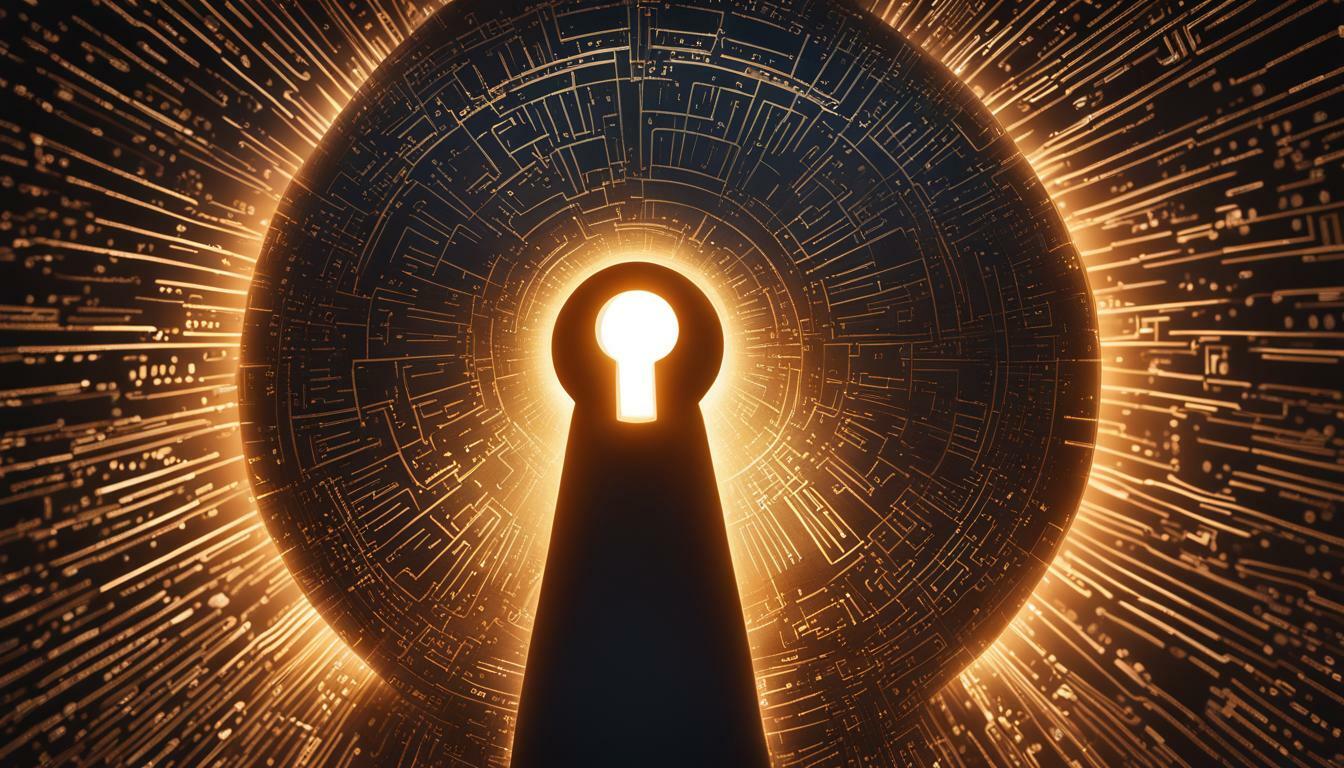
Welcome to our Java code snippets guide! Here, we will help you understand the importance of Java code snippets in programming. Whether you are a beginner or an experienced programmer, code snippets are a valuable tool for improving your skills. Our guide will cover programming examples in Java, Java coding samples, Java code examples, Java programming snippets, Java programming code, Java programming samples, Java code snippets for beginners, and Java code snippets for practice.
Our guide is divided into several sections, each designed to provide you with a comprehensive understanding of Java code snippets. We will cover the basics of Java code snippets, their syntax and structure, and how they can be used to solve complex problems. We will also provide easy-to-follow examples and exercises for beginners to practice with and advanced programmers to challenge themselves.
Understanding Java Code Snippets
Java code snippets are small pieces of code that can be reused in different parts of a program or in different programs altogether. They are designed to save time and effort by providing programmers with pre-written code that can be modified to fit their specific needs.
The syntax and structure of Java code snippets are similar to that of regular Java code. However, code snippets are typically smaller and more focused on a specific task or concept. This makes them easier to understand and implement, especially for beginners.
The benefits of using code snippets in Java programming are numerous. They can help programmers save time, improve efficiency, and reduce the risk of errors. Additionally, code snippets can be used to learn new programming techniques or to reinforce existing ones.
Examples of Java Code Snippets
Here are a few examples of Java code snippets:
Code Snippet | Description |
---|---|
System.out.println(“Hello, World!”); | Prints “Hello, World!” to the console. |
int x = 5; | Declares an integer variable named “x” and initializes it to the value 5. |
for (int i = 0; i System.out.println(i); } | Prints the numbers 0-9 to the console using a for loop. |
These examples demonstrate how Java code snippets can be used to perform simple tasks or to help streamline more complex processes.
Java Code Snippets for Beginners
If you are new to Java programming, using code snippets can be a great way to get started. These simple examples can help you understand the basics and build your foundational skills in Java programming.
One of the best things about Java code snippets for beginners is that they are easy to understand. They break down complex concepts into small, digestible pieces of code that anyone can follow.
Here are some examples of Java code snippets for beginners:
Code Snippet | Description |
---|---|
// Hello World Example public class HelloWorld { public static void main(String[] args) { System.out.println(“Hello, World!”); } } | This is a classic beginner example that simply prints “Hello, World!” to the console. |
// Add Two Numbers Example public class AddTwoNumbers { public static void main(String[] args) { int num1 = 5; int num2 = 7; int sum = num1 + num2; System.out.println(“The sum of ” + num1 + ” and ” + num2 + ” is ” + sum); } } | This example demonstrates how to add two numbers in Java and print their sum to the console. It also introduces the concept of variables. |
// For Loop Example public class ForLoopExample { public static void main(String[] args) { for (int i = 0; i System.out.println(“The value of i is ” + i); } } } | This example introduces the concept of loops in Java. It uses a for loop to print the value of the variable i five times. |
By practicing with these Java code snippets for beginners, you can begin to develop your skills and gain confidence in your programming abilities. Remember to take your time and experiment with the code to see how different modifications affect its behavior.
Advanced Java Code Snippets
For experienced programmers, using Java code snippets can help tackle more complex coding challenges. These snippets cater to those with a deeper understanding of Java programming. The use of advanced code snippets allows for more efficient, effective, and sophisticated coding practices.
Some advanced Java code snippets include:
Snippet | Description |
---|---|
try-catch-finally | This snippet is used for handling exceptions in Java programming. The try-catch-finally statement allows for a more structured approach to error handling. |
lambda expressions | This snippet can be used to improve code readability and reduce the amount of boilerplate code needed for certain processes. Lambda expressions allow for concise yet effective coding solutions. |
multithreading | This snippet allows for the execution of multiple threads simultaneously. This can improve the performance of Java applications by utilizing multiple cores of the CPU. |
Advanced Java code snippets require a solid understanding of the syntax and structure of Java programming. Mastery of these snippets can enhance a programmer’s ability to create efficient and sophisticated software applications.
Practicing with Java Code Snippets
Practice makes perfect! And that applies to programming too. To truly master Java code snippets, it is crucial to practice. Below are some coding exercises and challenges that will help you enhance your programming skills:
1. Basic Data Types
Create a Java program that declares variables of basic data types (int, char, double, boolean) and performs arithmetic and logical operations on them.
Variable Type | Example |
---|---|
int | int num1 = 5; |
char | char letter = ‘a’; |
double | double price = 9.99; |
boolean | boolean isTrue = true; |
2. Control Statements
Create a Java program that uses control statements (if-else, for, while) to perform operations based on specific conditions.
- Create a program that uses if-else statements to determine whether a number is even or odd.
- Create a program that uses a for loop to print out the Fibonacci sequence up to a certain number.
- Create a program that uses a while loop to find the factorial of a number.
3. Object-Oriented Programming
Create a Java program that utilizes object-oriented programming concepts like classes, objects, and inheritance.
- Create a class called “Person” with attributes like name and age. Create a method that outputs the person’s name and age.
- Create a class called “Student” that inherits from Person and has additional attributes like major and gpa. Create a method that outputs the student’s major and gpa.
- Create a class called “Teacher” that inherits from Person and has additional attributes like subject and years of experience. Create a method that outputs the teacher’s subject and years of experience.
Remember, these are just a few examples of the many exercises and challenges you can try out with Java code snippets. By practicing regularly, you’ll be able to apply the concepts and techniques you learn to real-world programming situations.
Conclusion
Java code snippets are a powerful tool for improving your programming skills. Throughout this guide, we’ve explored the concept of Java code snippets, from their syntax and structure to their practical applications in programming. We’ve provided easy-to-understand examples for beginners and more advanced snippets for experienced programmers.
By practicing with code snippets, you can reinforce your knowledge and build on your existing skills. We’ve provided a variety of exercises and challenges to help you practice and develop your proficiency in Java programming.
Keep Exploring with Java Code Snippets
Java code snippets are just the beginning of your journey as a programmer. There are countless ways to continue learning and experimenting with code snippets, from exploring new programming techniques to building your own projects from scratch.
We encourage you to keep exploring and experimenting with Java code snippets to further enhance your programming skills. With practice, you’ll gain the confidence and experience to tackle more challenging problems and develop even more complex applications.
Thank you for joining us on this journey into Java code snippets. We hope that this guide has provided you with a solid foundation to build on as you continue your programming journey. To learn more about Java programming and code snippets, be sure to check out the additional resources provided below.