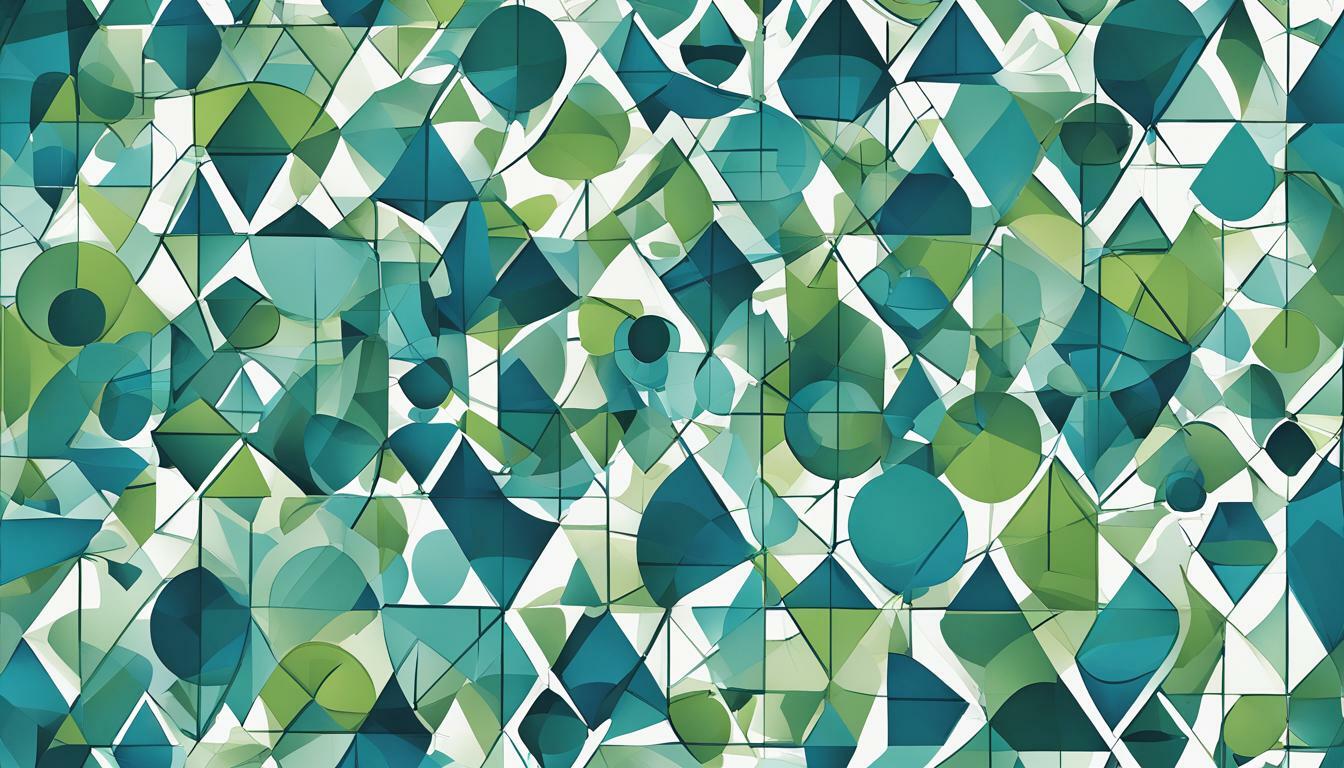
What is the Proxy Pattern in Object-Oriented Programming?
The Proxy Pattern is a design pattern used in object-oriented programming that provides a way to control access to real objects. In this pattern, a proxy object is created to act as a surrogate for the real object. The proxy object can perform additional tasks such as caching, logging, or even controlling access to the real object. The Proxy Pattern is a common technique used to add functionality to an existing object without changing its interface, making it a useful tool for developers.
The Proxy Pattern is particularly useful when real objects are expensive to create or when there is a need to limit access to sensitive objects. In these scenarios, a proxy object can be used to provide controlled access to the real object, reducing the chances of misuse or unauthorized access. By using the Proxy Pattern, developers can ensure that objects are being accessed in a safe and secure manner.
Overall, the Proxy Pattern is a powerful tool in object-oriented programming that can be used to solve a variety of access control challenges.
Enhancing Access Control with Proxy Objects
Proxy objects are a powerful tool in object-oriented programming for controlling access to real objects. They provide additional functionality and can be used to restrict access to sensitive or expensive resources. In this section, we explore the capabilities of proxy objects in enhancing access control.
Controlling Access to Sensitive Resources
Proxy objects can be used to gain control over access to sensitive resources such as personal information or confidential data. When a user requests access to these resources, the proxy object acts as a gatekeeper and determines whether the user has the necessary permissions to view the information. This helps to prevent unauthorized access and protects the privacy of sensitive data.
For example, in a healthcare application, a proxy object could be used to control access to patient information. Only authorized personnel with valid credentials could be granted access to view the patient’s medical records.
Restricting Access to Expensive Resources
Proxy objects can also be used to restrict access to expensive resources such as database connections or network resources. By controlling access to these resources, proxy objects can help to reduce server load and optimize performance.
For example, in a web application, a proxy object could be used to restrict access to a database connection. The proxy object could be configured to limit the number of concurrent database connections, preventing overloading of the database server.
Implementing Access Control with Proxy Objects
To implement access control using proxy objects, developers must define the necessary interfaces and methods for the proxy object to interact with the real object. The proxy object should also handle any necessary authentication and authorization checks before granting access to the real object.
For example, in a proxy object controlling access to a database connection, the proxy object would handle the database connection authentication and authorization checks before allowing access to the real database object.
Overall, the Proxy Pattern is a valuable tool for enhancing access control in object-oriented programming. By using proxy objects, developers can control access to sensitive or expensive resources, improving security and optimizing performance.
Steps to Implement the Proxy Pattern
The Proxy Pattern is a powerful tool for enhancing access control and adding functionality to real objects in software development. Follow these steps to successfully implement the Proxy Pattern in your application:
- Identify the real object: Determine which real object you want to control access to and enhance with additional functionality.
- Create the Proxy class: Design and implement the Proxy class that will control access to the real object.
- Implement the Proxy class: Define the functionality that the Proxy class will provide to the real object.
- Use the Proxy class: Integrate the Proxy class into your application and use it to control access to the real object.
When implementing the Proxy Pattern, it is essential to consider the unique requirements of your application. Take into account the type of real object, the desired level of access control, and the specific functionality you want to add with the Proxy class.
By following these steps, you can effectively incorporate the Proxy Pattern into your software development project and take advantage of its many benefits.
Practical Applications of the Proxy Pattern
The Proxy Pattern is a versatile design pattern that can be applied in various real-world scenarios. Here are some examples of how the Proxy Pattern is used:
1. Virtual Proxies for Optimizing Resource Usage
A virtual proxy is a type of proxy that creates a placeholder object for a resource-intensive object. When the real object is needed, the virtual proxy loads it, performs any necessary setup, and then delegates all further requests to the real object. This approach can significantly improve the performance of an application by reducing the number of times expensive resources are loaded and released.
For example, a virtual proxy can be used to load high-resolution images only when they are actually needed by the application, rather than loading them all at once. This can reduce the amount of memory and processing power required, ensuring that the application runs smoothly even on slower devices.
2. Protection Proxies for Access Control
A protection proxy is a type of proxy that provides access control to a sensitive object. The proxy checks the permissions of the user before allowing any access to the real object. This can be used to ensure that only authorized users can access sensitive data or perform critical operations.
For example, a protection proxy can be used to restrict access to a database of customer information. Only users with the appropriate permissions can access the database through the proxy, ensuring that the data is kept secure and confidential.
3. Remote Proxies for Remote Object Access
A remote proxy is a type of proxy that provides a local representation of a remote object. This can be used to access objects that are located on a remote server or network. The proxy acts as an intermediary between the client and the remote object, handling all communication and data transfer between the two.
For example, a remote proxy can be used to access a database that is located on a remote server. The client application sends requests to the proxy, which then communicates with the remote database to retrieve or update data.
4. Smart Proxies for Caching and Optimization
A smart proxy is a type of proxy that provides additional functionality beyond simple access control. The proxy can perform caching, logging, performance optimization, or other tasks to improve the overall performance of an application.
For example, a smart proxy can be used to cache frequently accessed data to reduce the load on the server. The proxy can store the data locally and serve it to clients as needed, reducing the amount of network traffic and improving the response time of the application.
Conclusion
The Proxy Pattern is a powerful design pattern that can be used to solve a wide range of access control and optimization challenges. By using proxies, developers can control access to sensitive objects, optimize resource usage, and improve the overall performance of their applications.
Pros and Cons of the Proxy Pattern
The Proxy Pattern is a powerful design pattern that provides numerous benefits for software developers. Here are some of the advantages:
- Enhanced security: Proxy objects can control access to sensitive resources, providing an additional layer of security. This is especially important in scenarios where unauthorized access could lead to serious consequences.
- Increased performance: Proxy objects can cache expensive resources, reducing the load on the system and improving overall performance.
- Improved scalability: Proxy objects allow for the dynamic creation and destruction of objects, enabling the system to scale more effectively.
- Flexible communication: Proxy objects can be used to establish communication between different components of a system, improving the overall flexibility and maintainability of the system.
However, there are also some potential drawbacks to using the Proxy Pattern:
- Increased complexity: Implementing the Proxy Pattern can add additional complexity to the codebase, requiring more time and effort from the development team.
- Potential performance issues: If not implemented correctly, the Proxy Pattern can actually have a negative impact on performance, particularly if there are frequent requests for expensive resources.
- Difficulty in debugging: Due to the indirection introduced by the Proxy Pattern, debugging can become more challenging, particularly in complex systems.
Overall, while the Proxy Pattern offers significant advantages, developers should carefully consider the potential limitations and weigh the benefits against the costs before deciding to implement the pattern in their applications.
Embracing the Smart Way: Proxy Pattern for Object Access Control
In conclusion, the Proxy Pattern has proven to be an effective solution for controlling object access in software development. By using proxy objects, developers can manage access to sensitive or expensive resources, while also providing additional functionality to the application.
Implementing the Proxy Pattern requires careful consideration of the necessary components and proper steps. However, the benefits of using this design pattern outweigh the challenges. It enhances access control and provides a smarter way to manage objects.
Real-world examples have showcased the versatility of the Proxy Pattern, demonstrating its effectiveness in solving common access control challenges. By embracing this smart way of controlling object access, developers can enhance the security and functionality of their applications.
Final Thoughts
The Proxy Pattern is a valuable tool for software developers. By implementing this pattern, developers can manage access to sensitive or expensive resources, while also providing additional functionality to the application. It is a smart way to control object access and improve the security and functionality of the application.
When using the Proxy Pattern, developers should carefully consider the necessary components and weigh the advantages and limitations. With the proper implementation and understanding of the pattern, developers can enhance their software development skills and create more functional and secure applications.
FAQ
Q: What is the Proxy Pattern in Object-Oriented Programming?
A: The Proxy Pattern is a design pattern in object-oriented programming that involves the use of proxy objects. These proxy objects act as intermediaries between clients and real objects, controlling access to the real objects and providing additional functionality.
Q: How do proxy objects enhance access control?
A: Proxy objects enhance access control by providing a layer of abstraction between clients and sensitive or expensive resources. They can enforce access restrictions, perform validation or authentication checks, and even cache results to improve performance.
Q: What are the steps to implement the Proxy Pattern?
A: To implement the Proxy Pattern, you need to define an interface for both the subject (real object) and the proxy class. Then, create concrete implementations of both classes and modify the client code to use the proxy instead of directly interacting with the subject. Finally, add any additional functionality or access control logic to the proxy class as needed.
Q: Can you provide some practical examples of the Proxy Pattern?
A: Certainly! The Proxy Pattern is commonly used in scenarios such as lazy loading of objects, remote method invocation, caching, access control, and logging. For example, a proxy object can delay the creation of a resource until it is actually needed (lazy loading), or it can handle remote method invocations by forwarding the requests to a remote server.
Q: What are the pros and cons of using the Proxy Pattern?
A: The Proxy Pattern offers advantages such as improved access control, increased modularity, and enhanced performance through caching. However, it also introduces additional complexity, can impact the responsiveness of the system, and may require careful consideration of thread safety in multi-threaded environments.
Q: Why should developers embrace the Proxy Pattern for object access control?
A: The Proxy Pattern provides a smart and flexible approach to object access control. By using proxy objects, developers can easily enforce access restrictions, implement caching mechanisms, and add custom functionality without modifying the core behavior of the real objects. It empowers developers to design robust and secure applications while maintaining code modularity and reusability.