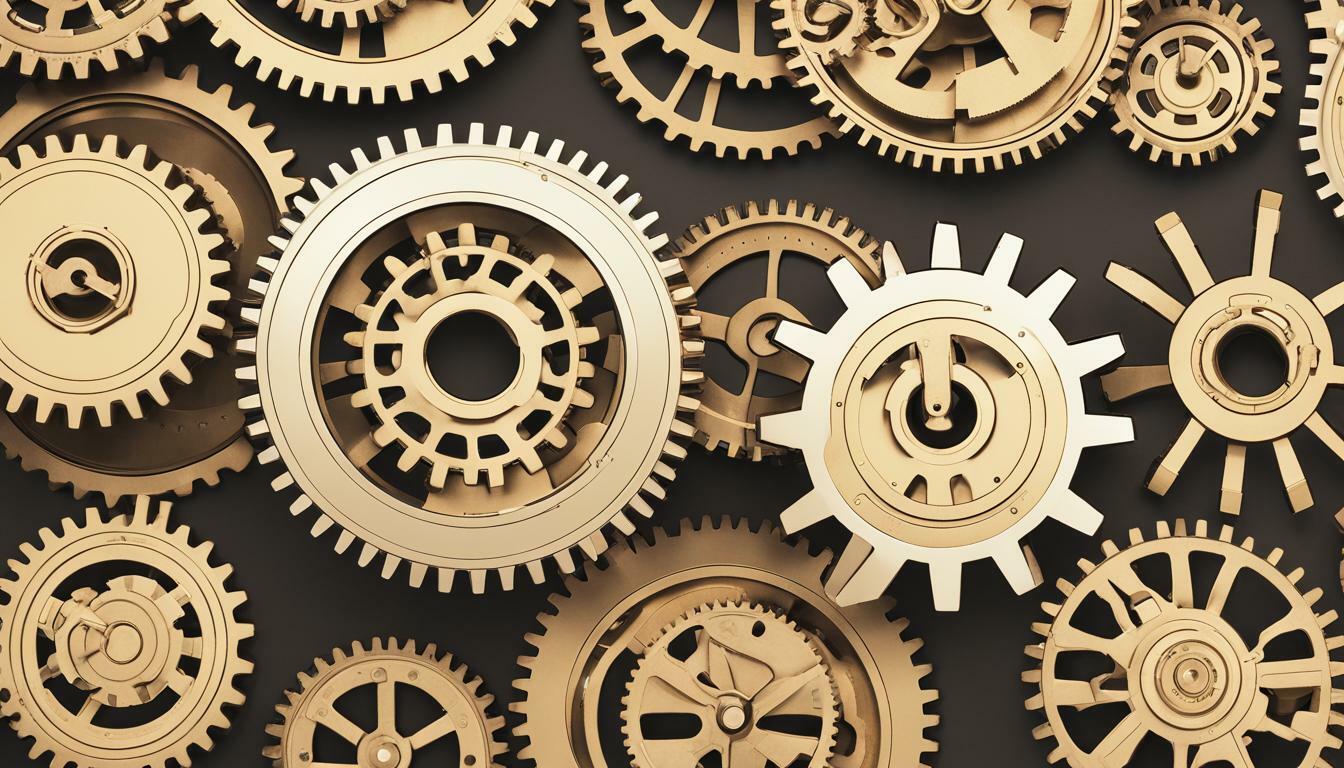
Software development is a complex process that involves creating robust and efficient systems. However, developers often encounter common challenges that can make this process more difficult. Software patterns offer recurring solutions to these challenges, providing developers with a proven way to address these issues.
Software patterns are a set of recurring solutions to commonly encountered problems in software development. These patterns can be used to optimize the coding process, improve software architecture, and ensure maintainability and scalability of the software. By using software patterns, developers can reduce the time and effort required to develop a software system, making it more efficient and effective.
Key Takeaways:
- Software patterns offer recurring solutions to commonly encountered problems in software development.
- Utilizing software patterns can optimize the coding process, improve software architecture, and ensure maintainability and scalability of the software.
- By using software patterns, developers can reduce the time and effort required to develop a software system, making it more efficient and effective.
Understanding Development Patterns: Best Practices and Strategies
In software development, adhering to best practices and implementing effective strategies can make all the difference. By utilizing development patterns, developers can create efficient and reusable code, leading to improved software engineering and a better end product.
Software architecture plays a crucial role in establishing effective development patterns. By creating a solid foundation, developers can focus on implementing patterns that will lead to optimal coding practices. This includes identifying areas where patterns can be implemented, understanding the underlying principles of effective software architecture, and creating a scalable and maintainable system.
Best Practices in Software Development Strategies
When it comes to software development strategies, there are several best practices to keep in mind. First and foremost, developers should prioritize code readability and maintainability. This means using descriptive variable and function names, breaking code into smaller, more manageable modules, and utilizing version control systems to keep track of changes.
Additionally, software development should be approached in a modular and iterative way. This means breaking down large projects into smaller components, testing each component individually, and making any necessary changes before moving on to the next component. This approach helps catch errors early on and ensures that each component is working as intended before being integrated into the larger system.
Strategies for Effective Software Architecture
Effective software architecture is essential for implementing development patterns. This includes creating a scalable and maintainable system that can handle future changes and updates. To achieve this, developers should prioritize modular design, identifying areas where components can be reused and breaking down larger systems into smaller, more manageable pieces.
Another key strategy for effective software architecture is to prioritize performance and efficiency. This means utilizing algorithms and data structures that are optimized for speed and memory usage, as well as minimizing unnecessary code and reducing redundancy.
By implementing these best practices and strategies, developers can create efficient and effective software systems through the use of development patterns and optimized software architecture.
Exploring Common Software Patterns: Solutions for Common Challenges
Software patterns offer recurring solutions to common development challenges, and utilizing these patterns can greatly enhance the coding process. In this section, we will explore some of the most common software patterns and how they can be applied to solve design challenges.
Singleton Pattern
The Singleton Pattern is a design pattern that ensures a class has only one instance and provides a global point of access to it. This pattern is commonly used in situations where you only need one instance of a class, such as a database connection or a logging service. By limiting the number of instances, the Singleton Pattern can help to reduce resource usage and increase efficiency.
Observer Pattern
The Observer Pattern is a design pattern that defines a one-to-many dependency relationship between objects so that when one object changes state, all of its dependents are notified and updated automatically. This pattern is useful in scenarios where one object’s state change should trigger updates in other objects. Examples include event-driven user interfaces and message passing systems.
Factory Pattern
The Factory Pattern is a design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. This pattern is useful when you need to create objects that require complex initialization or when you want to abstract the object creation process from the client code. The Factory Pattern can also be used to implement object pooling and object caching.
Decorator Pattern
The Decorator Pattern is a design pattern that allows you to add new functionality to an existing object dynamically. This pattern is useful when you want to add functionality to an object without subclassing it or when you want to add functionality at runtime. Examples include adding logging or caching functionality to an existing object.
By using these and other software patterns, developers can address common development challenges while creating efficient and robust software architecture. In the next section, we will discuss guidelines and considerations for effectively implementing software patterns in your development process.
Implementing Software Patterns: Guidelines and Considerations
Software patterns are a valuable tool in solving common development challenges, offering recurring solutions that can enhance the coding process. However, effectively implementing software patterns requires careful consideration and planning. Consider these guidelines to ensure successful integration of development patterns into your coding practices.
Choose the Right Pattern
When selecting a pattern, it is essential to understand the problem you are trying to solve and choose a pattern that addresses it effectively. Be careful not to force a pattern into a situation where it does not fit or is unnecessary. It is also important to keep in mind that there may be multiple patterns that can address a particular problem, so choose the one that is most appropriate for your specific situation.
Follow Best Practices
Ensure that you are following best practices when implementing software patterns. This includes writing clean and maintainable code, adhering to coding standards, and documenting your code effectively. Consistency is also crucial when introducing patterns into existing codebases, as inconsistencies can lead to confusion and mistakes.
Avoid Over-Engineering
When implementing software patterns, it is essential to find a balance between code simplicity and complexity. Over-engineering solutions can lead to unnecessary complexity, which can be difficult to maintain and evolve. Instead, try to keep your solutions simple and avoid adding unnecessary complexity that may lead to code bloat.
Maintainability and Scalability
When implementing patterns, it is important to consider maintainability and scalability. Ensure that your code is easy to maintain and that changes can be made efficiently. Plan for future growth and ensure that your code can scale effectively to meet changing business requirements.
In summary, implementing software patterns can be a valuable tool in solving common development challenges. However, it is essential to choose the right pattern, follow best practices, avoid over-engineering, and ensure maintainability and scalability. By carefully considering these guidelines, you can effectively integrate software patterns into your coding practices and achieve optimal results.
Conclusion
Software patterns are essential in software development, providing recurring solutions to common challenges. By utilizing these patterns, developers can optimize their coding practices and achieve efficient and robust software architecture.
Design patterns, in particular, offer proven solutions to commonly encountered problems. They can be applied in real-world scenarios to enhance the coding process. However, it’s important to follow best practices and guidelines when implementing software patterns to ensure maintainability and scalability.
Incorporating software patterns into your coding practices can seem daunting at first, but it’s a worthwhile investment. By understanding development patterns and the role of software architecture, you can create reusable code and efficient systems, leading to improved software engineering overall.
Start exploring software patterns in your own coding practices, and see the benefits they can bring to your software development process.
FAQ
Q: What are software patterns?
A: Software patterns are recurring solutions to common development challenges. They offer proven solutions that can enhance the coding process and address commonly encountered problems in software development.
Q: Why are software patterns valuable?
A: Software patterns are valuable because they provide reusable solutions that have been proven to work in various coding scenarios. They can improve the efficiency and reliability of software development by offering best practices and strategies.
Q: How can software patterns enhance the coding process?
A: Software patterns enhance the coding process by providing a set of guidelines and considerations that can be followed. They can help in creating maintainable and scalable code, as well as improving software architecture and engineering practices.
Q: What are development patterns?
A: Development patterns refer to recurring solutions and best practices in software development. They encompass various design patterns that can be applied to solve common challenges and improve coding practices.
Q: How can development patterns improve software engineering?
A: Development patterns can improve software engineering by offering reusable solutions and strategies that have been proven to work. By following these patterns, developers can enhance the quality and efficiency of their code.
Q: What are some common software patterns?
A: Some common software patterns include the Singleton pattern, Factory pattern, Observer pattern, and Adapter pattern. These patterns offer solutions to common challenges like object creation, event handling, and interface compatibility.
Q: How can software patterns be implemented effectively?
A: Software patterns can be implemented effectively by following guidelines and considerations. It is important to understand when to use a particular pattern, avoid potential pitfalls, and ensure the maintainability and scalability of the code.
Q: Why is software architecture important in implementing software patterns?
A: Software architecture plays a crucial role in implementing software patterns because it provides the structure and framework for the patterns to be integrated. A solid software architecture ensures that patterns can be applied effectively and that the resulting code is robust and efficient.