responsibilities effectively. By following software design principles, adopting modular programming, and utilizing appropriate design patterns, developers can optimize their software like professionals. Embracing this approach leads to better code organization, improved scalability, and enhanced collaboration among teams.
By implementing these best practices, developers can create software that is easier to understand, maintain, and extend, ensuring long-term success in the fast-paced world of technology. But it’s not always smooth sailing. Developers face challenges when implementing separation of concerns, such as complex dependencies, communication between components, and potential performance impacts. However, with strategies in place, developers can overcome these hurdles.
Dividing responsibilities using separation of concerns is a crucial best practice in software development. It improves modularity, testability, and reusability of code. By applying these principles, developers can create software that is scalable, reliable, and efficient. It’s time to embrace separation of concerns and streamline your software development process today.
FAQ
Q: What is Separation of Concerns?
A: Separation of Concerns is a software development principle that involves dividing responsibilities into distinct parts. This allows for better organization and management of code, making it more modular and maintainable.
Q: Why is Separation of Concerns important in software development?
A: Separation of Concerns is important in software development because it improves code clarity, scalability, and reusability. By dividing responsibilities, developers can more easily understand and modify specific parts of the codebase without impacting the entire system.
Q: How can I achieve Separation of Concerns in my code?
A: You can achieve Separation of Concerns in your code by using modular programming techniques, such as dividing your codebase into components and modules. Additionally, adopting appropriate design patterns, such as Model-View-Controller (MVC) or Dependency Injection, can also help separate concerns effectively.
Q: What are the benefits of Separation of Concerns in software development?
A: Separation of Concerns in software development brings several benefits. It improves code modularity, making it easier to test and maintain. It also enhances code reusability, as individual components can be reused in different contexts. Furthermore, it promotes collaboration among teams, as each team can focus on their specific domain of expertise.
Q: How can I implement Separation of Concerns in my software project?
A: To implement Separation of Concerns in your software project, start by identifying different concerns or responsibilities within your codebase. Once you have identified them, create separate modules or components for each concern. Finally, ensure proper communication and interaction between these components to fulfill the overall functionality of your project.
Q: What challenges and pitfalls can I expect when implementing Separation of Concerns?
A: When implementing Separation of Concerns, developers may face challenges such as managing complex dependencies between components, ensuring effective communication between them, and addressing potential performance impacts. It’s important to carefully plan and design your software architecture to overcome these challenges.