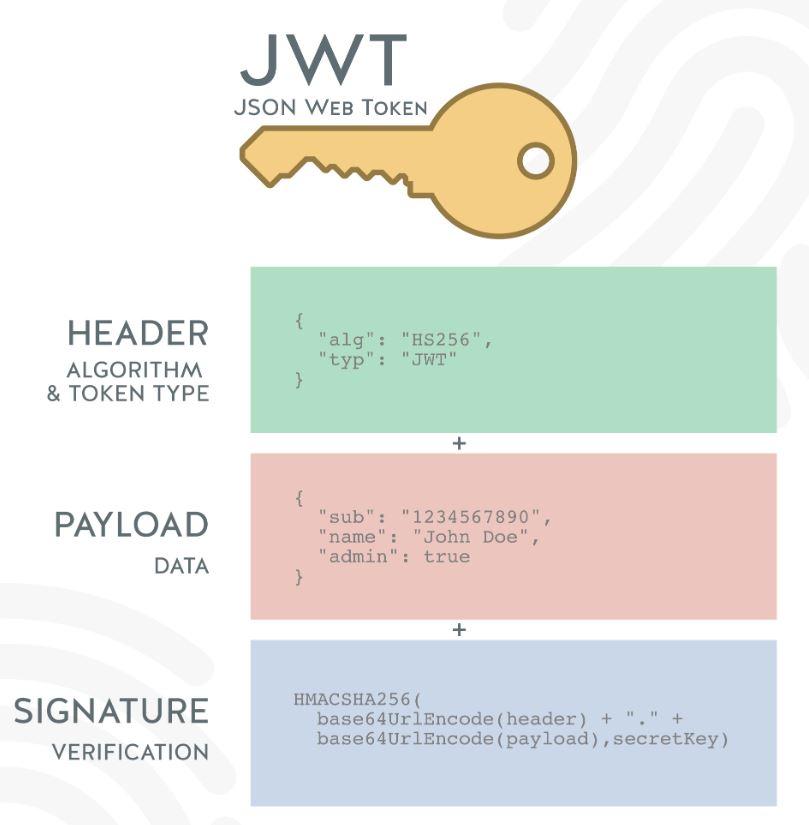
Introduction
In today’s digital landscape, secure authentication mechanisms are crucial for ensuring the integrity and confidentiality of data transmitted over the Internet. JSON Web Tokens (JWTs) have gained significant popularity as a means of securely transmitting information between parties. This article aims to provide a comprehensive understanding of JWTs, including their structure, benefits, and best practices for implementation.
What is a JSON Web Token (JWT)?
A JSON Web Token, commonly referred to as JWT, is an open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. JWTs are primarily used for authentication and authorization purposes and are widely adopted in modern web applications and APIs.
Structure of a JWT
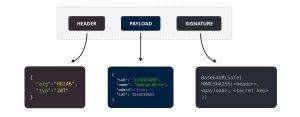
A JWT is composed of three parts: the header, the payload, and the signature. These parts are concatenated with periods (‘.’) to form a compact string representation. Let’s delve into each part’s details:
1. Header: The header contains two components: the type of token, which is JWT, and the signing algorithm used to secure the token, such as HMAC, RSA, or ECDSA. For example, a typical header can be represented as follows:
{
"alg": "HS256",
"typ": "JWT"
}
2. Payload: The payload contains the claims, which are statements about the entity (user, client, or server) and additional metadata. Claims are divided into three categories: registered, public, and private claims. Registered claims include standardized claims such as “iss” (issuer), “exp” (expiration time), “sub” (subject), and more. Public claims are defined by the JWT specification but are not mandatory, while private claims are custom claims specific to the application. An example of a payload can be:
{
"sub": "1234567890",
"name": "John Doe",
"iat": 1625663387
}
3. Signature: The signature is used to verify the integrity of the token and ensure that it has not been tampered with. It is generated by combining the encoded header, encoded payload, a secret key, and the specified signing algorithm. The secret key is known only to the server generating the token and is used to validate incoming tokens. For example:
HMACSHA256(
base64UrlEncode(header) + '.' +
base64UrlEncode(payload),
secret
)
Working Flow of JWTs
The typical flow of JWTs involves three parties: the client, the server providing the JWT, and the server validating the JWT. The steps are as follows:
1. Authentication: The client submits valid credentials (e.g., username and password) to the authentication server.
2. Token Generation: Upon successful authentication, the authentication server generates a JWT by signing the header and payload with a secret key.
3. Token Transmission: The JWT is returned to the client and is stored either in local storage, session storage, or a cookie, depending on the application’s requirements.
4. Requesting Protected Resources: The client includes the JWT in the Authorization header of subsequent requests to access protected resources. The JWT is typically sent in the “Bearer” authentication scheme, like this:
Authorization: Bearer <jwt-token>
5. Token Validation: The server receiving the request verifies the JWT’s integrity by recomputing the signature using the secret key. If the signature matches and the token is not expired, the request is considered authenticated.
Benefits of JWTs
JWTs offer several advantages, making them a popular choice for secure authentication:
1. Stateless and Scalable: Since JWTs contain all the necessary information, including user details and permissions, servers can verify the token’s integrity without needing database lookups or session management. This statelessness allows JWTs to be easily scaled across multiple servers, making them ideal for distributed systems.
2. Cross-Domain Usage: JWTs can be used across different domains or servers, as they are not tied to a specific session or cookie. This flexibility enables seamless integration between various services or microservices.
3. Security: JWTs are digitally signed, ensuring data integrity and preventing tampering. Additionally, they can be encrypted for further security, although encryption is optional in the JWT specification.
4. Extensibility: The payload of a JWT can be extended with custom claims, allowing developers to include application-specific data in the token. This extensibility makes JWTs versatile for different use cases.
Best Practices for JWT Implementation
To ensure the secure implementation of JWTs, consider the following best practices:
1. Protect the Secret Key: The secret key used to sign the JWT should be kept confidential and securely stored on the server. Leakage of the secret key compromises the security of the entire system.
2. Use Strong Signing Algorithms: Choose strong signing algorithms, such as HMAC with SHA-256 or RSA with at least a 2048-bit key size. Avoid using weak algorithms that can be easily compromised.
3. Validate and Verify: Always validate the received JWT, checking the signature’s integrity and expiration time. Additionally, validate the token’s claims to ensure they meet the expected criteria.
4. Avoid Storing Sensitive Data: Although JWTs are digitally signed, it is advisable not to include sensitive information (e.g., passwords) in the token’s payload to prevent any potential exposure.
5. Set Reasonable Expiration Times: Configure a sensible expiration time for the JWT to limit its validity. Shorter expiration times reduce the risk of token abuse if compromised.
Conclusion
JSON Web Tokens (JWTs) provide a secure and efficient mechanism for transmitting authentication and authorization data between parties. Their compact structure, statelessness, and extensibility make them an excellent choice for modern web applications and APIs. By following best practices and understanding the underlying concepts, developers can leverage JWTs to enhance the security and usability of their systems while promoting interoperability across various domains.